Create a Tool to Preview Google Suggestions Worldwide
Google's Autocomplete (or Google Suggest) provides real-time search query suggestions as users type. While Google doesn't offer a public, documented API for this, the underlying endpoint can be accessed. In this article, you'll learn how to build a tool that previews these suggestions for different locales (language/country combinations). This project is great for understanding frontend API calls and tackling common issues like CORS errors. Google Autocomplete Checker demo Link to the demo: https://autocomplete.corsfix.com Understanding the CORS Challenge When you try to call the Google Suggest endpoint directly from your frontend (e.g., fetching suggestions like https://suggestqueries.google.com/complete/search?output=toolbar&hl=en&gl=us&q=yourquery), you'll likely encounter a CORS error. This happens because the Google endpoint doesn’t return the necessary CORS headers (Access-Control-Allow-Origin) for direct browser access from different origins. Your browser, enforcing the Same-Origin Policy, blocks the response. Access to fetch at 'https://suggestqueries.google.com/...' from origin 'http://localhost:3000' has been blocked by CORS policy: No 'Access-Control-Allow-Origin' header is present on the requested resource. CORS Error when calling Google Suggest endpoint directly The Workaround: Using a CORS Proxy To bypass this limitation without needing your own backend server, you can use a CORS proxy. A CORS proxy acts as an intermediary: it fetches the data from the target API (Google Suggest, in this case) on your behalf and forwards the response back to your frontend, adding the required CORS headers. This allows your browser to access the data smoothly. fetch("https://proxy.corsfix.com/?"); Using CORS proxy to fetch Google Suggest data Setting Up Your Project For this project, we'll use Next.js. While the demo uses a standard Next.js setup, you could adapt it for static export if needed, allowing deployment on various static hosting services (like GitHub Pages, Cloudflare Pages, Netlify, or Firebase Hosting) without a dedicated Node.js server. What Your App Needs to Do Fetch Google Autocomplete Data: Retrieve search suggestions from the Google Suggest endpoint via a CORS proxy for specified locales and store them in your component state. Create Locale Selectors: Allow users to add, remove, and configure multiple locales (language and country pairs). Display Suggestions: Render the list of suggestions grouped by locale. Handle User Input: Capture the user's search query. Now let’s dive into each of these steps. Building the App 1. Fetching the Google Autocomplete API Directly calling the Google Suggest endpoint from your frontend causes CORS issues. We use a CORS proxy to fetch the data. You'll need the user's query and the selected locale (language hl and country gl) parameters. The response is in XML format, so you'll need to parse it. // Example fetch using a CORS proxy for 'en-us' locale async function fetchSuggestions(query, locale) { const url = new URL("https://suggestqueries.google.com/complete/search"); url.searchParams.set("output", "toolbar"); url.searchParams.set("hl", locale.language); // e.g., 'en' url.searchParams.set("gl", locale.country); // e.g., 'us' url.searchParams.set("q", query); try { const response = await fetch( `https://proxy.corsfix.com/?