Building My First Telegram Bot with Node.js — A Beginner’s Journey. My first step to escape tutorial HELL
Good Morning guys, so When i was preparing for JEE, I had a friend who knew python and made a telegram bot. I wondered how? I was amazed, I always thought it would be amazing if i was able to make it someday. And in college years i choose JavaScript as my programming language. In my second year i thought why not try making a telegram bot but with javascript. and here i'm going to teach you how i actually did it. Well before knowing that you should first have a clear difference between how normal servers, web-sockets and polling work Servers:- Its like when you post something(lets say from client) it receives the request do stuff and just reply with response back to client. Here the thing you have to notice is that request is always from client. Backend(server) never request for anything and just replies. WebSockets:- This is like a continuous conversation between the client and server. Once the connection is made, the server can send updates to the client whenever something new happens, without the client needing to constantly ask. In short, both client and server can request for and response and not just client. Polling:- when the client (in this case, your Telegram bot) asks the server repeatedly for updates at a certain interval. It’s like sending a "Hey, any new messages?" every 5 seconds. This can be inefficient, as you're constantly making requests even if there's nothing new. In server Client requests, server responds. In WebSockets Continuous, real-time bidirectional communication. In Polling Client repeatedly asks the server for updates. Okay great now that you know difference between these three its time to start building. P.S.: I built this using Polling. Why? Because HTTP-based requests aren't real-time. You'd have to keep asking the server repeatedly to check for updates (which is basically what polling does). And right now, Telegram doesn't support WebSockets for bots, so polling was the most suitable method for achieving real-time communication. So, Whats the first step? I hope you already installed NodeJs and all now *STEP 1:- Create a Telegram Bot with BotFather * Open your telegram. In the search bar, type "BotFather" and click on the verified user (it will be the official bot provided by Telegram). Click the "Start" button at the bottom of the screen. And select /newbot to create a new bot BotFather will ask you to give your bot a name. This can be anything, like "ChatBot" or "Tele-bot" anything. Then, you'll have to choose a username for your bot, but it has to end with "bot" example:- TetrisBot or tetris_bot (The username for your Telegram bot has to be unique.) Yayy, you have officially created your bot. But wait when i start the boat why isn't it responding? We will get there soon, First copy the HTTP API token and save it to .env file (Don’t share this token, it’s like the password to your bot) *STEP 2:- INTEGRATING "HELLO WORLD" FROM CHATBOT * Now that you have NodeJs installed give simple npm init and make your server.js Now You'll need to install the necessary Telegram Bot API package for Node.js. In this case, we're using node-telegram-bot-api. This will allow you to interact with the Telegram API. By using this api, you can create a Telegram bot without dealing with the low-level complexities of the Telegram API. Whether it’s polling, sending messages, or handling updates, this package simplifies the process and helps you build bots faster. (node-telegram-bot-api Docs) If you refer their docs you will understand the first few lines of code ` // Import the library that lets your Node.js app talk to the Telegram Bot API. const TelegramBot = require('node-telegram-bot-api'); // replace the value below with the Telegram token you receive from @botfather (use require dotenv) const token = 'YOUR_TELEGRAM_BOT_TOKEN'; // This creates a new instance of your Telegram bot using the token. Polling true allow bot to use polling method. const bot = new TelegramBot(token, {polling: true}); ` Start bot by adding bot.on('message', callback) it is like saying: “Hey, whenever someone sends a message to the bot, do something.” that something is the callback Inside the call back we give some parameter lets say msg. That msg is going to all information regarding the message. i.e who sent it(id), the text, and more. msg.chat.id gets the unique ID of the chat. while bot.sendMessage is used to send the response to client, when we give it a chatID it will send that text to particular chat (whose chatid is provided) bot.on('message', (msg) => { const chatId = msg.chat.id; bot.sendMessage(chatId, 'Hello, World!'); }); Now Your bot is sending Hello, World! NOW HERE'S A WAY TO ESCAPE THE TUTORIAL HELL. YOU CAN REFER THIS GITHUB FOR HELP IF YOU NEED 1) You can create a route that accepts a parameter. You can use msg.text inside bot.on to access the text coming from the client and send that as an argument to the route. Then, you can use that text to send it to Gemini, process the
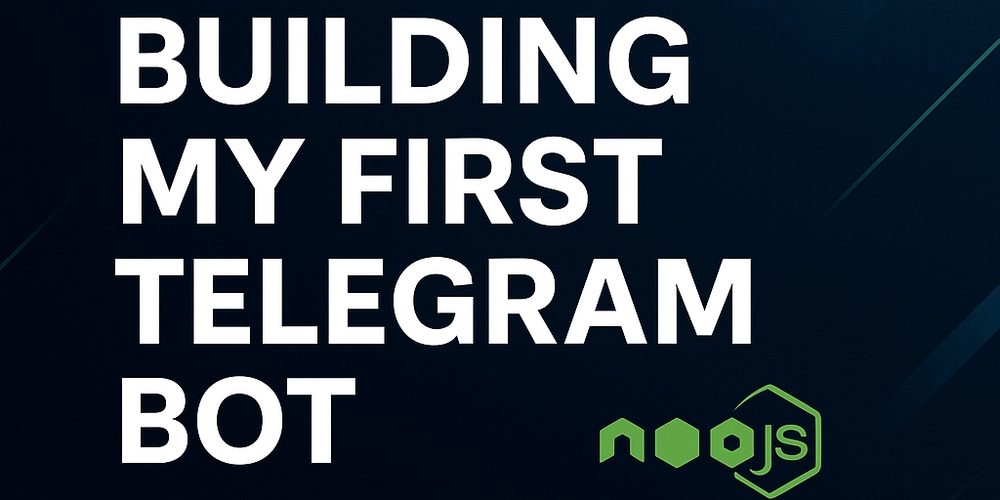
Good Morning guys, so When i was preparing for JEE, I had a friend who knew python and made a telegram bot. I wondered how? I was amazed, I always thought it would be amazing if i was able to make it someday. And in college years i choose JavaScript as my programming language. In my second year i thought why not try making a telegram bot but with javascript. and here i'm going to teach you how i actually did it.
