Getting Started with Angular Signals: A Beginner’s Guide Part 1
Angular is evolving, and one of its most exciting new features are Signals. Signals offer a fresh approach to reactive programming by providing a simpler, more efficient way to manage and track state changes. In this guide, we’ll introduce you to Angular Signals, explore their benefits, and show you how to get started with practical code examples. Introduction Angular Signals are a new reactive primitive that encapsulates a value and automatically notifies the application when that value changes. Unlike traditional change detection techniques or even RxJS observables, signals simplify state management by reducing boilerplate code and providing direct access to updated values without the need for manual subscriptions. In this post, we’ll cover: What Angular Signals are and why they’re useful. The difference between writable and computed signals. Practical examples to demonstrate how signals can be implemented in your components. Best practices and common pitfalls when using signals. What Are Angular Signals? At its core, a signal is like a box that holds a value. When the value inside the box changes, Angular automatically notifies any part of your app that depends on it. There are two main types of signals: Writable Signals: These hold a value that you can update directly. They work like regular variables but with automatic change detection. Computed Signals: These derive their value from one or more other signals. When any of the underlying signals change, the computed signal automatically recalculates its value. A Simple Counter Example Let’s look at a basic example of a counter using a writable signal: import { Component, signal } from '@angular/core'; @Component({ selector: 'app-counter', template: ` Counter: {{ counter() }} Increment `, }) export class CounterComponent { // Create a writable signal initialized to 0 counter = signal(0); // Update the signal using the update method increment() { this.counter.update(value => value + 1); } } In this example, calling counter() reads the current value, and the increment() method updates it. Angular takes care of updating the view whenever the signal’s value changes. Benefits of Using Signals Angular Signals bring several advantages: Simplicity: With signals, there is no need to subscribe or unsubscribe manually. Reading a signal is as simple as calling it like a function. Performance: Signals allow for fine-grained reactivity, meaning Angular updates only the parts of the UI that depend on the changed value. Reduced Boilerplate: Signals eliminate the need for extensive RxJS code in many cases, making your codebase easier to understand and maintain. Setting Up Your Angular Environment Before you start using signals, ensure you have Angular 16 or later installed. You can set up a new Angular project with the Angular CLI: npm install -g @angular/cli ng new angular-signals-demo cd angular-signals-demo ng serve Once your project is running, you’re ready to integrate signals into your components. Implementing Signals in Your Application Using Writable Signals Writable signals are perfect for storing and updating state directly. In our counter example, we saw how to create a writable signal with signal(0) and update it using update(). Creating Computed Signals Computed signals allow you to derive a new value based on one or more signals. Here’s an example that builds on our counter to create a computed signal: import { Component, signal, computed } from '@angular/core'; @Component({ selector: 'app-double-counter', template: ` Counter: {{ counter() }} Double: {{ doubleCounter() }} Increment `, }) export class DoubleCounterComponent { // Writable signal for the counter counter = signal(0); // Computed signal that doubles the counter value doubleCounter = computed(() => this.counter() * 2); increment() { this.counter.update(value => value + 1); } } In this snippet, doubleCounter automatically recalculates whenever counter is updated. Best Practices and Common Pitfalls While signals simplify reactivity, keep these tips in mind: Avoid Direct Mutations: Always use the provided methods (set(), update()) to change signal values. This ensures that Angular’s change detection is properly triggered. Be Cautious with Computed Signals: Ensure that any signal used within a computed function is always read during the computation to maintain proper dependencies. Integrate Gradually: If you’re transitioning from RxJS, consider using Angular’s toSignal and toObservable utilities to mix signals with observables until you’re comfortable with the new pattern. However, be aware that toObservable will break the reactivity graph, which can lead to unexpected behavior. We will explore this issue further in Part 2. Advanced technique
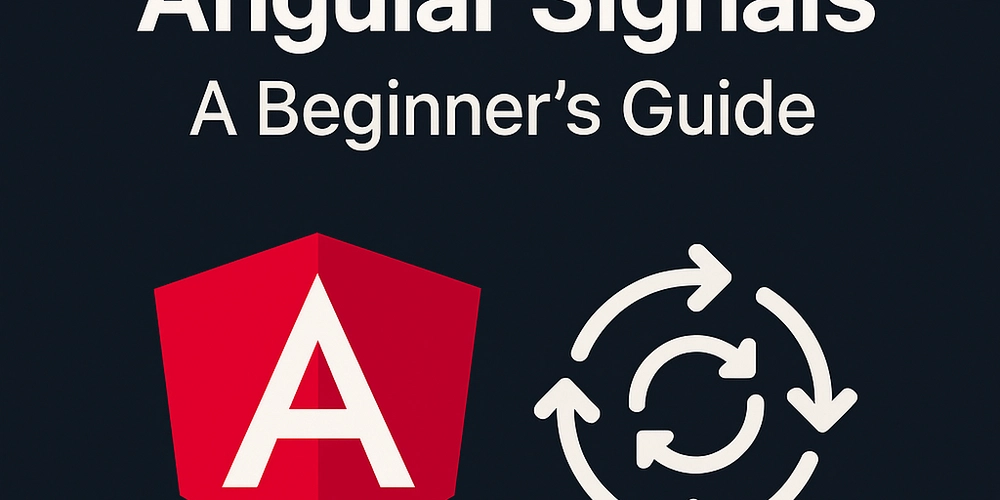
Angular is evolving, and one of its most exciting new features are Signals. Signals offer a fresh approach to reactive programming by providing a simpler, more efficient way to manage and track state changes. In this guide, we’ll introduce you to Angular Signals, explore their benefits, and show you how to get started with practical code examples.