${url.toString()}` ); if (!response.ok) { throw new Error(`HTTP error! status: ${response.status}`); } const xmlText = await response.text(); const parser = new DOMParser(); const xmlDoc = parser.parseFromString(xmlText, "text/xml"); const suggestionsNodes = xmlDoc.querySelectorAll( "CompleteSuggestion > suggestion" ); const suggestions = Array.from(suggestionsNodes).map((node) => ({ text: node.getAttribute("data") || "", })); // Store the suggestions in state console.log(suggestions); return suggestions; } catch (err) { console.error("Error fetching Google suggestions:", err); return []; } } 2. Creating Locale Selectors Google Suggest results vary by language (hl) and country (gl). You need UI elements to let users select these. You can store the selected locales in component state. // Simplified example of locale data and state const languages = [{ name: "English", code: "en" } /* ... */]; const countries = [{ name: "United States", code: "us" } /* ... */]; // In your component state const [locales, setLocales] = useState([ { country: "us", language: "en", name: "United States" }, ]); // Functions to add, remove, or update locales in the state array const handleAddLocale = () => { /* ... add new locale */ }; const handleRemoveLocale = (index) => { /* ... remove locale at index */ }; const handleLocaleChange = (index, updatedLocale) => { /* ... update locale at index */ }; You can use dropdowns, combo boxes (lik
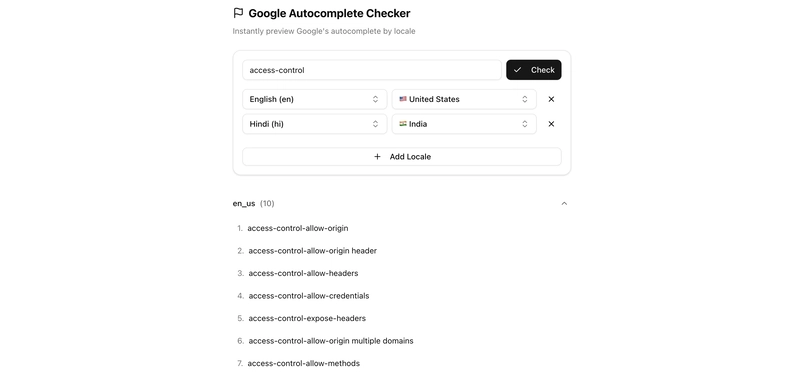
Google's Autocomplete (or Google Suggest) provides real-time search query suggestions as users type. While Google doesn't offer a public, documented API for this, the underlying endpoint can be accessed. In this article, you'll learn how to build a tool that previews these suggestions for different locales (language/country combinations). This project is great for understanding frontend API calls and tackling common issues like CORS errors.
Google Autocomplete Checker demo
Link to the demo: https://autocomplete.corsfix.com
Understanding the CORS Challenge
When you try to call the Google Suggest endpoint directly from your frontend (e.g., fetching suggestions like https://suggestqueries.google.com/complete/search?output=toolbar&hl=en&gl=us&q=yourquery
), you'll likely encounter a CORS error. This happens because the Google endpoint doesn’t return the necessary CORS headers (Access-Control-Allow-Origin
) for direct browser access from different origins. Your browser, enforcing the Same-Origin Policy, blocks the response.
Access to fetch at 'https://suggestqueries.google.com/...' from origin 'http://localhost:3000' has been blocked by CORS policy: No 'Access-Control-Allow-Origin' header is present on the requested resource.
CORS Error when calling Google Suggest endpoint directly
The Workaround: Using a CORS Proxy
To bypass this limitation without needing your own backend server, you can use a CORS proxy. A CORS proxy acts as an intermediary: it fetches the data from the target API (Google Suggest, in this case) on your behalf and forwards the response back to your frontend, adding the required CORS headers. This allows your browser to access the data smoothly.
fetch("https://proxy.corsfix.com/? ");
Using CORS proxy to fetch Google Suggest data
Setting Up Your Project
For this project, we'll use Next.js. While the demo uses a standard Next.js setup, you could adapt it for static export if needed, allowing deployment on various static hosting services (like GitHub Pages, Cloudflare Pages, Netlify, or Firebase Hosting) without a dedicated Node.js server.
What Your App Needs to Do
- Fetch Google Autocomplete Data: Retrieve search suggestions from the Google Suggest endpoint via a CORS proxy for specified locales and store them in your component state.
- Create Locale Selectors: Allow users to add, remove, and configure multiple locales (language and country pairs).
- Display Suggestions: Render the list of suggestions grouped by locale.
- Handle User Input: Capture the user's search query.
Now let’s dive into each of these steps.