Well before knowing that you should first have a clear difference between how normal servers, web-sockets and polling work
Servers:- Its like when you post something(lets say from client) it receives the request do stuff and just reply with response back to client. Here the thing you have to notice is that request is always from client. Backend(server) never request for anything and just replies.
WebSockets:- This is like a continuous conversation between the client and server. Once the connection is made, the server can send updates to the client whenever something new happens, without the client needing to constantly ask. In short, both client and server can request for and response and not just client.
Polling:- when the client (in this case, your Telegram bot) asks the server repeatedly for updates at a certain interval. It’s like sending a "Hey, any new messages?" every 5 seconds. This can be inefficient, as you're constantly making requests even if there's nothing new. In server Client requests, server responds. In WebSockets Continuous, real-time bidirectional communication. In Polling Client repeatedly asks the server for updates.
Okay great now that you know difference between these three its time to start building. P.S.: I built this using Polling. Why? Because HTTP-based requests aren't real-time. You'd have to keep asking the server repeatedly to check for updates (which is basically what polling does). And right now, Telegram doesn't support WebSockets for bots, so polling was the most suitable method for achieving real-time communication.
So, Whats the first step? I hope you already installed NodeJs and all
now
*STEP 1:- Create a Telegram Bot with BotFather
*
Open your telegram. In the search bar, type "BotFather" and click on the verified user (it will be the official bot provided by Telegram).
Click the "Start" button at the bottom of the screen. And select /newbot to create a new bot
BotFather will ask you to give your bot a name. This can be anything, like "ChatBot" or "Tele-bot" anything.
Then, you'll have to choose a username for your bot, but it has to end with "bot" example:- TetrisBot or tetris_bot (The username for your Telegram bot has to be unique.)
Yayy, you have officially created your bot. But wait when i start the boat why isn't it responding? We will get there soon, First copy the HTTP API token and save it to .env file (Don’t share this token, it’s like the password to your bot)
*STEP 2:- INTEGRATING "HELLO WORLD" FROM CHATBOT
*
Now that you have NodeJs installed give simple npm init and make your server.js
Now You'll need to install the necessary Telegram Bot API package for Node.js. In this case, we're using node-telegram-bot-api. This will allow you to interact with the Telegram API. By using this api, you can create a Telegram bot without dealing with the low-level complexities of the Telegram API. Whether it’s polling, sending messages, or handling updates, this package simplifies the process and helps you build bots faster. (node-telegram-bot-api Docs)
If you refer their docs you will understand the first few lines of code
`
// Import the library that lets your Node.js app talk to the Telegram Bot API.
const TelegramBot = require('node-telegram-bot-api');
// replace the value below with the Telegram token you receive from @botfather (use require dotenv)
const token = 'YOUR_TELEGRAM_BOT_TOKEN';
// This creates a new instance of your Telegram bot using the token. Polling true allow bot to use polling method.
const bot = new TelegramBot(token, {polling: true});
`
Start bot by adding bot.on('message', callback) it is like saying: “Hey, whenever someone sends a message to the bot, do something.” that something is the callback
Inside the call back we give some parameter lets say msg. That msg is going to all information regarding the message. i.e who sent it(id), the text, and more. msg.chat.id gets the unique ID of the chat.
while bot.sendMessage is used to send the response to client, when we give it a chatID it will send that text to particular chat (whose chatid is provided)
bot.on('message', (msg) => {
const chatId = msg.chat.id;
bot.sendMessage(chatId, 'Hello, World!');
});
- Now Your bot is sending Hello, World!
NOW HERE'S A WAY TO ESCAPE THE TUTORIAL HELL. YOU CAN REFER THIS GITHUB FOR HELP IF YOU NEED
1) You can create a route that accepts a parameter. You can use msg.text inside bot.on to access the text coming from the client and send that as an argument to the route. Then, you can use that text to send it to Gemini, process the response, and send it back to the client. Well its a simple task
2) Generally Gemini don't remember the previous conversation. You can use your mongoDB skill to store the conversation and then use the stored responses
3) You can set specific commands for your bot to handle. For example, /start can be used to initiate the conversation, and /help can give the user a list of commands or instructions. This helps organize the conversation and lets the user know what your bot can do.
4) Add a feature where users can set reminders or tasks. Your bot can send them notifications or reminders based on certain keywords or commands like /remind me to.... This turns your bot into a personal assistant.
So there you have it! You’ve just created your own Telegram bot. Keep experimenting and adding new features to it. With endless possibilities, your bot can evolve into a real powerhouse. With this, I'm Gulshan Kumar in case I don't see ya, good afternoon, good evening, and good night!