TS2329: Index signature for type '{0}' is missing in type '{1}'
TS2329: Index signature for type '{0}' is missing in type '{1}' TypeScript is a strongly typed superset of JavaScript. This means that it builds on top of JavaScript by adding an optional type system (a way to define and enforce the kinds of data being used in your code). TypeScript is a powerful language designed to catch errors early during development, making your JavaScript codebases more robust and maintainable. In TypeScript, "types" play a central role. A type defines the shape of data, specifying what kind of data will be stored, its expected structure, and the operations you can perform on it. For example, string, number, boolean, and object are common types used in TypeScript. Types ensure that your code only operates on the data it was designed to handle; if it doesn’t match the expected type, TypeScript will alert you during the development process. If you're eager to learn more about TypeScript or improve your programming skills using AI tools, consider following our blog or trying tools like GPTeach that can help you master coding concepts. What are Interfaces? An interface in TypeScript is a way to define the shape of an object. It serves as a contract or blueprint that an object must adhere to. Interfaces are extremely useful for defining the expected structure of objects and ensuring that the object matches this structure. For example: interface Person { name: string; age: number; } const john: Person = { name: "John Doe", age: 30, }; // This works because john adheres to the Person interface. Interfaces save developers from making mistakes such as accidentally leaving out properties or assigning values of the wrong type. They're fundamental to writing clean, reusable, and maintainable TypeScript code, which leads us to the error TS2329: Index signature for type '{0}' is missing in type '{1}'. Understanding TS2329: Index signature for type '{0}' is missing in type '{1}' What Does the Error Mean? The error TS2329: Index signature for type '{0}' is missing in type '{1}' occurs when you attempt to assign an object to a type (e.g., an interface or other type) that requires an index signature, but the object you're passing doesn’t satisfy this requirement. An index signature allows TypeScript to specify the allowed property names for an object. For example: interface StringDictionary { [key: string]: string; // Index signature: any key of type 'string', value must be 'string' } const example: StringDictionary = { username: "john_doe", age: 30, // ❌ Error: number is not assignable to string }; Here are two key reasons why you might encounter this error: Your object is missing an index signature when the type or interface requires one. The types of the values in your object do not match the expected type defined in the index signature. Important to Know! What is an Index Signature?: An index signature is a way to tell TypeScript that an object can have any number of properties of a certain type, as long as the keys and/or values align with the type definition. For example: interface NumericIndex { [key: string]: number; // Any key of type 'string' must have a 'number' value. } Why Use Index Signatures?: Index signatures are especially handy when you don't know the property names ahead of time but still want to enforce a structure on potential values. Example of TS2329: Index signature for type '{0}' is missing in type '{1}' Let’s dive into an example where this error occurs: interface UserRoles { [role: string]: boolean; // All property keys must be strings, and values must be boolean } const roles: UserRoles = { admin: true, editor: false, viewer: "yes", // ❌ Error! Index signature requires value to be a boolean }; In this case, the error TS2329: Index signature for type '{string}' is missing in type '{UserRoles}' occurs because the value "yes" is a string, but the index signature specifies boolean. To fix this, every property value must match the boolean type: const roles: UserRoles = { admin: true, editor: false, viewer: false, // ✅ Fixed: now matches index signature. }; How to Fix the Error? Here’s a step-by-step checklist to resolve TS2329: Index signature for type '{0}' is missing in type '{1}' in your TypeScript code: Understand the Index Signature Requirements: Double-check what your interface or type expects in terms of property keys and values. If you're working with an index signature, ensure your object adheres to it. Update the Object's Values: Ensure that the values of the object's properties match the type specified in the index signature. For example: interface Config { [key: string]: number; // All values must be numbers. } const appConfig: Config = { maxUsers: 100, debugMode: "true", // ❌ Error, string is not a number }; const fixedConfig: C
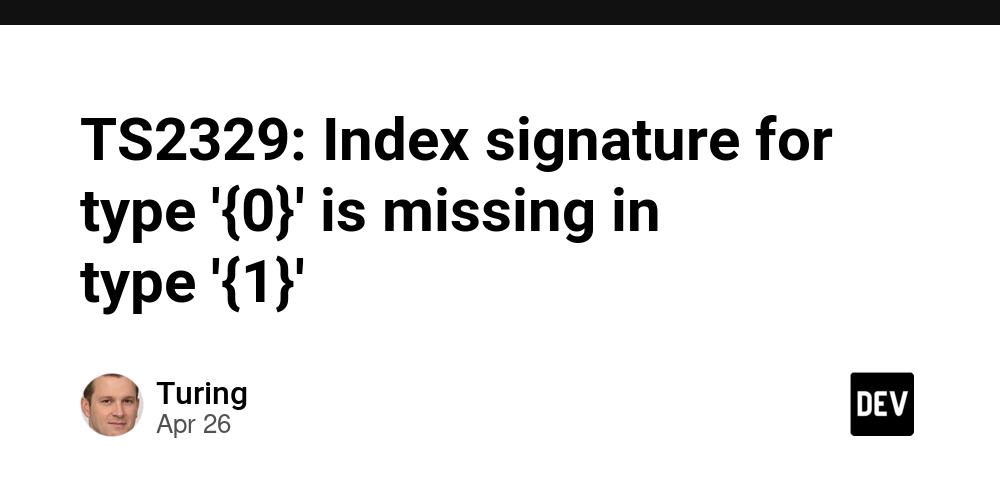
TS2329: Index signature for type '{0}' is missing in type '{1}'
TypeScript is a strongly typed superset of JavaScript. This means that it builds on top of JavaScript by adding an optional type system (a way to define and enforce the kinds of data being used in your code). TypeScript is a powerful language designed to catch errors early during development, making your JavaScript codebases more robust and maintainable.
