TS2326: Types of property '{0}' are incompatible
TS2326: Types of property '{0}' are incompatible TypeScript is a statically typed superset of JavaScript designed to enhance developer productivity and code quality. In essence, TypeScript extends JavaScript by adding static typing (you define the data types of variables, function parameters, and objects). By catching errors at compile-time, it helps developers write more predictable and error-free code, which is particularly useful in larger or more complex projects. If you're looking to learn TypeScript or explore advanced AI coding tools like GPTeach, consider subscribing to our blog for tips, tutorials, and helpful coding resources. In this article, we will discuss the TypeScript error TS2326: Types of property '{0}' are incompatible, explain why it occurs, and how to resolve it. Before that, we'll take a closer look at what "types" are in TypeScript as they form the foundation of understanding this error. What Are Types in TypeScript? In TypeScript, types are like blueprints that define what kind of value a variable can hold. For example, a variable can be a string, a number, a boolean, or even a combination of multiple types. Types add a layer of safety to your code by ensuring that your variables adhere to the expected structure, which helps prevent runtime errors. Example of Basic Types in TypeScript let name: string = 'John'; // 'name' must hold a string let age: number = 25; // 'age' must hold a number let isActive: boolean = true; // 'isActive' must hold a boolean By defining types explicitly, TypeScript ensures the correct usage of variables and functions. Without proper type definitions, you risk introducing bugs caused by unintended values. With this understanding of types, let’s dive into the error TS2326: Types of property '{0}' are incompatible, its causes, and solutions. Understanding the Error: TS2326: Types of property '{0}' are incompatible The error TS2326: Types of property '{0}' are incompatible occurs when there is a mismatch between the types of a property in two different structures (such as in objects or interfaces). Essentially, TypeScript is telling you that two properties do not align in terms of their type definitions. Let’s break this down with an example. Example 1: Misaligned Property Types Between Interfaces interface Car { make: string; model: string; year: number; } interface ElectricCar { make: string; model: string; year: string; // Here is the issue: 'year' is a string, not a number } const myElectricCar: ElectricCar = { make: 'Tesla', model: 'Model 3', year: '2023' // Since year is 'string', TypeScript will warn us if we use it as a 'Car' }; const compareCars = (car1: Car, car2: Car) => { console.log(car1.make, car2.model); }; // Error: TS2326: Types of property 'year' are incompatible! compareCars(myElectricCar, { make: 'Toyota', model: 'Camry', year: 2020 }); In this example, the year property in ElectricCar is defined as a string, but Car expects year to be a number. When the compareCars function attempts to use myElectricCar (which is an ElectricCar), TypeScript raises an error because year does not match. How to Fix It To resolve this error, make sure the type definitions of the conflicting properties match. Updated Example: Fixing the Incompatibility interface Car { make: string; model: string; year: number; } interface ElectricCar { make: string; model: string; year: number; // Fix the type mismatch: 'year' should be a number } const myElectricCar: ElectricCar = { make: 'Tesla', model: 'Model 3', year: 2023 // Now it matches 'Car' }; const compareCars = (car1: Car, car2: Car) => { console.log(car1.make, car2.model); }; // No error now compareCars(myElectricCar, { make: 'Toyota', model: 'Camry', year: 2020 }); By ensuring that the property types align between Car and ElectricCar, the TS2326: Types of property '{0}' are incompatible error is resolved. Why Does TS2326 Happen? Mismatched Types: The most frequent cause is mismatched types between two objects or interfaces. As shown above, if a property in one structure is defined as a string but the other expects a number, the error occurs. Partial Overlap: Sometimes, you may define an object partially or incorrectly. Incorrect Function Signatures: Passing incompatible arguments to a function parameter can also trigger this error. Important to Know - TypeScript is Strict TypeScript enforces strict type checking to ensure that your code runs as expected, even before runtime. This is why it raises errors like TS2326: Types of property '{0}' are incompatible if there's even a minor mismatch in types. While this might feel restrictive, it prevents bugs that could otherwise crash your code in production. Frequently Asked Questions (FAQs) How Do I Debug TS2326: Types of property '{0}' are inco
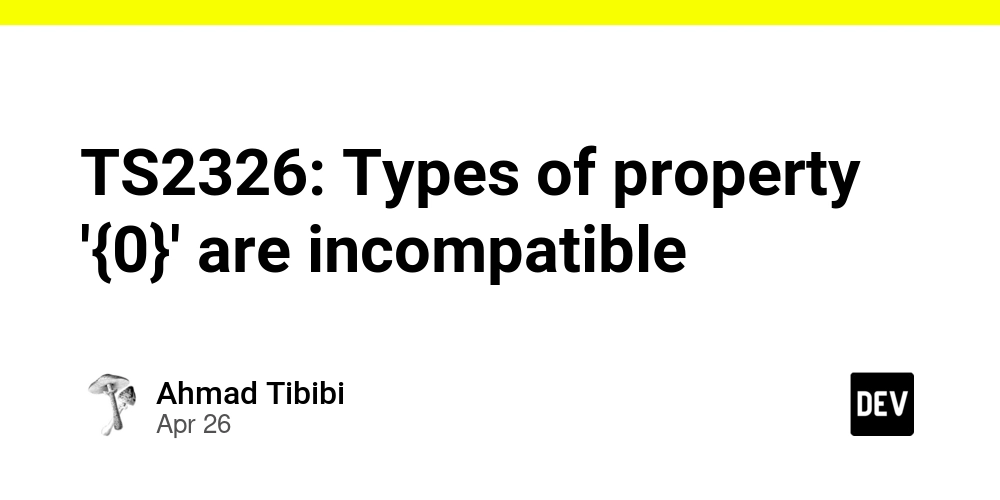
TS2326: Types of property '{0}' are incompatible
TypeScript is a statically typed superset of JavaScript designed to enhance developer productivity and code quality. In essence, TypeScript extends JavaScript by adding static typing (you define the data types of variables, function parameters, and objects). By catching errors at compile-time, it helps developers write more predictable and error-free code, which is particularly useful in larger or more complex projects. If you're looking to learn TypeScript or explore advanced AI coding tools like GPTeach, consider subscribing to our blog for tips, tutorials, and helpful coding resources.
