Go - (6) Arrays and Slices
Arrays In Go, arrays have a fixed size. var marks [10]int // an array of 10 integers Assigning values to an array looks like this. names := [3]string{ "Edward", "Bella", "Jcob", } In Go, if you assign an array to another array, it copies all the elements of the first array. Also, when you pass an array to a function, it gets a copy of the array. But what if you want to allocate size dynamically? Slice Built on top of arrays. Holds a reference to the underlying array. Dynamically-sized In Go, we work with slices 90% of the time. Unlike assigning an array to another array, when you assign a slice to another slice, both points to the same array. This gives pointer-like behaviour. sliceA := make([]int, 4, 8) sliceFixed := make([]string, 5) sliceLiteral := []string{"hello", "hi", "thanks"} Capacity of a slice is the maximum length it can take. If the data exceeded the capacity, the slice is reallocated. You can get the capacity of a slice using the cap() function. Length is the current length (number of elements) a slice has. You can get the length of a slice using the len() function. In the above example, sliceA is an int slice, which is 4 in length but has a capacity of 8. Both the length and capacity of the sliceFixed size are 5. Also, if you print the length of sliceLiteral, it will give 3. Variadic functions A function that can take an arbitrary number of final arguments. Check the example below. The printNames() function takes the names argument, which is a slice. You can see it in the main function where we call the printNames(). Note that we can pass a slice any number of string elements. func printNames(names ...string) { // names is a slice for i := 0; i
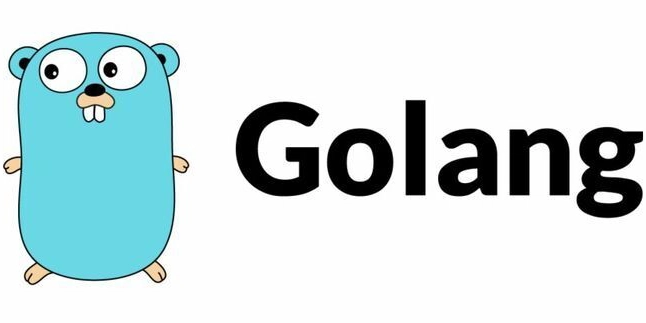
Arrays
- In Go, arrays have a fixed size.
var marks [10]int // an array of 10 integers
Assigning values to an array looks like this.
names := [3]string{
"Edward",
"Bella",
"Jcob",
}
In Go, if you assign an array to another array, it copies all the elements of the first array. Also, when you pass an array to a function, it gets a copy of the array.
But what if you want to allocate size dynamically?
Slice
- Built on top of arrays.
- Holds a reference to the underlying array.
- Dynamically-sized
- In Go, we work with slices 90% of the time.
Unlike assigning an array to another array, when you assign a slice to another slice, both points to the same array. This gives pointer-like behaviour.
sliceA := make([]int, 4, 8)
sliceFixed := make([]string, 5)
sliceLiteral := []string{"hello", "hi", "thanks"}
Capacity of a slice is the maximum length it can take. If the data exceeded the capacity, the slice is reallocated. You can get the capacity of a slice using the
cap()
function.Length is the current length (number of elements) a slice has. You can get the length of a slice using the
len()
function.
In the above example, sliceA
is an int slice, which is 4 in length but has a capacity of 8. Both the length and capacity of the sliceFixed
size are 5. Also, if you print the length of sliceLiteral
, it will give 3.
Variadic functions
- A function that can take an arbitrary number of final arguments.
Check the example below. The printNames()
function takes the names
argument, which is a slice. You can see it in the main function where we call the printNames()
. Note that we can pass a slice any number of string elements.
func printNames(names ...string) {
// names is a slice
for i := 0; i < len(names); i++ {
fmt.Println(names[i])
}
}
func main() {
users := []string{"edward", "jcob", "bella"}
printNames(users...)
}
Instead of a for
loop, you can use range
to print elements of a slice.
func printSweets() {
sweets := []string{"cake", "chocolate", "toffee", "macrons"}
for _, s := range sweets {
fmt.Println(s)
}
}