Bloom Filters: A Comprehensive Guide for Web Developers
Bloom Filters are one of the most intriguing data structures that every web developer and software engineer should know about. They offer a space-efficient, probabilistic solution for membership testing—always a hot topic in scalability and performance engineering. In this guide, we'll dive deep into how Bloom Filters work, explore real-world applications, and provide code examples in both Python and JavaScript to help you integrate this technique into your projects. Table of Contents Introduction What is a Bloom Filter? How Do Bloom Filters Work? Advantages and Disadvantages Implementing a Bloom Filter Python Example JavaScript Example Real-World Applications Conclusion Further Resources Introduction In the realm of web development and software engineering, efficient data processing and storage are paramount. Bloom Filters are used when you need to quickly check if an element is present in a set, without the overhead of storing the entire dataset. Although Bloom Filters can return false positives, they never yield false negatives, making them ideal for applications where speed and space are critical. In this article, we'll learn how to build and implement your very own Bloom Filter, understand its inner workings, and examine its pros and cons. What is a Bloom Filter? A Bloom Filter is a probabilistic data structure that allows you to test whether an element is a member of a set. It is particularly useful when the dataset is so large that storing every element is impractical. Instead of storing the elements themselves, the Bloom Filter maintains a bit array and several independent hash functions. The basic concept is as follows: Adding an element: Each hash function is applied to the element, and the bits at the resulting positions in the array are set to 1. Querying membership: If all the bits at the hash function positions for an element are set to 1, the filter reports that the element may be in the set; if any of the bits is 0, the element is definitely not in the set. This wonderfully efficient approach makes Bloom Filters ideal for tasks like caching, spell-checking, and network security filters. The trade-off? A controlled risk of false positives. This means that while it might sometimes claim an element is in the set when it’s not, it will never mistakenly exclude an element that is present. How Do Bloom Filters Work? At the heart of a Bloom Filter lies the combination of a bit array and multiple hash functions: Bit Array: A fixed-size array initialized with all bits set to 0. Hash Functions: Independent functions that each map an input element to a different array index. Insertion Example: Suppose you want to add the string "developer". The hash functions might convert "developer" (with different seeds or variations) into indices like 5, 18, and 26. The Bloom Filter then sets the bits at these indices to 1. Membership Checking: To check if "developer" exists in the set, the same hash functions generate the indices 5, 18, and 26. If all these bits are 1, the element may be in the set; otherwise, it is definitely not present. This process highlights the trade-off inherent in Bloom Filters—efficiency at the cost of some accuracy (false positives). This makes them especially useful in scenarios where speed and memory usage trump perfect accuracy. Advantages and Disadvantages Advantages Space Efficiency: Requires significantly less memory compared to storing large datasets. Speed: Lookups and insertions are extremely fast. Simplicity: Easy to implement, even in performance-critical environments. Disadvantages False Positives: They can mistakenly indicate that an element is present. Inability to Delete: Standard Bloom Filters do not support deletion. (Counted Bloom Filters can overcome this with extra complexity.) Fixed Size: The size of the bit array must be determined in advance, and poor parameter tuning can impact performance. Implementing a Bloom Filter In this section, we’ll walk through the implementation of a simple Bloom Filter with code examples in both Python and JavaScript. These examples are designed to be clear and educational, so you can quickly see how to integrate Bloom Filters into your own projects. Python Example Below is a basic implementation of a Bloom Filter in Python. This example uses Python's built-in hash() function to simulate multiple hash functions. In production, you’d likely use more robust and independent hash functions. class BloomFilter: def __init__(self, size, hash_count): self.size = size self.hash_count = hash_count self.bit_array = [0] * size def add(self, item): for i in range(self.hash_count): # Create a unique hash by combining the item with the hash function index index = hash(f"{item}{i}") % self.size self.bit_array[inde
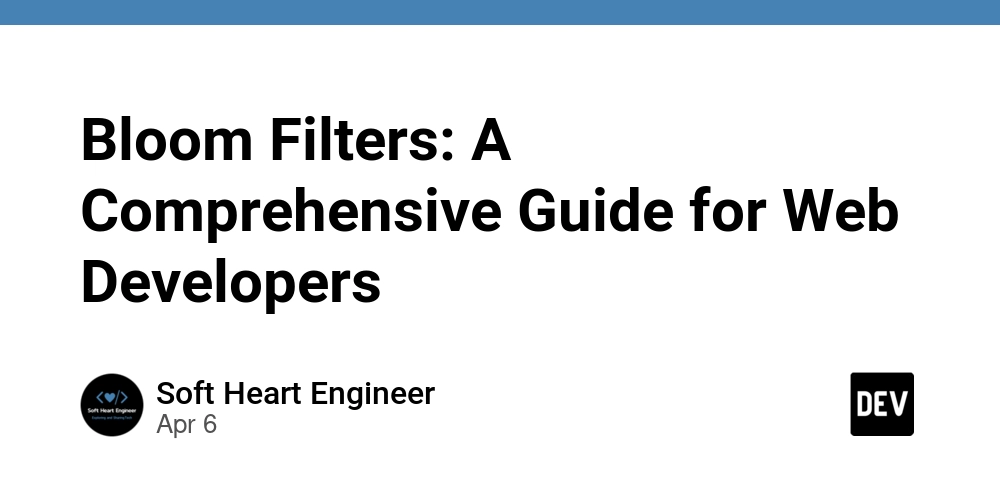
Bloom Filters are one of the most intriguing data structures that every web developer and software engineer should know about. They offer a space-efficient, probabilistic solution for membership testing—always a hot topic in scalability and performance engineering. In this guide, we'll dive deep into how Bloom Filters work, explore real-world applications, and provide code examples in both Python and JavaScript to help you integrate this technique into your projects.
