Error handling in NestJS
What is Error Handling? Error handling is the process of managing and responding to errors that occur during the execution of a program. It involves detecting, handling, and recovering from errors gracefully to ensure the stability and reliability of the application. How Does NestJS Handle Exceptions? When an exception is thrown in JavaScript, whether inside an asynchronous function or synchronous code, it interrupts the normal flow of execution. The JavaScript runtime immediately stops executing the current function and begins to unwind the call stack, looking for a suitable exception handler to handle the error. This is called “exception unwinding”. Here’s how it works: Exception Thrown: When an exception occurs, JavaScript immediately stops executing the current code block and starts to unwind the call stack. Call Stack Unwinding: The JavaScript runtime begins to pop functions off the call stack, returning control to the functions that invoked them. This process continues until the exception is caught by a suitable exception handler or until it reaches the global scope. Exception Handling: If there’s a try-catch block surrounding the code that threw the exception, JavaScript jumps to the appropriate catch block. If there’s no catch block in the current function, JavaScript continues unwinding the call stack, searching for a catch block in the calling function, and so on. Global Exception Filter: If the exception isn’t caught in try-catch anywhere, then the exception filter intercepts and handles it gracefully. However, this doesn’t always work in the case of errors thrown in asynchronous functions. Unhandled Exception: If the exception isn’t caught anywhere above, it becomes an “unhandled rejection”. What Happens When an Exception is Thrown within an Asynchronous Function? In NestJS, the global exception filter plays a crucial role in catching and handling errors that occur within the application. NestJS’s global exception filters operate synchronously, intercepting errors as they propagate up the call stack. However, when it comes to errors thrown in asynchronous functions, the global exception filter might not always catch them as expected. This behaviour occurs because async functions in JavaScript return a Promise. When an error is thrown within an async function, it is encapsulated within that Promise. As a result, the error is not immediately thrown in the calling context but rather captured within the Promise’s rejection handler and the error thrown is queued within the event loop, i.e., it’s deferred until the Promise is either awaited or handled. This means that by the time the error propagates back to the global exception filter, it might have already passed the point where the filter could intercept it. To address this issue, you can manually catch errors within the async functions and handle them appropriately. You can find more information about exception filters in NestJS’s documentation — Exception Filters
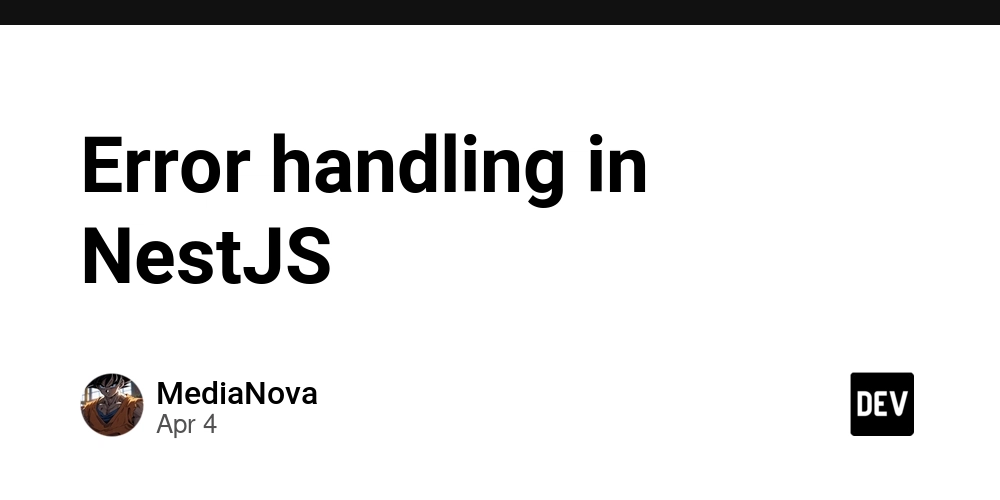
What is Error Handling?
Error handling is the process of managing and responding to errors that occur during the execution of a program. It involves detecting, handling, and recovering from errors gracefully to ensure the stability and reliability of the application.
How Does NestJS Handle Exceptions?
When an exception is thrown in JavaScript, whether inside an asynchronous function or synchronous code, it interrupts the normal flow of execution. The JavaScript runtime immediately stops executing the current function and begins to unwind the call stack, looking for a suitable exception handler to handle the error. This is called “exception unwinding”.
Here’s how it works:
Exception Thrown: When an exception occurs, JavaScript immediately stops executing the current code block and starts to unwind the call stack.
Call Stack Unwinding: The JavaScript runtime begins to pop functions off the call stack, returning control to the functions that invoked them. This process continues until the exception is caught by a suitable exception handler or until it reaches the global scope.
Exception Handling: If there’s a try-catch block surrounding the code that threw the exception, JavaScript jumps to the appropriate catch block. If there’s no catch block in the current function, JavaScript continues unwinding the call stack, searching for a catch block in the calling function, and so on.
Global Exception Filter: If the exception isn’t caught in try-catch anywhere, then the exception filter intercepts and handles it gracefully. However, this doesn’t always work in the case of errors thrown in asynchronous functions.
Unhandled Exception: If the exception isn’t caught anywhere above, it becomes an “unhandled rejection”.
What Happens When an Exception is Thrown within an Asynchronous Function?
In NestJS, the global exception filter plays a crucial role in catching and handling errors that occur within the application. NestJS’s global exception filters operate synchronously, intercepting errors as they propagate up the call stack. However, when it comes to errors thrown in asynchronous functions, the global exception filter might not always catch them as expected.
This behaviour occurs because async functions in JavaScript return a Promise. When an error is thrown within an async function, it is encapsulated within that Promise. As a result, the error is not immediately thrown in the calling context but rather captured within the Promise’s rejection handler and the error thrown is queued within the event loop, i.e., it’s deferred until the Promise is either awaited or handled. This means that by the time the error propagates back to the global exception filter, it might have already passed the point where the filter could intercept it.
To address this issue, you can manually catch errors within the async functions and handle them appropriately.
You can find more information about exception filters in NestJS’s documentation — Exception Filters