Dockerize Laravel Vue Application! - By Ariful Haque Sajib
Dockerize laravel vue application — by Ariful Haque Sajib . In this blog, I’ll walk you through setting up a Laravel 11 and Vue.js 3 with Inertia.js project in a Dockerized environment. The setup orchestrates PHP, NGINX, and MySQL services, creating a smooth development experience. Let’s break it all down. Folder Structure Here’s the folder structure for our project: This structure keeps our Docker-specific files (docker/) separate from the application code (src/). The src/ The folder contains both the Laravel backend and the Vue.js frontend, which are integrated with Breeze for authentication. In Collapse Setting Up Laravel and Vue.js I use the Laravel Global installer to install the Laravel Vue Inertia application using the steps below. docker-compose.yml docker-compose.yml defines and configures three essential services: PHP, NGINX, and MySQL. version: '3.8' services: app: build: context: ./docker/php container_name: laravel-vue-app volumes: - ./src:/var/www/html ports: - "9000:9000" networks: - laravel web: image: nginx:latest container_name: laravel-vue-nginx ports: - "8081:80" volumes: - ./src:/var/www/html - ./docker/nginx/default.conf:/etc/nginx/conf.d/default.conf depends_on: - app networks: - laravel db: image: mysql:8.0 container_name: laravel-vue-db environment: MYSQL_ROOT_PASSWORD: root MYSQL_DATABASE: laravel MYSQL_USER: laravel MYSQL_PASSWORD: laravel volumes: - db-data:/var/lib/mysql ports: - "3307:3306" networks: - laravel volumes: db-data: networks: laravel: Key Points: PHP Service (app): Uses the Dockerfile in docker/php to build the PHP image. Mounts the src/ folder to /var/www/html inside the container for live syncing. Exposes port 9000 for PHP-FPM. NGINX Service (web): Serves the Laravel application from /var/www/html/public. Maps the host port 8081 to the container port 80. Relies on a custom configuration file default.conf. MySQL Service (db): Runs MySQL version 8.0. Sets up environment variables for database credentials. Stores data in a Docker-managed volume db-data. Networks and Volumes: A custom laravel network connects all services. A named volume db-data persists the MySQL database data. PHP Dockerfile The Dockerfile in docker/php/ defines the PHP environment for Laravel. FROM php:8.2-fpm Install required dependencies RUN apt-get update && apt-get install -y \ git \ unzip \ curl \ libpng-dev \ libonig-dev \ libxml2-dev \ zip \ libzip-dev \ && docker-php-ext-install pdo_mysql mbstring exif pcntl bcmath gd zip Install Composer COPY --from=composer:2.6 /usr/bin/composer /usr/bin/composer Set working directory WORKDIR /var/www/html Key Points: Base Image: Uses the official php:8.2-fpm image optimized for Laravel. Dependencies: Installs required extensions like pdo_mysql for MySQL, gd for image processing, and zip for file compression. Composer: Adds Composer for dependency management. Working Directory: Sets the working directory to /var/www/html. NGINX Configuration The NGINX configuration file default.conf is placed inside docker/nginx/. server { listen 80; server_name localhost; index index.php index.html; root /var/www/html/public; location / { try_files $uri $uri/ /index.php?$query_string; } location ~ \.php$ { include fastcgi_params; fastcgi_pass app:9000; fastcgi_index index.php; fastcgi_param SCRIPT_FILENAME $document_root$fastcgi_script_name; } location ~ /\.ht { deny all; } } Key Points: Root Directory: Points to Laravel’s public/ folder. PHP Requests: Forwards .php requests to the PHP service (app) on port 9000. Security: Denies access to hidden files (e.g., .htaccess). Environment Setup .env copy and paste in Laravel .env file DB_CONNECTION=mysql DB_HOST=db DB_PORT=3306 DB_DATABASE=laravel DB_USERNAME=laravel DB_PASSWORD=laravel Running the Docker Containers Start the Docker services with docker-compose: docker-compose up -d Access the Application: Laravel App: http://localhost:8081 MySQL: Accessible on port 3307. Docker Commands Start containers docker-compose up -d Stop containers docker-compose down View container logs docker-compose logs -f Access PHP container shell docker-compose exec laravel-vue-app bash List running containers docker-compose ps Conclusion This setup ensures that Laravel and Vue.js run seamlessly in a Dockerized environment. It separates concerns, making it easy to manage and scale. With services like PHP, NGINX, and MySQL running in isolated containers, your development workflow becomes efficient and predictable. Feel free to tweak this setup based on your project’s needs. Happy coding!
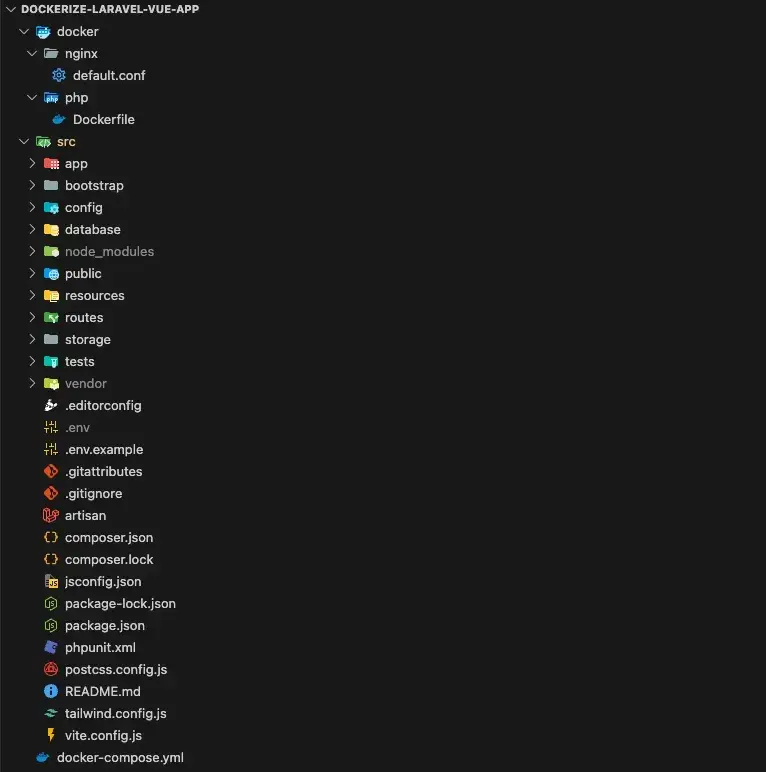
Dockerize laravel vue application — by Ariful Haque Sajib .
