Building a Simple Terminal-Based File Manager in Bash
A while ago, I found myself juggling between ls, cd, rm, and mv commands just to move and manage files across different directories. It felt inefficient—why not have a lightweight file manager right inside the terminal? If you’ve ever wished for a simpler way to navigate and manipulate files without leaving the terminal, this guide will show you how to build a basic file manager in Bash—one that lets you browse, create, delete, and move files interactively. Understanding the Core of a Terminal File Manager A terminal-based file manager is essentially a Bash script that allows users to navigate directories and manage files using menus and keyboard inputs. What It Should Do ✅ Display a list of files and directories ✅ Let users navigate between folders ✅ Provide options to delete, move, or rename files ✅ Offer a simple interface using the terminal This can be accomplished using a combination of Bash commands like ls, cd, rm, and mv, along with interactive menus using select or read. Step 1: Listing Files and Directories The first step is displaying the current directory contents in a structured way. Instead of using a plain ls, let’s format the output to distinguish between files and directories. for item in *; do if [ -d "$item" ]; then echo "[DIR] $item" else echo "[FILE] $item" fi done
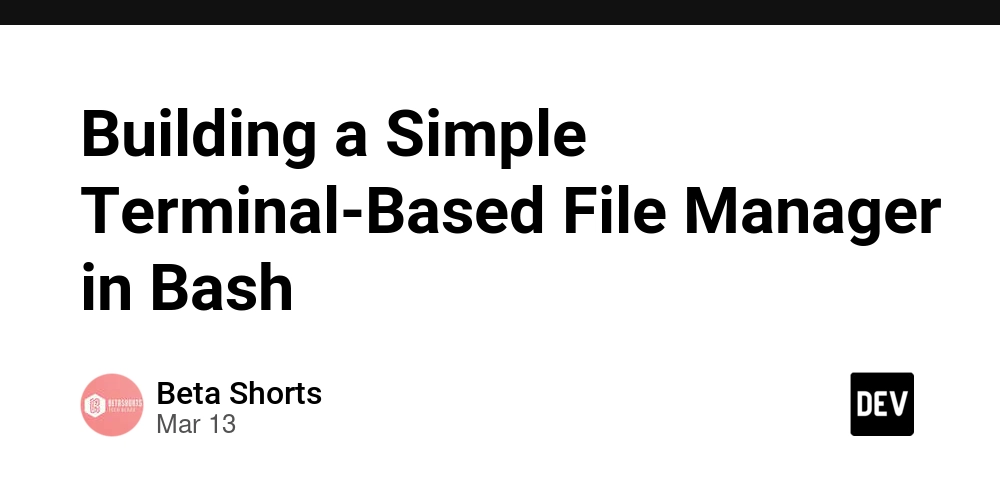
A while ago, I found myself juggling between ls
, cd
, rm
, and mv
commands just to move and manage files across different directories. It felt inefficient—why not have a lightweight file manager right inside the terminal?
If you’ve ever wished for a simpler way to navigate and manipulate files without leaving the terminal, this guide will show you how to build a basic file manager in Bash—one that lets you browse, create, delete, and move files interactively.
Understanding the Core of a Terminal File Manager
A terminal-based file manager is essentially a Bash script that allows users to navigate directories and manage files using menus and keyboard inputs.
What It Should Do
✅ Display a list of files and directories
✅ Let users navigate between folders
✅ Provide options to delete, move, or rename files
✅ Offer a simple interface using the terminal
This can be accomplished using a combination of Bash commands like ls
, cd
, rm
, and mv
, along with interactive menus using select
or read
.
Step 1: Listing Files and Directories
The first step is displaying the current directory contents in a structured way. Instead of using a plain ls
, let’s format the output to distinguish between files and directories.
for item in *; do
if [ -d "$item" ]; then
echo "[DIR] $item"
else
echo "[FILE] $item"
fi
done