Building the App
1. Fetching the Google Autocomplete API
Directly calling the Google Suggest endpoint from your frontend causes CORS issues. We use a CORS proxy to fetch the data. You'll need the user's query and the selected locale (language hl
and country gl
) parameters. The response is in XML format, so you'll need to parse it.
// Example fetch using a CORS proxy for 'en-us' locale
async function fetchSuggestions(query, locale) {
const url = new URL("https://suggestqueries.google.com/complete/search");
url.searchParams.set("output", "toolbar");
url.searchParams.set("hl", locale.language); // e.g., 'en'
url.searchParams.set("gl", locale.country); // e.g., 'us'
url.searchParams.set("q", query);
try {
const response = await fetch(
`https://proxy.corsfix.com/?${url.toString()}`
);
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
const xmlText = await response.text();
const parser = new DOMParser();
const xmlDoc = parser.parseFromString(xmlText, "text/xml");
const suggestionsNodes = xmlDoc.querySelectorAll(
"CompleteSuggestion > suggestion"
);
const suggestions = Array.from(suggestionsNodes).map((node) => ({
text: node.getAttribute("data") || "",
}));
// Store the suggestions in state
console.log(suggestions);
return suggestions;
} catch (err) {
console.error("Error fetching Google suggestions:", err);
return [];
}
}
2. Creating Locale Selectors
Google Suggest results vary by language (hl
) and country (gl
). You need UI elements to let users select these. You can store the selected locales in component state.
// Simplified example of locale data and state
const languages = [{ name: "English", code: "en" } /* ... */];
const countries = [{ name: "United States", code: "us" } /* ... */];
// In your component state
const [locales, setLocales] = useState([
{ country: "us", language: "en", name: "United States" },
]);
// Functions to add, remove, or update locales in the state array
const handleAddLocale = () => {
/* ... add new locale */
};
const handleRemoveLocale = (index) => {
/* ... remove locale at index */
};
const handleLocaleChange = (index, updatedLocale) => {
/* ... update locale at index */
};
You can use dropdowns, combo boxes (like the Popover
and Command
components from shadcn/ui
used in the demo), or other inputs to manage the locales
state array.
3. Displaying the Suggestion Data
Once you have the suggestion data (grouped by locale key, e.g., en_us
) in your component's state, you can map over it to render the results. Using an Accordion component helps organize results for multiple locales.
// Simplified JSX structure for displaying suggestions by locale
{
isLoading ? (
<p>Loading...p>
) : error ? (
<p>Error: {error}p>
) : (
<Accordion type="multiple">
{Object.entries(results).map(([localeKey, suggestions]) => (
<AccordionItem key={localeKey} value={localeKey}>
<AccordionTrigger>
{localeKey} ({suggestions.length})
AccordionTrigger>
<AccordionContent>
{suggestions.length > 0 ? (
<ul>
{suggestions.map((suggestion, index) => (
<li key={index}>
<span>{index + 1}.span> {suggestion.text}
{/* Optional: Link to Google search */}
<a
href={`https://www.google.com/search?q=${encodeURIComponent(
suggestion.text
)}&hl=${localeKey.split("_")[0]}&gl=${
localeKey.split("_")[1]
}`}
target="_blank"
>
Search
a>
li>
))}
ul>
) : (
<p>No suggestions foundp>
)}
AccordionContent>
AccordionItem>
))}
Accordion>
);
}
This structure displays suggestions grouped by locale, showing the rank and text, with an optional link to perform the search on Google.
Conclusion
You’ve now built a Google Autocomplete Checker tool that uses the unofficial Google Suggest endpoint and effectively handles the CORS errors typically encountered. You learned how to overcome these issues using a CORS proxy, implement locale selection, fetch and parse XML data, and display the results clearly.
If you need a reliable CORS proxy for your frontend projects to access third-party APIs without backend hassle, give Corsfix a try! It's free to get started.
Happy coding!
Demo: https://autocomplete.corsfix.com
Code: https://github.com/corsfix/autocomplete-checker