Day 6:Parameters vs Arguments
Parameters vs. Arguments in Java Methods Key Difference Term Definition When Used Example Parameter Variables listed in the method declaration When defining a method void print(int num) Argument Actual values passed when calling the method When calling a method print(10) 1. Parameters (Formal Parameters) Definition: Variables declared in the method signature. Act as placeholders for the values that will be passed. Scope: Local to the method. Example: // 'name' and 'age' are parameters void greet(String name, int age) { System.out.println("Hello, " + name + "! Age: " + age); } 2. Arguments (Actual Parameters) Definition: Real values passed to the method when invoked. Must match the parameter type and order. Example: public class Main { public static void main(String[] args) { greet("Alice", 25); // "Alice" and 25 are arguments } static void greet(String name, int age) { // Parameters System.out.println("Hello, " + name + "! Age: " + age); } } Output: Hello, Alice! Age: 25 3. Parameter Passing Mechanisms (A) Pass by Value (Primitive Types) Java copies the value of the argument. Changes inside the method do not affect the original variable. void increment(int x) { // x is a copy of 'num' x++; System.out.println("Inside method: " + x); // 11 } public static void main(String[] args) { int num = 10; increment(num); System.out.println("Outside method: " + num); // 10 (unchanged) } (B) Pass by Reference Value (Objects) For objects, Java passes a copy of the reference (memory address). Changes to the object's state are reflected outside. class Student { String name; Student(String name) { this.name = name; } } void changeName(Student s) { // s points to the same object s.name = "Bob"; } public static void main(String[] args) { Student student = new Student("Alice"); changeName(student); System.out.println(student.name); // "Bob" (modified) } 4. Variable-Length Arguments (Varargs) Allows a method to accept any number of arguments of the same type. Syntax: Type... variableName. Example: int sum(int... numbers) { // Accepts 0 or more ints int total = 0; for (int num : numbers) { total += num; } return total; } public static void main(String[] args) { System.out.println(sum(1, 2, 3)); // 6 System.out.println(sum(10, 20)); // 30 } 5. Common Mistakes ❌ Mismatched Argument Types void printLength(String text) { ... } printLength(10); // ❌ Error (int passed, String expected) ❌ Incorrect Parameter Order void register(String name, int age) { ... } register(25, "Alice"); // ❌ Error (parameters swapped) ❌ Assuming Primitive Arguments Are Modified void swap(int a, int b) { // ❌ Won't affect original variables int temp = a; a = b; b = temp; } 6. Best Practices Use descriptive names for parameters (username instead of s). Keep parameter lists short (max 3-4). Use objects if needed. Document parameters with JavaDoc: /** * Calculates the area of a rectangle. * @param length The length of the rectangle. * @param width The width of the rectangle. */ double area(double length, double width) { ... } Summary Table Concept Description Example Parameter Variable in method declaration void log(String message) Argument Value passed during method call log("Error!") Pass by Value Primitive types are copied int x = 10; modify(x) Pass by Reference Value Objects share the same reference Student s; changeName(s) Varargs Accepts variable arguments int sum(int... nums) Final Quiz public class Test { static void update(int[] arr) { arr[0] = 100; // Modifies the original array } public static void main(String[] args) { int[] numbers = {1, 2, 3}; update(numbers); System.out.println(numbers[0]); // What's the output? } } Answer: 100 (arrays are objects, so changes persist). Let me know if you'd like to explore method overloading next!
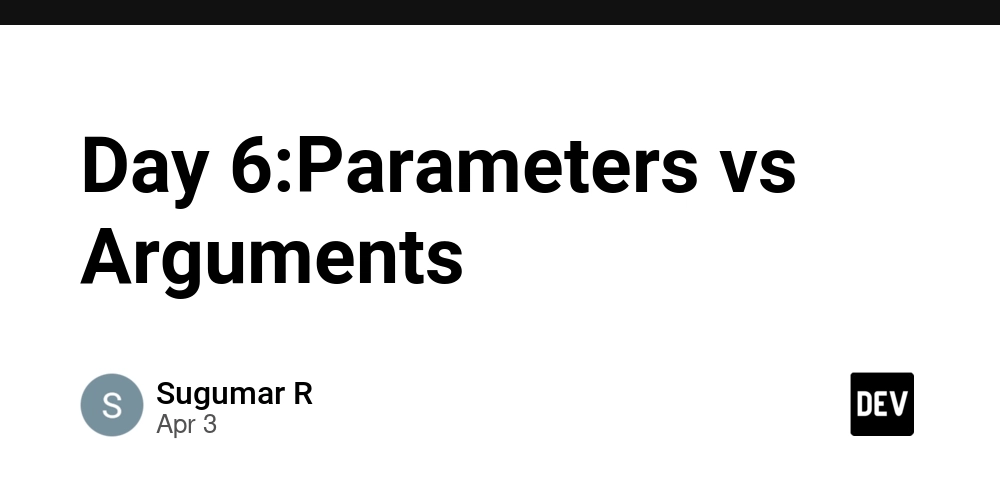
Parameters vs. Arguments in Java Methods
Key Difference
Term | Definition | When Used | Example |
---|---|---|---|
Parameter | Variables listed in the method declaration | When defining a method | void print(int num) |
Argument | Actual values passed when calling the method | When calling a method | print(10) |
1. Parameters (Formal Parameters)
- Definition: Variables declared in the method signature.
