Day-11:Class and Object
class A Class is like an object constructor, or a "blueprint" for creating objects. Create a class named "Main" with a variable x: public class Main { int x = 5; } Object In Java, an object is created from a class. We have already created the class named Main, so now we can use this to create objects. To create an object of Main, specify the class name, followed by the object name, and use the keyword new Object: Combination of States and Behaviours Example Create an object called "myObj" and print the value of x: public class Main { int x = 5; public static void main(String[] args) { Main myObj = new Main(); System.out.println(myObj.x); } } Multiple object You can create multiple objects of one class: public class Main { int x = 5; public static void main(String[] args) { Main myObj1 = new Main(); // Object 1 Main myObj2 = new Main(); // Object 2 System.out.println(myObj1.x); System.out.println(myObj2.x); } } Multiple class You can also create an object of a class and access it in another class. This is often used for better organization of classes (one class has all the attributes and methods, while the other class holds the main() method (code to be executed)). Remember that the name of the java file should match the class name. In this example, we have created two files in the same directory/folder: 1.Main.java 2.Second.java Main.java public class Main { int x = 5; } Second.java class Second { public static void main(String[] args) { Main myObj = new Main(); System.out.println(myObj.x); } } Naming conventions 1) Class name should start with capital Letter Eg. Calculator my first java program 2) Should Follow Camel Case MyFirstJavaProgram, My_First_Java_Program 3) Should not start with Numbers Eg. 2Calculator --> Calculator2 4) No Special Characters except _, $ 5) MEANINGFUL NAME 6) Class Names should be Nouns, should not be Verbs. Eg. Browser, Browse is not correct. Naming Convention: Class -> First letter CAPITAL Interface String is a class. int, float, boolean - Not classes Example 1.Theatre public class Theatre { int ticket_fare; //int float show_time; //float String movie_name; //String boolean on_screen; //boolean public static void main(String[] args) { Theatre screen1 = new Theatre(); Theatre screen2 = new Theatre(); Theatre screen3 = new Theatre(); screen1.movie_name = "Leo"; screen2.movie_name = "Vidaamuyarchi"; screen3.movie_name = "VDS Part 2"; screen1.show_time = 11.30f; screen2.show_time = 11.00f; screen3.show_time = 11.20f; System.out.println(screen1.movie_name); } } 2.school `public class School { int no_students; //int float timing; //float String name; //String boolean open; //boolean public static void main(String[] args) { } } 3.Bank `public class Bank { int amount; //int float interest_rate; //float String name; //String boolean open; //boolean public static void main() { Bank bank = new Bank(); } } Reference link https://www.w3schools.com/java/java_classes.asp
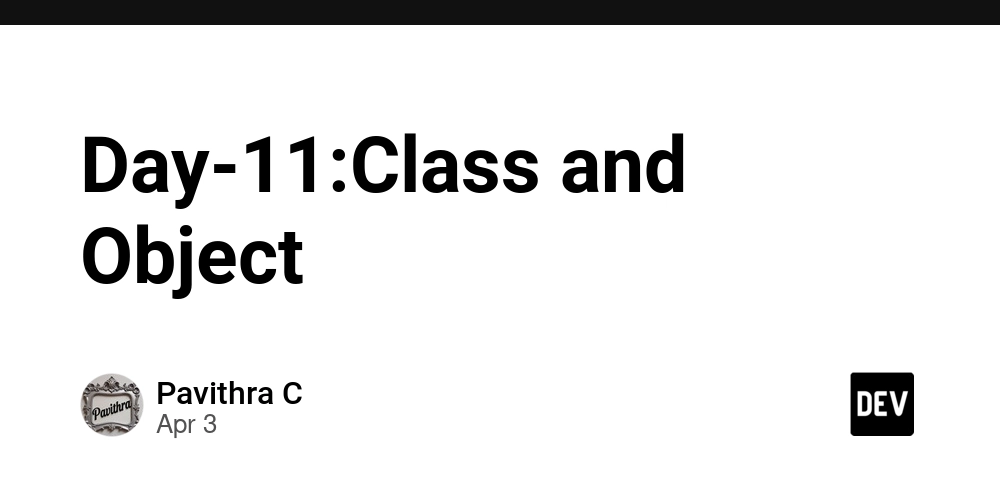
class
A Class is like an object constructor, or a "blueprint" for creating objects.
Create a class named "Main" with a variable x:
public class Main {
int x = 5;
}
Object
In Java, an object is created from a class. We have already created the class named Main, so now we can use this to create objects.
To create an object of Main, specify the class name, followed by the object name, and use the keyword new
Object: Combination of States and Behaviours
Example
Create an object called "myObj" and print the value of x:
public class Main {
int x = 5;
public static void main(String[] args) {
Main myObj = new Main();
System.out.println(myObj.x);
}
}
Multiple object
You can create multiple objects of one class:
public class Main {
int x = 5;
public static void main(String[] args) {
Main myObj1 = new Main(); // Object 1
Main myObj2 = new Main(); // Object 2
System.out.println(myObj1.x);
System.out.println(myObj2.x);
}
}
Multiple class
You can also create an object of a class and access it in another class. This is often used for better organization of classes (one class has all the attributes and methods, while the other class holds the main() method (code to be executed)).
Remember that the name of the java file should match the class name. In this example, we have created two files in the same directory/folder:
1.Main.java
2.Second.java
Main.java
public class Main {
int x = 5;
}
Second.java
class Second {
public static void main(String[] args) {
Main myObj = new Main();
System.out.println(myObj.x);
}
}
Naming conventions
1) Class name should start with capital Letter
Eg. Calculator
my first java program
2) Should Follow Camel Case
MyFirstJavaProgram, My_First_Java_Program
3) Should not start with Numbers
Eg. 2Calculator --> Calculator2
4) No Special Characters except _, $
5) MEANINGFUL NAME
6) Class Names should be Nouns, should not be Verbs.
Eg. Browser, Browse is not correct.
Naming Convention:
Class -> First letter CAPITAL
Interface
String is a class.
int, float, boolean - Not classes
Example
1.Theatre
public class Theatre
{
int ticket_fare; //int
float show_time; //float
String movie_name; //String
boolean on_screen; //boolean
public static void main(String[] args)
{
Theatre screen1 = new Theatre();
Theatre screen2 = new Theatre();
Theatre screen3 = new Theatre();
screen1.movie_name = "Leo";
screen2.movie_name = "Vidaamuyarchi";
screen3.movie_name = "VDS Part 2";
screen1.show_time = 11.30f;
screen2.show_time = 11.00f;
screen3.show_time = 11.20f;
System.out.println(screen1.movie_name);
}
}
2.school
`public class School {
int no_students; //int
float timing; //float
String name; //String
boolean open; //boolean
public static void main(String[] args) {
}
}
3.Bank
`public class Bank {
int amount; //int
float interest_rate; //float
String name; //String
boolean open; //boolean
public static void main() {
Bank bank = new Bank();
}
}
Reference link
https://www.w3schools.com/java/java_classes.asp