Se o Ownership é o coração de Rust, o Borrowing é o cérebro que permite trabalhar com dados sem perder a segurança. O que é Borrowing? Em Rust, emprestar um valor significa usá-lo temporariamente sem tomar a sua posse. Isso permite que múltiplas partes do código acessem os mesmos dados, mas sem risco de acessos inválidos ou modificações inesperadas. fn main() { let nome = String::from("Rust"); // Empresta 'nome' para a função, sem transferir a posse saudacao(&nome); println!("Olá, {}", nome); // 'nome' ainda é válido aqui } fn saudacao(n: &String) { println!("Olá, {}!", n); } Tipos de Empréstimos &T (Empréstimo Imutável): Permite ler o valor, mas não modificá-lo. &mut T (Empréstimo Mutável): Permite ler e modificar o valor, mas só pode existir um empréstimo mutável por vez. fn main() { let mut valor = 10; // Empréstimo imutável imprime(&valor); // Empréstimo mutável modifica(&mut valor); println!("Valor final: {}", valor); } fn imprime(v: &i32) { println!("Valor: {}", v); } fn modifica(v: &mut i32) { *v += 5; // Modifica o valor original } O que não se pode fazer? Não se pode ter empréstimos mutáveis e imutáveis ao mesmo tempo sobre o mesmo valor. Isso evita situações onde um valor é lido enquanto é modificado, o que poderia causar comportamentos imprevisíveis. Porque é que isto é importante? O sistema de Borrowing permite: Acesso seguro a dados sem cópias desnecessárias Controle total sobre quem pode ler ou modificar os dados Código mais eficiente e livre de erros como data races Rust obriga a pensar como os dados são usados — e embora pareça exigente no início, dá muito mais poder e confiança a longo prazo. If Ownership is the heart of Rust, Borrowing is the brain that allows you to work with data without losing safety. What is Borrowing? In Rust, borrowing a value means using it temporarily without taking ownership of it. This allows multiple parts of the code to access the same data, but without the risk of invalid accesses or unexpected modifications. fn main() { let nome = String::from("Rust"); // Borrow 'nome' to the function without transferring ownership saudacao(&nome); println!("Hello, {}", nome); // 'nome' is still valid here } fn saudacao(n: &String) { println!("Hello, {}!", n); } Types of Borrowing &T (Immutable Borrow): Allows reading the value but not modifying it. &mut T (Mutable Borrow): Allows reading and modifying the value, but only one mutable borrow can exist at a time. fn main() { let mut valor = 10; // Immutable borrow imprime(&valor); // Mutable borrow modifica(&mut valor); println!("Final value: {}", valor); } fn imprime(v: &i32) { println!("Value: {}", v); } fn modifica(v: &mut i32) { *v += 5; // Modifies the original value } What can't you do? You cannot have mutable and immutable borrows at the same time over the same value. This prevents situations where a value is read while being modified, which could cause unpredictable behavior. Why is this important? The borrowing system allows: Safe access to data without unnecessary copies Full control over who can read or modify the data More efficient and error-free code, avoiding issues like data races Rust forces you to think about how data is used — and although it may seem demanding at first, it gives you much more power and confidence in the long run.
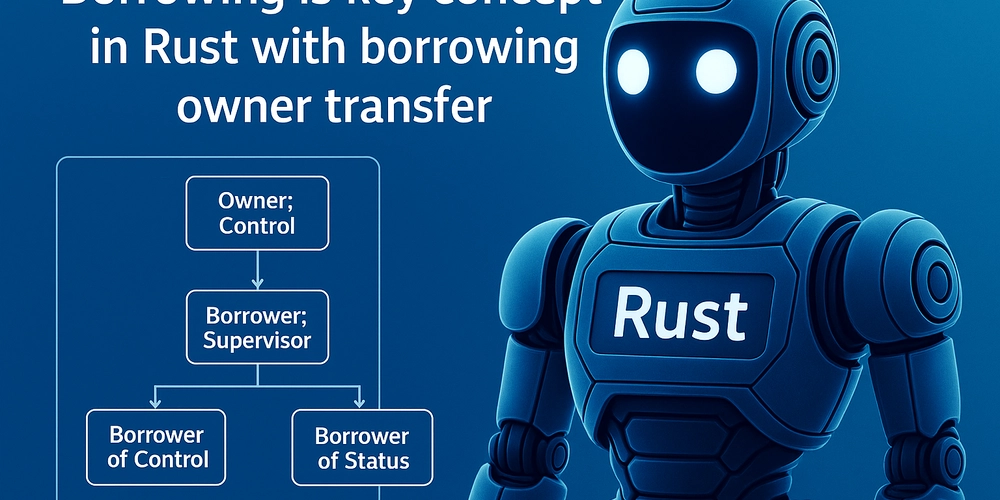
Se o Ownership é o coração de Rust, o Borrowing é o cérebro que permite trabalhar com dados sem perder a segurança.