In this blog, I’ll walk you through setting up a Laravel 11 and Vue.js 3 with Inertia.js project in a Dockerized environment. The setup orchestrates PHP, NGINX, and MySQL services, creating a smooth development experience. Let’s break it all down.
Folder Structure
Here’s the folder structure for our project:
This structure keeps our Docker-specific files (docker/) separate from the application code (src/). The src/ The folder contains both the Laravel backend and the Vue.js frontend, which are integrated with Breeze for authentication.
In Collapse
- Setting Up Laravel and Vue.js I use the Laravel Global installer to install the Laravel Vue Inertia application using the steps below.
- docker-compose.yml docker-compose.yml defines and configures three essential services: PHP, NGINX, and MySQL.
version: '3.8'
services:
app:
build:
context: ./docker/php
container_name: laravel-vue-app
volumes:
- ./src:/var/www/html
ports:
- "9000:9000"
networks:
- laravel
web:
image: nginx:latest
container_name: laravel-vue-nginx
ports:
- "8081:80"
volumes:
- ./src:/var/www/html
- ./docker/nginx/default.conf:/etc/nginx/conf.d/default.conf
depends_on:
- app
networks:
- laravel
db:
image: mysql:8.0
container_name: laravel-vue-db
environment:
MYSQL_ROOT_PASSWORD: root
MYSQL_DATABASE: laravel
MYSQL_USER: laravel
MYSQL_PASSWORD: laravel
volumes:
- db-data:/var/lib/mysql
ports:
- "3307:3306"
networks:
- laravel
volumes:
db-data:
networks:
laravel:
Key Points:
PHP Service (app):
Uses the Dockerfile in docker/php to build the PHP image.
Mounts the src/ folder to /var/www/html inside the container for live syncing.
Exposes port 9000 for PHP-FPM.
NGINX Service (web):
Serves the Laravel application from /var/www/html/public.
Maps the host port 8081 to the container port 80.
Relies on a custom configuration file default.conf.
MySQL Service (db):
Runs MySQL version 8.0.
Sets up environment variables for database credentials.
Stores data in a Docker-managed volume db-data.
Networks and Volumes:
A custom laravel network connects all services.
A named volume db-data persists the MySQL database data.
- PHP Dockerfile The Dockerfile in docker/php/ defines the PHP environment for Laravel.
FROM php:8.2-fpm
Install required dependencies
RUN apt-get update && apt-get install -y \
git \
unzip \
curl \
libpng-dev \
libonig-dev \
libxml2-dev \
zip \
libzip-dev \
&& docker-php-ext-install pdo_mysql mbstring exif pcntl bcmath gd zip
Install Composer
COPY --from=composer:2.6 /usr/bin/composer /usr/bin/composer
Set working directory
WORKDIR /var/www/html
Key Points:
- Base Image: Uses the official php:8.2-fpm image optimized for Laravel.
- Dependencies: Installs required extensions like pdo_mysql for MySQL, gd for image processing, and zip for file compression.
- Composer: Adds Composer for dependency management.
Working Directory: Sets the working directory to /var/www/html.
NGINX Configuration
The NGINX configuration file default.conf is placed inside docker/nginx/.
server {
listen 80;
server_name localhost;
index index.php index.html;
root /var/www/html/public;
location / {
try_files $uri $uri/ /index.php?$query_string;
}
location ~ \.php$ {
include fastcgi_params;
fastcgi_pass app:9000;
fastcgi_index index.php;
fastcgi_param SCRIPT_FILENAME $document_root$fastcgi_script_name;
}
location ~ /\.ht {
deny all;
}
}
Key Points:
Root Directory: Points to Laravel’s public/ folder.
PHP Requests: Forwards .php requests to the PHP service (app) on port 9000.
Security: Denies access to hidden files (e.g., .htaccess).
- Environment Setup .env copy and paste in Laravel .env file
DB_CONNECTION=mysql
DB_HOST=db
DB_PORT=3306
DB_DATABASE=laravel
DB_USERNAME=laravel
DB_PASSWORD=laravel
- Running the Docker Containers Start the Docker services with docker-compose:
docker-compose up -d
Access the Application:
Laravel App: http://localhost:8081
MySQL: Accessible on port 3307.
Docker Commands
Start containers
docker-compose up -d
Stop containers
docker-compose down
View container logs
docker-compose logs -f
Access PHP container shell
docker-compose exec laravel-vue-app bash
List running containers
docker-compose ps
Conclusion
This setup ensures that Laravel and Vue.js run seamlessly in a Dockerized environment. It separates concerns, making it easy to manage and scale. With services like PHP, NGINX, and MySQL running in isolated containers, your development workflow becomes efficient and predictable.
Feel free to tweak this setup based on your project’s needs. Happy coding!