In TypeScript, "types" play a central role. A type defines the shape of data, specifying what kind of data will be stored, its expected structure, and the operations you can perform on it. For example, string
, number
, boolean
, and object
are common types used in TypeScript. Types ensure that your code only operates on the data it was designed to handle; if it doesn’t match the expected type, TypeScript will alert you during the development process.
If you're eager to learn more about TypeScript or improve your programming skills using AI tools, consider following our blog or trying tools like GPTeach that can help you master coding concepts.
What are Interfaces?
An interface in TypeScript is a way to define the shape of an object. It serves as a contract or blueprint that an object must adhere to. Interfaces are extremely useful for defining the expected structure of objects and ensuring that the object matches this structure. For example:
interface Person {
name: string;
age: number;
}
const john: Person = {
name: "John Doe",
age: 30,
};
// This works because john adheres to the Person interface.
Interfaces save developers from making mistakes such as accidentally leaving out properties or assigning values of the wrong type. They're fundamental to writing clean, reusable, and maintainable TypeScript code, which leads us to the error TS2329: Index signature for type '{0}' is missing in type '{1}'.
Understanding TS2329: Index signature for type '{0}' is missing in type '{1}'
What Does the Error Mean?
The error TS2329: Index signature for type '{0}' is missing in type '{1}' occurs when you attempt to assign an object to a type (e.g., an interface or other type) that requires an index signature, but the object you're passing doesn’t satisfy this requirement. An index signature allows TypeScript to specify the allowed property names for an object.
For example:
interface StringDictionary {
[key: string]: string; // Index signature: any key of type 'string', value must be 'string'
}
const example: StringDictionary = {
username: "john_doe",
age: 30, // ❌ Error: number is not assignable to string
};
Here are two key reasons why you might encounter this error:
- Your object is missing an index signature when the type or interface requires one.
- The types of the values in your object do not match the expected type defined in the index signature.
Important to Know!
- What is an Index Signature?: An index signature is a way to tell TypeScript that an object can have any number of properties of a certain type, as long as the keys and/or values align with the type definition. For example:
interface NumericIndex {
[key: string]: number; // Any key of type 'string' must have a 'number' value.
}
- Why Use Index Signatures?: Index signatures are especially handy when you don't know the property names ahead of time but still want to enforce a structure on potential values.
Example of TS2329: Index signature for type '{0}' is missing in type '{1}'
Let’s dive into an example where this error occurs:
interface UserRoles {
[role: string]: boolean; // All property keys must be strings, and values must be boolean
}
const roles: UserRoles = {
admin: true,
editor: false,
viewer: "yes", // ❌ Error! Index signature requires value to be a boolean
};
In this case, the error TS2329: Index signature for type '{string}' is missing in type '{UserRoles}' occurs because the value "yes"
is a string, but the index signature specifies boolean
. To fix this, every property value must match the boolean
type:
const roles: UserRoles = {
admin: true,
editor: false,
viewer: false, // ✅ Fixed: now matches index signature.
};
How to Fix the Error?
Here’s a step-by-step checklist to resolve TS2329: Index signature for type '{0}' is missing in type '{1}' in your TypeScript code:
-
Understand the Index Signature Requirements:
- Double-check what your interface or type expects in terms of property keys and values.
- If you're working with an index signature, ensure your object adheres to it.
-
Update the Object's Values:
- Ensure that the values of the object's properties match the type specified in the index signature. For example:
interface Config {
[key: string]: number; // All values must be numbers.
}
const appConfig: Config = {
maxUsers: 100,
debugMode: "true", // ❌ Error, string is not a number
};
const fixedConfig: Config = {
maxUsers: 100,
debugMode: 1, // ✅ Fixed
};
-
Check for Index Signature in the Type or Interface:
- Add an appropriate index signature to your type or interface if one doesn’t exist but you expect the object to have dynamic properties.
interface ProductInventory {
[productId: string]: number;
}
const warehouse: ProductInventory = {
"item-123": 50,
"item-456": 10,
}; // ✅ Works because the interface supports these dynamic properties.
-
Avoid Mismatched Types:
- Index signatures are strict about types. If a type mismatch exists, refactor your code to align with the expected key-value pair types.
Important to Know!
- Dynamic Objects: If you’re working with objects whose properties can vary (e.g., data from an API), use index signatures to enforce type safety while maintaining flexibility.
- Explicit Types: Always ensure that both keys and values explicitly match the required types when applying an index signature.
FAQ Section
Q: Why do I need an index signature in TypeScript?
A: Index signatures are crucial when working with objects that have dynamic property names. For instance, if you’re managing a dictionary-like structure where the property names aren’t fixed, index signatures help enforce the expected types for property keys and values.
Q: Can I use both fixed properties and an index signature in an interface?
A: Yes! You can define fixed properties alongside an index signature, as long as the fixed properties adhere to the rules of the index signature. For example:
interface PersonDetails {
name: string; // Fixed property
[key: string]: string; // Index signature
}
Q: What causes TS2329 specifically?
A: This error happens when you try to assign an object to a type with an index signature, but the object doesn’t meet the requirements (e.g., mismatched types or missing signatures).
In conclusion, TS2329: Index signature for type '{0}' is missing in type '{1}' is a common TypeScript error when working with custom types or interfaces requiring index signatures. By understanding how index signatures work and ensuring your objects align with these definitions, you’ll avoid this error and write better, safer TypeScript code. TypeScript is a tool to help developers, and errors like these push us to create more structured and thoughtful applications. Happy coding!