Deep Dive into Angular Dependency Injection (DI)
Dependency Injection (DI) is a fundamental aspect of Angular, significantly affecting application scalability, maintainability, and testability. For middle to senior developers, a deeper understanding beyond the basics of DI can enhance application architecture and design. Quick Recap: What is Dependency Injection? DI is a design pattern where dependencies are injected into a class rather than the class creating its dependencies. This promotes modularity and facilitates easier testing and maintainability. Comparison: Basic vs. Advanced DI Concepts Your previous article provided foundational concepts and essential practices. Let's briefly recap and then dive deeper into more advanced aspects of DI: Aspect Basic Understanding Advanced Understanding (Senior Level) Providers Define how services are created and their scope (root, module, component). Use advanced providers like factory providers and multi-providers to manage complex dependency scenarios dynamically. Injectors Angular uses hierarchical injectors for managing dependency scopes. Leverage injector hierarchies strategically to optimize performance and encapsulation, creating tailored injector trees for specific application parts. Services Classes encapsulating reusable logic, typically injected into components. Design services carefully, managing state and side effects clearly, and ensuring they are appropriately scoped to reduce memory leaks and complexity. Injection Tokens Used for injecting simple values and configurations. Implement flexible and dynamic configurations with Injection Tokens, providing runtime flexibility and reducing tightly coupled configuration management. Deep Dive: Advanced Dependency Injection Techniques Multi-Providers: Injecting Multiple Implementations Multi-providers enable injecting multiple dependencies associated with a single token. A common scenario is HTTP interceptors: providers: [ { provide: HTTP_INTERCEPTORS, useClass: AuthInterceptor, multi: true }, { provide: HTTP_INTERCEPTORS, useClass: LoggingInterceptor, multi: true } ] Factory Providers: Dynamic Dependency Resolution Factory providers dynamically instantiate dependencies based on runtime conditions: providers: [{ provide: LoggerService, useFactory: () => isDevMode() ? new DevLogger() : new ProdLogger() }] Injection Tokens for Configuration Use Injection Tokens for flexible and decoupled configuration management: export const API_ENDPOINT = new InjectionToken('API_ENDPOINT'); providers: [{ provide: API_ENDPOINT, useValue: 'https://api.example.com' }] Best Practices for Advanced Developers Advanced Provider Patterns: Utilize complex provider configurations to manage sophisticated application requirements. Injector Hierarchies: Carefully manage injector lifecycles, keeping service instances scoped efficiently. Dynamic Injection: Leverage runtime decisions to inject services, enhancing application flexibility and adaptability. Common Pitfalls to Avoid Avoid over-engineering DI solutions; use complexity only when genuinely needed. Prevent memory leaks by carefully managing provider scopes and service lifetimes. Maintain clear documentation of complex DI strategies to support ongoing maintainability. Conclusion Mastering advanced Dependency Injection techniques empowers Angular developers to create robust, scalable, and highly maintainable applications. By carefully managing providers, injectors, and services, senior-level developers can greatly enhance application performance and flexibility.
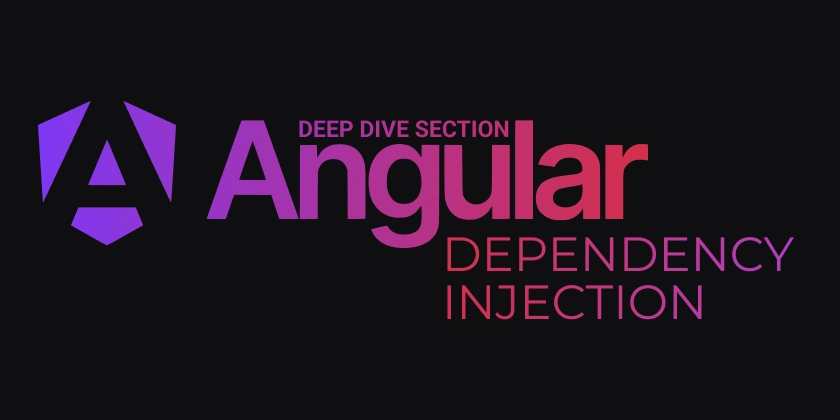
Dependency Injection (DI) is a fundamental aspect of Angular, significantly affecting application scalability, maintainability, and testability. For middle to senior developers, a deeper understanding beyond the basics of DI can enhance application architecture and design.
Quick Recap: What is Dependency Injection?
DI is a design pattern where dependencies are injected into a class rather than the class creating its dependencies. This promotes modularity and facilitates easier testing and maintainability.
Comparison: Basic vs. Advanced DI Concepts
Your previous article provided foundational concepts and essential practices. Let's briefly recap and then dive deeper into more advanced aspects of DI:
Aspect | Basic Understanding | Advanced Understanding (Senior Level) |
---|---|---|
Providers | Define how services are created and their scope (root, module, component). | Use advanced providers like factory providers and multi-providers to manage complex dependency scenarios dynamically. |
Injectors | Angular uses hierarchical injectors for managing dependency scopes. | Leverage injector hierarchies strategically to optimize performance and encapsulation, creating tailored injector trees for specific application parts. |
Services | Classes encapsulating reusable logic, typically injected into components. | Design services carefully, managing state and side effects clearly, and ensuring they are appropriately scoped to reduce memory leaks and complexity. |
Injection Tokens | Used for injecting simple values and configurations. | Implement flexible and dynamic configurations with Injection Tokens, providing runtime flexibility and reducing tightly coupled configuration management. |
Deep Dive: Advanced Dependency Injection Techniques
Multi-Providers: Injecting Multiple Implementations
Multi-providers enable injecting multiple dependencies associated with a single token. A common scenario is HTTP interceptors:
providers: [
{ provide: HTTP_INTERCEPTORS, useClass: AuthInterceptor, multi: true },
{ provide: HTTP_INTERCEPTORS, useClass: LoggingInterceptor, multi: true }
]
Factory Providers: Dynamic Dependency Resolution
Factory providers dynamically instantiate dependencies based on runtime conditions:
providers: [{
provide: LoggerService,
useFactory: () => isDevMode() ? new DevLogger() : new ProdLogger()
}]
Injection Tokens for Configuration
Use Injection Tokens for flexible and decoupled configuration management:
export const API_ENDPOINT = new InjectionToken<string>('API_ENDPOINT');
providers: [{ provide: API_ENDPOINT, useValue: 'https://api.example.com' }]
Best Practices for Advanced Developers
- Advanced Provider Patterns: Utilize complex provider configurations to manage sophisticated application requirements.
- Injector Hierarchies: Carefully manage injector lifecycles, keeping service instances scoped efficiently.
- Dynamic Injection: Leverage runtime decisions to inject services, enhancing application flexibility and adaptability.
Common Pitfalls to Avoid
- Avoid over-engineering DI solutions; use complexity only when genuinely needed.
- Prevent memory leaks by carefully managing provider scopes and service lifetimes.
- Maintain clear documentation of complex DI strategies to support ongoing maintainability.
Conclusion
Mastering advanced Dependency Injection techniques empowers Angular developers to create robust, scalable, and highly maintainable applications. By carefully managing providers, injectors, and services, senior-level developers can greatly enhance application performance and flexibility.