- Act as placeholders for the values that will be passed.
- Scope: Local to the method.
Example:
// 'name' and 'age' are parameters
void greet(String name, int age) {
System.out.println("Hello, " + name + "! Age: " + age);
}
2. Arguments (Actual Parameters)
- Definition: Real values passed to the method when invoked.
- Must match the parameter type and order.
Example:
public class Main {
public static void main(String[] args) {
greet("Alice", 25); // "Alice" and 25 are arguments
}
static void greet(String name, int age) { // Parameters
System.out.println("Hello, " + name + "! Age: " + age);
}
}
Output:
Hello, Alice! Age: 25
3. Parameter Passing Mechanisms
(A) Pass by Value (Primitive Types)
- Java copies the value of the argument.
- Changes inside the method do not affect the original variable.
void increment(int x) { // x is a copy of 'num'
x++;
System.out.println("Inside method: " + x); // 11
}
public static void main(String[] args) {
int num = 10;
increment(num);
System.out.println("Outside method: " + num); // 10 (unchanged)
}
(B) Pass by Reference Value (Objects)
- For objects, Java passes a copy of the reference (memory address).
- Changes to the object's state are reflected outside.
class Student {
String name;
Student(String name) { this.name = name; }
}
void changeName(Student s) { // s points to the same object
s.name = "Bob";
}
public static void main(String[] args) {
Student student = new Student("Alice");
changeName(student);
System.out.println(student.name); // "Bob" (modified)
}
4. Variable-Length Arguments (Varargs)
- Allows a method to accept any number of arguments of the same type.
- Syntax:
Type... variableName
.
Example:
int sum(int... numbers) { // Accepts 0 or more ints
int total = 0;
for (int num : numbers) {
total += num;
}
return total;
}
public static void main(String[] args) {
System.out.println(sum(1, 2, 3)); // 6
System.out.println(sum(10, 20)); // 30
}
5. Common Mistakes
❌ Mismatched Argument Types
void printLength(String text) { ... }
printLength(10); // ❌ Error (int passed, String expected)
❌ Incorrect Parameter Order
void register(String name, int age) { ... }
register(25, "Alice"); // ❌ Error (parameters swapped)
❌ Assuming Primitive Arguments Are Modified
void swap(int a, int b) { // ❌ Won't affect original variables
int temp = a;
a = b;
b = temp;
}
6. Best Practices
-
Use descriptive names for parameters (
username
instead ofs
). - Keep parameter lists short (max 3-4). Use objects if needed.
- Document parameters with JavaDoc:
/**
* Calculates the area of a rectangle.
* @param length The length of the rectangle.
* @param width The width of the rectangle.
*/
double area(double length, double width) { ... }
Summary Table
Concept | Description | Example |
---|---|---|
Parameter | Variable in method declaration | void log(String message) |
Argument | Value passed during method call | log("Error!") |
Pass by Value | Primitive types are copied | int x = 10; modify(x) |
Pass by Reference Value | Objects share the same reference | Student s; changeName(s) |
Varargs | Accepts variable arguments | int sum(int... nums) |
Final Quiz
public class Test {
static void update(int[] arr) {
arr[0] = 100; // Modifies the original array
}
public static void main(String[] args) {
int[] numbers = {1, 2, 3};
update(numbers);
System.out.println(numbers[0]); // What's the output?
}
}
Answer: 100
(arrays are objects, so changes persist).
Let me know if you'd like to explore method overloading next!