Data and Variables: The Core of Programming
Module 2: Data and Variables: The Core of Programming Introduction Data and variables form the backbone of any programming language. In this module, you'll learn how to store, manipulate, and work with various types of data to build dynamic, responsive applications. Lesson 1: Understanding Data Types: Numbers, Text, Booleans & More Concept: Data types are classifications of data that tell the computer how the data will be used. Understanding different data types helps in designing efficient and bug-free programs. Primary Data Types: Numbers: Integers (int) and floating-point numbers (float) for mathematical operations. Text (Strings): Sequences of characters for storing and manipulating text. Booleans: True/False values for decision-making and logical operations. Complex Types: Lists, dictionaries, and tuples for advanced data structures. Code Example: # Different data types age = 25 # Integer price = 19.99 # Float name = "Alice" # String is_member = True # Boolean Pro Tip: Always choose the most suitable data type to optimize memory and performance. Lesson 2: Variables and Constants: Storing and Managing Data Concept: Variables are containers that store data values which can change, whereas constants are fixed values that do not change during execution. Example Code: # Variable example age = 25 print(age) # Re-assigning variable age = age + 1 print("New age:", age) # Constant example PI = 3.14159 RADIUS = 5 area = PI * RADIUS * RADIUS print("Area:", area) Best Practices: Use descriptive variable names. Use uppercase for constants to differentiate them from variables. Minimize the use of global variables for better program structure. Pro Tip: Initialize variables with meaningful defaults to avoid unexpected errors. Lesson 3: Operators and Expressions: Performing Calculations and Comparisons Concept: Operators are symbols that perform operations on variables and values. Expressions are combinations of values and operators that are evaluated to produce a result. Types of Operators: Arithmetic Operators: +, -, *, /, % Comparison Operators: ==, !=, , = Logical Operators: and, or, not Example Code: x = 10 y = 5 # Arithmetic print(x + y) print(x * y) # Comparison print(x == y) print(x > y) # Logical is_adult = True has_ticket = False print(is_adult and has_ticket) Pro Tip: Keep expressions simple for better readability and easier debugging. Lesson 4: Type Conversion: Working with Mixed Data Concept: Type conversion allows you to convert data from one type to another, essential for operations involving mixed data types. Types of Conversion: Implicit Conversion: Automatically handled by Python. Explicit Conversion: Manual conversion using functions like int(), float(), and str(). Example Code: # Implicit conversion num = 10 flt = 2.5 result = num + flt print(result) # Explicit conversion num_str = "100" num_int = int(num_str) print(num_int + 50) Common Pitfalls: Be cautious with string-to-number conversions to avoid ValueError. Handle exceptions gracefully when type conversion might fail. Pro Tip: Use helper functions to validate data types before performing conversions. Conclusion Understanding data types, variables, constants, operators, and type conversions is fundamental to writing effective and efficient programs. These concepts ensure that data is correctly processed and stored, forming the foundation for complex logic. Key Takeaways: Choose the right data types for optimized memory and performance. Use variables and constants appropriately for better readability and maintenance. Master operators and expressions to simplify calculations and logic. Apply type conversion carefully to prevent runtime errors. What's Next? In the next module, we'll explore how to control the flow of your program using conditions and loops to automate tasks and make dynamic decisions. Visit us at Testamplify | X | Instagram | LinkedIn
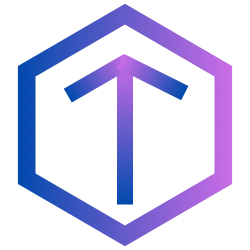
Module 2: Data and Variables: The Core of Programming
Introduction
Data and variables form the backbone of any programming language. In this module, you'll learn how to store, manipulate, and work with various types of data to build dynamic, responsive applications.
Lesson 1: Understanding Data Types: Numbers, Text, Booleans & More
Concept:
Data types are classifications of data that tell the computer how the data will be used. Understanding different data types helps in designing efficient and bug-free programs.
Primary Data Types:
-
Numbers: Integers (
int
) and floating-point numbers (float
) for mathematical operations. - Text (Strings): Sequences of characters for storing and manipulating text.
- Booleans: True/False values for decision-making and logical operations.
- Complex Types: Lists, dictionaries, and tuples for advanced data structures.
Code Example:
# Different data types
age = 25 # Integer
price = 19.99 # Float
name = "Alice" # String
is_member = True # Boolean
Pro Tip: Always choose the most suitable data type to optimize memory and performance.
Lesson 2: Variables and Constants: Storing and Managing Data
Concept:
Variables are containers that store data values which can change, whereas constants are fixed values that do not change during execution.
Example Code:
# Variable example
age = 25
print(age)
# Re-assigning variable
age = age + 1
print("New age:", age)
# Constant example
PI = 3.14159
RADIUS = 5
area = PI * RADIUS * RADIUS
print("Area:", area)
Best Practices:
- Use descriptive variable names.
- Use uppercase for constants to differentiate them from variables.
- Minimize the use of global variables for better program structure.
Pro Tip: Initialize variables with meaningful defaults to avoid unexpected errors.
Lesson 3: Operators and Expressions: Performing Calculations and Comparisons
Concept:
Operators are symbols that perform operations on variables and values. Expressions are combinations of values and operators that are evaluated to produce a result.
Types of Operators:
-
Arithmetic Operators:
+
,-
,*
,/
,%
-
Comparison Operators:
==
,!=
,<
,>
,<=
,>=
-
Logical Operators:
and
,or
,not
Example Code:
x = 10
y = 5
# Arithmetic
print(x + y)
print(x * y)
# Comparison
print(x == y)
print(x > y)
# Logical
is_adult = True
has_ticket = False
print(is_adult and has_ticket)
Pro Tip: Keep expressions simple for better readability and easier debugging.
Lesson 4: Type Conversion: Working with Mixed Data
Concept:
Type conversion allows you to convert data from one type to another, essential for operations involving mixed data types.
Types of Conversion:
- Implicit Conversion: Automatically handled by Python.
-
Explicit Conversion: Manual conversion using functions like
int()
,float()
, andstr()
.
Example Code:
# Implicit conversion
num = 10
flt = 2.5
result = num + flt
print(result)
# Explicit conversion
num_str = "100"
num_int = int(num_str)
print(num_int + 50)
Common Pitfalls:
- Be cautious with string-to-number conversions to avoid
ValueError
. - Handle exceptions gracefully when type conversion might fail.
Pro Tip: Use helper functions to validate data types before performing conversions.
Conclusion
Understanding data types, variables, constants, operators, and type conversions is fundamental to writing effective and efficient programs. These concepts ensure that data is correctly processed and stored, forming the foundation for complex logic.
Key Takeaways:
- Choose the right data types for optimized memory and performance.
- Use variables and constants appropriately for better readability and maintenance.
- Master operators and expressions to simplify calculations and logic.
- Apply type conversion carefully to prevent runtime errors.
What's Next?
In the next module, we'll explore how to control the flow of your program using conditions and loops to automate tasks and make dynamic decisions.
Visit us at Testamplify | X | Instagram | LinkedIn