In this article, we will discuss the TypeScript error TS2326: Types of property '{0}' are incompatible, explain why it occurs, and how to resolve it. Before that, we'll take a closer look at what "types" are in TypeScript as they form the foundation of understanding this error.
What Are Types in TypeScript?
In TypeScript, types are like blueprints that define what kind of value a variable can hold. For example, a variable can be a string
, a number
, a boolean
, or even a combination of multiple types. Types add a layer of safety to your code by ensuring that your variables adhere to the expected structure, which helps prevent runtime errors.
Example of Basic Types in TypeScript
let name: string = 'John'; // 'name' must hold a string
let age: number = 25; // 'age' must hold a number
let isActive: boolean = true; // 'isActive' must hold a boolean
By defining types explicitly, TypeScript ensures the correct usage of variables and functions. Without proper type definitions, you risk introducing bugs caused by unintended values.
With this understanding of types, let’s dive into the error TS2326: Types of property '{0}' are incompatible, its causes, and solutions.
Understanding the Error: TS2326: Types of property '{0}' are incompatible
The error TS2326: Types of property '{0}' are incompatible occurs when there is a mismatch between the types of a property in two different structures (such as in objects or interfaces). Essentially, TypeScript is telling you that two properties do not align in terms of their type definitions.
Let’s break this down with an example.
Example 1: Misaligned Property Types Between Interfaces
interface Car {
make: string;
model: string;
year: number;
}
interface ElectricCar {
make: string;
model: string;
year: string; // Here is the issue: 'year' is a string, not a number
}
const myElectricCar: ElectricCar = {
make: 'Tesla',
model: 'Model 3',
year: '2023' // Since year is 'string', TypeScript will warn us if we use it as a 'Car'
};
const compareCars = (car1: Car, car2: Car) => {
console.log(car1.make, car2.model);
};
// Error: TS2326: Types of property 'year' are incompatible!
compareCars(myElectricCar, { make: 'Toyota', model: 'Camry', year: 2020 });
In this example, the year
property in ElectricCar
is defined as a string
, but Car
expects year
to be a number
. When the compareCars
function attempts to use myElectricCar
(which is an ElectricCar
), TypeScript raises an error because year
does not match.
How to Fix It
To resolve this error, make sure the type definitions of the conflicting properties match.
Updated Example: Fixing the Incompatibility
interface Car {
make: string;
model: string;
year: number;
}
interface ElectricCar {
make: string;
model: string;
year: number; // Fix the type mismatch: 'year' should be a number
}
const myElectricCar: ElectricCar = {
make: 'Tesla',
model: 'Model 3',
year: 2023 // Now it matches 'Car'
};
const compareCars = (car1: Car, car2: Car) => {
console.log(car1.make, car2.model);
};
// No error now
compareCars(myElectricCar, { make: 'Toyota', model: 'Camry', year: 2020 });
By ensuring that the property types align between Car
and ElectricCar
, the TS2326: Types of property '{0}' are incompatible error is resolved.
Why Does TS2326 Happen?
Mismatched Types: The most frequent cause is mismatched types between two objects or interfaces. As shown above, if a property in one structure is defined as a
string
but the other expects anumber
, the error occurs.Partial Overlap: Sometimes, you may define an object partially or incorrectly.
Incorrect Function Signatures: Passing incompatible arguments to a function parameter can also trigger this error.
Important to Know - TypeScript is Strict
TypeScript enforces strict type checking to ensure that your code runs as expected, even before runtime. This is why it raises errors like TS2326: Types of property '{0}' are incompatible if there's even a minor mismatch in types. While this might feel restrictive, it prevents bugs that could otherwise crash your code in production.
Frequently Asked Questions (FAQs)
How Do I Debug TS2326: Types of property '{0}' are incompatible?
- Step 1: Check the type definition of the property mentioned in the error.
- Step 2: Ensure that all relevant definitions align (e.g., interface-to-interface or parameter-to-function-signature).
- Step 3: Make corrections to ensure uniformity across type declarations.
Can I Avoid TS2326 by Disabling Type Checking?
While you can loosen TypeScript's strict rules using compiler options (e.g., strict: false
), it is not recommended. Correctly resolving type conflicts makes your codebase more reliable and maintainable.
What Tools Can Help Debug Type Issues?
Tools like IDEs (Visual Studio Code) and AI learning platforms like GPTeach can help you identify and resolve TypeScript type issues effectively.
Important to Know - Type Definitions Help Avoid future Errors
Clearly defining types and interfaces for your code ensures that later changes to your codebase do not lead to unexpected errors. Use strict definitions for objects, parameters, and return types to avoid confusion.
Summary List: Solving TS2326
- Compare the property types in conflicting definitions.
- Ensure consistent type definitions (e.g., between interfaces or objects).
- Use TypeScript's static analysis tools to catch these issues early.
By applying these solutions, you’ll be able to resolve the TS2326: Types of property '{0}' are incompatible error and better understand how TypeScript handles type compatibility.
Remember, errors like these are opportunities to improve your understanding of static typing and write more robust code. If you'd like step-by-step guidance, revisit the basics of TypeScript or consider AI-based platforms like GPTeach to sharpen your skills.