Cypress Automation - Verify if your SSL Certificates are Valid
Recently I had to create a PoC where in the project's automation testing I needed to add a test that checks the validity of a SSL Certificate for a specific API. I was not sure how this could be achieved and didn't find useful tutorials. I had to dig deeper and test a few external libs/modules until I found the right one. Let me show you what I did... Install SSL-Checker SSL-Checker is a small Node.js module that checks the validity of an SSL certificate. We can use this with Cypress in order to create small tests.... npm install ssl-checker Creating a Cypress task The module above, being a Node.js one, will not run in a browser, so we need to address this. Cypress provides an event "task" which can be used in conjunction with the cy.task() command in order to execute arbitrary Node.js code. The first step is to build a new task that will run the sslChecker method and return the response, which we'll use in our test for an assertion: import { defineConfig } from 'cypress'; // Import the SSL-Check module import sslCheck from 'ssl-checker'; export default defineConfig({ e2e: { // Other configurations can go here setupNodeEvents (on) { // Create the new task on('task', { // Task name will be "getSSLValidity" getSSLValidity: (host) => { // The "host" param will be the URL we need to verify return sslCheck(host) } }) }, }, }) *In order to be able to use 'import' for our module, we need to add this line to package.json : "type": "module" Creating the test Our Cypress test would be fairly simple. Using the above task we get the SSL information and use it in a simple assertion. describe('BlogDeIT.ro Tests', () => { it('Should be more than 30 days before SSL Certificate expires', async () => { // Using the task "getSSLValidity" on "blogdeit.ro" cy.task('getSSLValidity', 'blogdeit.ro').then((cert) => { // Check if the SSL Certificate is valid for more than 30 days expect(cert.daysRemaining).to.be.greaterThan(30); }); }); }); The video version of this tutorial can be found here, but please note that it is in my native language, Romanian.
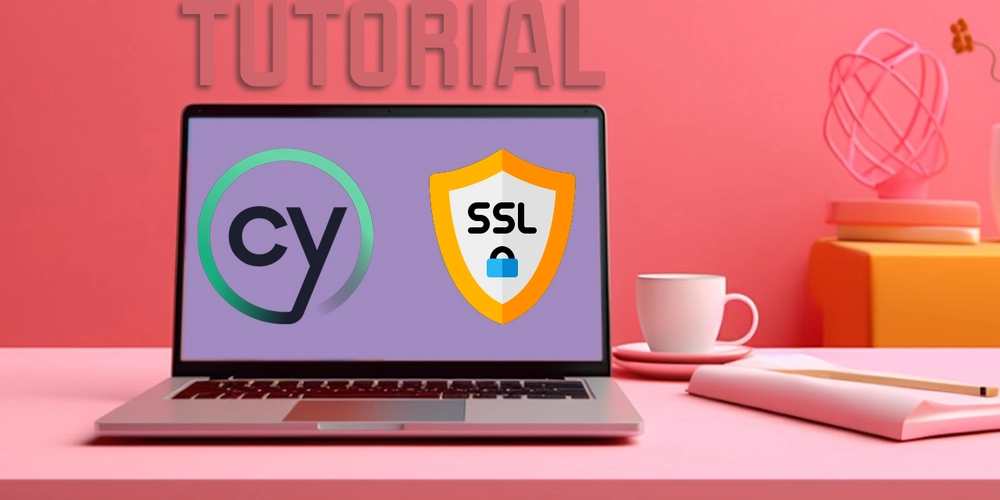
Recently I had to create a PoC where in the project's automation testing I needed to add a test that checks the validity of a SSL Certificate for a specific API. I was not sure how this could be achieved and didn't find useful tutorials. I had to dig deeper and test a few external libs/modules until I found the right one. Let me show you what I did...
Install SSL-Checker
SSL-Checker is a small Node.js module that checks the validity of an SSL certificate. We can use this with Cypress in order to create small tests....
npm install ssl-checker
Creating a Cypress task
The module above, being a Node.js one, will not run in a browser, so we need to address this. Cypress provides an event "task" which can be used in conjunction with the cy.task() command in order to execute arbitrary Node.js code.
The first step is to build a new task that will run the sslChecker method and return the response, which we'll use in our test for an assertion:
import { defineConfig } from 'cypress';
// Import the SSL-Check module
import sslCheck from 'ssl-checker';
export default defineConfig({
e2e: {
// Other configurations can go here
setupNodeEvents (on) {
// Create the new task
on('task', {
// Task name will be "getSSLValidity"
getSSLValidity: (host) => {
// The "host" param will be the URL we need to verify
return sslCheck(host)
}
})
},
},
})
*In order to be able to use 'import' for our module, we need to add this line to package.json : "type": "module"
Creating the test
Our Cypress test would be fairly simple. Using the above task we get the SSL information and use it in a simple assertion.
describe('BlogDeIT.ro Tests', () => {
it('Should be more than 30 days before SSL Certificate expires', async () => {
// Using the task "getSSLValidity" on "blogdeit.ro"
cy.task('getSSLValidity', 'blogdeit.ro').then((cert) => {
// Check if the SSL Certificate is valid for more than 30 days
expect(cert.daysRemaining).to.be.greaterThan(30);
});
});
});
The video version of this tutorial can be found here, but please note that it is in my native language, Romanian.