O que é Borrowing?
Em Rust, emprestar um valor significa usá-lo temporariamente sem tomar a sua posse. Isso permite que múltiplas partes do código acessem os mesmos dados, mas sem risco de acessos inválidos ou modificações inesperadas.
fn main() {
let nome = String::from("Rust");
// Empresta 'nome' para a função, sem transferir a posse
saudacao(&nome);
println!("Olá, {}", nome); // 'nome' ainda é válido aqui
}
fn saudacao(n: &String) {
println!("Olá, {}!", n);
}
Tipos de Empréstimos
- &T (Empréstimo Imutável): Permite ler o valor, mas não modificá-lo.
- &mut T (Empréstimo Mutável): Permite ler e modificar o valor, mas só pode existir um empréstimo mutável por vez.
fn main() {
let mut valor = 10;
// Empréstimo imutável
imprime(&valor);
// Empréstimo mutável
modifica(&mut valor);
println!("Valor final: {}", valor);
}
fn imprime(v: &i32) {
println!("Valor: {}", v);
}
fn modifica(v: &mut i32) {
*v += 5; // Modifica o valor original
}
O que não se pode fazer?
Não se pode ter empréstimos mutáveis e imutáveis ao mesmo tempo sobre o mesmo valor. Isso evita situações onde um valor é lido enquanto é modificado, o que poderia causar comportamentos imprevisíveis.
Porque é que isto é importante?
O sistema de Borrowing permite:
- Acesso seguro a dados sem cópias desnecessárias
- Controle total sobre quem pode ler ou modificar os dados
- Código mais eficiente e livre de erros como data races
Rust obriga a pensar como os dados são usados — e embora pareça exigente no início, dá muito mais poder e confiança a longo prazo.
If Ownership is the heart of Rust, Borrowing is the brain that allows you to work with data without losing safety.
What is Borrowing?
In Rust, borrowing a value means using it temporarily without taking ownership of it. This allows multiple parts of the code to access the same data, but without the risk of invalid accesses or unexpected modifications.
fn main() {
let nome = String::from("Rust");
// Borrow 'nome' to the function without transferring ownership
saudacao(&nome);
println!("Hello, {}", nome); // 'nome' is still valid here
}
fn saudacao(n: &String) {
println!("Hello, {}!", n);
}
Types of Borrowing
- &T (Immutable Borrow): Allows reading the value but not modifying it.
- &mut T (Mutable Borrow): Allows reading and modifying the value, but only one mutable borrow can exist at a time.
fn main() {
let mut valor = 10;
// Immutable borrow
imprime(&valor);
// Mutable borrow
modifica(&mut valor);
println!("Final value: {}", valor);
}
fn imprime(v: &i32) {
println!("Value: {}", v);
}
fn modifica(v: &mut i32) {
*v += 5; // Modifies the original value
}
What can't you do?
You cannot have mutable and immutable borrows at the same time over the same value. This prevents situations where a value is read while being modified, which could cause unpredictable behavior.
Why is this important?
The borrowing system allows:
- Safe access to data without unnecessary copies
- Full control over who can read or modify the data
- More efficient and error-free code, avoiding issues like data races
Rust forces you to think about how data is used — and although it may seem demanding at first, it gives you much more power and confidence in the long run.