Introduction
Angular Signals are a new reactive primitive that encapsulates a value and automatically notifies the application when that value changes. Unlike traditional change detection techniques or even RxJS observables, signals simplify state management by reducing boilerplate code and providing direct access to updated values without the need for manual subscriptions.
In this post, we’ll cover:
- What Angular Signals are and why they’re useful.
- The difference between writable and computed signals.
- Practical examples to demonstrate how signals can be implemented in your components.
- Best practices and common pitfalls when using signals.
What Are Angular Signals?
At its core, a signal is like a box that holds a value. When the value inside the box changes, Angular automatically notifies any part of your app that depends on it.
There are two main types of signals:
- Writable Signals: These hold a value that you can update directly. They work like regular variables but with automatic change detection.
- Computed Signals: These derive their value from one or more other signals. When any of the underlying signals change, the computed signal automatically recalculates its value.
A Simple Counter Example
Let’s look at a basic example of a counter using a writable signal:
import { Component, signal } from '@angular/core';
@Component({
selector: 'app-counter',
template: `
Counter: {{ counter() }}
`,
})
export class CounterComponent {
// Create a writable signal initialized to 0
counter = signal(0);
// Update the signal using the update method
increment() {
this.counter.update(value => value + 1);
}
}
In this example, calling counter()
reads the current value, and the increment()
method updates it. Angular takes care of updating the view whenever the signal’s value changes.
Benefits of Using Signals
Angular Signals bring several advantages:
- Simplicity: With signals, there is no need to subscribe or unsubscribe manually. Reading a signal is as simple as calling it like a function.
- Performance: Signals allow for fine-grained reactivity, meaning Angular updates only the parts of the UI that depend on the changed value.
- Reduced Boilerplate: Signals eliminate the need for extensive RxJS code in many cases, making your codebase easier to understand and maintain.
Setting Up Your Angular Environment
Before you start using signals, ensure you have Angular 16 or later installed. You can set up a new Angular project with the Angular CLI:
npm install -g @angular/cli
ng new angular-signals-demo
cd angular-signals-demo
ng serve
Once your project is running, you’re ready to integrate signals into your components.
Implementing Signals in Your Application
Using Writable Signals
Writable signals are perfect for storing and updating state directly. In our counter example, we saw how to create a writable signal with signal(0)
and update it using update()
.
Creating Computed Signals
Computed signals allow you to derive a new value based on one or more signals. Here’s an example that builds on our counter to create a computed signal:
import { Component, signal, computed } from '@angular/core';
@Component({
selector: 'app-double-counter',
template: `
Counter: {{ counter() }}
Double: {{ doubleCounter() }}
`,
})
export class DoubleCounterComponent {
// Writable signal for the counter
counter = signal(0);
// Computed signal that doubles the counter value
doubleCounter = computed(() => this.counter() * 2);
increment() {
this.counter.update(value => value + 1);
}
}
In this snippet, doubleCounter
automatically recalculates whenever counter
is updated.
Best Practices and Common Pitfalls
While signals simplify reactivity, keep these tips in mind:
-
Avoid Direct Mutations: Always use the provided methods (
set()
,update()
) to change signal values. This ensures that Angular’s change detection is properly triggered. - Be Cautious with Computed Signals: Ensure that any signal used within a computed function is always read during the computation to maintain proper dependencies.
- Integrate Gradually: If you’re transitioning from RxJS, consider using Angular’s toSignal and toObservable utilities to mix signals with observables until you’re comfortable with the new pattern. However, be aware that toObservable will break the reactivity graph, which can lead to unexpected behavior. We will explore this issue further in Part 2.
Advanced techniques
If you want to explore more advanced usage of Angular Signals, including fine-grained reactivity and more complex scenarios, check out this great blog post series: