Building an AI-Powered 'Erase and Replace' Image Tool with Next.js and React
Building an AI-Powered 'Erase and Replace' Image Tool with Next.js and React Ever wanted to remove an unwanted object from a photo or replace it entirely with something else? With advancements in AI, this is now possible. In this tutorial, we'll explore how to build an AI erase and replace image editing tool using Next.js and React. We'll leverage AI services to manipulate images and integrate them into a web application. Understanding AI-Powered Image Editing AI-powered image editing tools use advanced machine learning algorithms to understand image content and manipulate it intelligently. One of these functionalities is erase and replace, where users can remove objects from an image and replace them with generated content that blends seamlessly with the surroundings. Technologies We'll Use Before we dive in, let's look at the technologies we'll be using: Next.js: A React framework for server-rendered applications and static websites. It provides features like file-based routing, server-side rendering, and easy API creation. React: A JavaScript library for building user interfaces. We'll use it to create our frontend components. Webpack: Although Next.js handles bundling internally with Webpack, understanding it helps in customizing the build process if needed. We'll also integrate with an AI service for image manipulation. For demonstration purposes, we'll use a mock API, but in a production app, you might use services like TensorFlow.js, OpenAI's API, or other AI-as-a-Service platforms. Setting Up the Project First, set up a new Next.js project: bash npx create-next-app ai-erase-and-replace Navigate to the project directory: bash cd ai-erase-and-replace Start the development server: bash npm run dev You should now have a basic Next.js app running at http://localhost:3000. Implementing the AI Erase and Replace Functionality We'll build a page where users can upload an image, select an area to erase, provide a description of what they want to replace it with, and see the AI-manipulated result. Step 1: Creating the Upload Component Let's create a component that allows users to upload images: x // components/ImageUpload.js import { useState } from 'react'; export default function ImageUpload({ onUpload }) { const [selectedImage, setSelectedImage] = useState(null); const handleImageChange = (e) => { if (e.target.files && e.target.files[0]) { setSelectedImage(URL.createObjectURL(e.target.files[0])); onUpload(e.target.files[0]); } }; return (
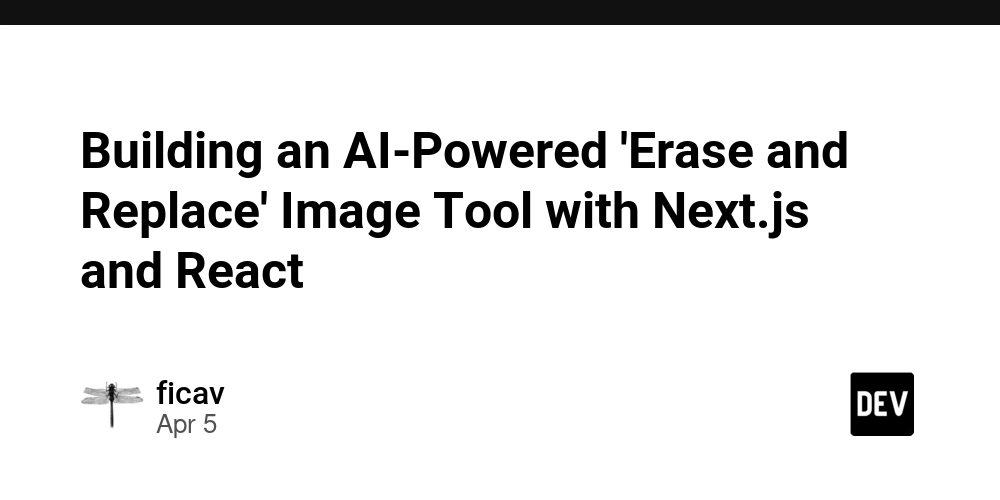
Building an AI-Powered 'Erase and Replace' Image Tool with Next.js and React
Ever wanted to remove an unwanted object from a photo or replace it entirely with something else? With advancements in AI, this is now possible. In this tutorial, we'll explore how to build an AI erase and replace image editing tool using Next.js and React. We'll leverage AI services to manipulate images and integrate them into a web application.
Understanding AI-Powered Image Editing
AI-powered image editing tools use advanced machine learning algorithms to understand image content and manipulate it intelligently. One of these functionalities is erase and replace, where users can remove objects from an image and replace them with generated content that blends seamlessly with the surroundings.
Technologies We'll Use
Before we dive in, let's look at the technologies we'll be using:
Next.js: A React framework for server-rendered applications and static websites. It provides features like file-based routing, server-side rendering, and easy API creation.
React: A JavaScript library for building user interfaces. We'll use it to create our frontend components.
Webpack: Although Next.js handles bundling internally with Webpack, understanding it helps in customizing the build process if needed.
We'll also integrate with an AI service for image manipulation. For demonstration purposes, we'll use a mock API, but in a production app, you might use services like TensorFlow.js, OpenAI's API, or other AI-as-a-Service platforms.
Setting Up the Project
First, set up a new Next.js project:
bash
npx create-next-app ai-erase-and-replace
Navigate to the project directory:
bash
cd ai-erase-and-replace
Start the development server:
bash
npm run dev
You should now have a basic Next.js app running at http://localhost:3000
.
Implementing the AI Erase and Replace Functionality
We'll build a page where users can upload an image, select an area to erase, provide a description of what they want to replace it with, and see the AI-manipulated result.
Step 1: Creating the Upload Component
Let's create a component that allows users to upload images:
x
// components/ImageUpload.js
import { useState } from 'react';
export default function ImageUpload({ onUpload }) {
const [selectedImage, setSelectedImage] = useState(null);
const handleImageChange = (e) => {
if (e.target.files && e.target.files[0]) {
setSelectedImage(URL.createObjectURL(e.target.files[0]));
onUpload(e.target.files[0]);
}
};
return (