Table of Contents
- Introduction
- What is a Bloom Filter?
- How Do Bloom Filters Work?
- Advantages and Disadvantages
-
Implementing a Bloom Filter
- Python Example
- JavaScript Example
- Real-World Applications
- Conclusion
- Further Resources
Introduction
In the realm of web development and software engineering, efficient data processing and storage are paramount. Bloom Filters are used when you need to quickly check if an element is present in a set, without the overhead of storing the entire dataset. Although Bloom Filters can return false positives, they never yield false negatives, making them ideal for applications where speed and space are critical. In this article, we'll learn how to build and implement your very own Bloom Filter, understand its inner workings, and examine its pros and cons.
What is a Bloom Filter?
A Bloom Filter is a probabilistic data structure that allows you to test whether an element is a member of a set. It is particularly useful when the dataset is so large that storing every element is impractical. Instead of storing the elements themselves, the Bloom Filter maintains a bit array and several independent hash functions.
The basic concept is as follows:
- Adding an element: Each hash function is applied to the element, and the bits at the resulting positions in the array are set to 1.
- Querying membership: If all the bits at the hash function positions for an element are set to 1, the filter reports that the element may be in the set; if any of the bits is 0, the element is definitely not in the set.
This wonderfully efficient approach makes Bloom Filters ideal for tasks like caching, spell-checking, and network security filters. The trade-off? A controlled risk of false positives. This means that while it might sometimes claim an element is in the set when it’s not, it will never mistakenly exclude an element that is present.
How Do Bloom Filters Work?
At the heart of a Bloom Filter lies the combination of a bit array and multiple hash functions:
- Bit Array: A fixed-size array initialized with all bits set to 0.
- Hash Functions: Independent functions that each map an input element to a different array index.
Insertion Example:
- Suppose you want to add the string
"developer"
. - The hash functions might convert
"developer"
(with different seeds or variations) into indices like5
,18
, and26
. - The Bloom Filter then sets the bits at these indices to 1.
Membership Checking:
- To check if
"developer"
exists in the set, the same hash functions generate the indices5
,18
, and26
. - If all these bits are 1, the element may be in the set; otherwise, it is definitely not present.
This process highlights the trade-off inherent in Bloom Filters—efficiency at the cost of some accuracy (false positives). This makes them especially useful in scenarios where speed and memory usage trump perfect accuracy.
Advantages and Disadvantages
Advantages
- Space Efficiency: Requires significantly less memory compared to storing large datasets.
- Speed: Lookups and insertions are extremely fast.
- Simplicity: Easy to implement, even in performance-critical environments.
Disadvantages
- False Positives: They can mistakenly indicate that an element is present.
- Inability to Delete: Standard Bloom Filters do not support deletion. (Counted Bloom Filters can overcome this with extra complexity.)
- Fixed Size: The size of the bit array must be determined in advance, and poor parameter tuning can impact performance.
Implementing a Bloom Filter
In this section, we’ll walk through the implementation of a simple Bloom Filter with code examples in both Python and JavaScript. These examples are designed to be clear and educational, so you can quickly see how to integrate Bloom Filters into your own projects.
Python Example
Below is a basic implementation of a Bloom Filter in Python. This example uses Python's built-in hash()
function to simulate multiple hash functions. In production, you’d likely use more robust and independent hash functions.
class BloomFilter:
def __init__(self, size, hash_count):
self.size = size
self.hash_count = hash_count
self.bit_array = [0] * size
def add(self, item):
for i in range(self.hash_count):
# Create a unique hash by combining the item with the hash function index
index = hash(f"{item}{i}") % self.size
self.bit_array[index] = 1
def is_member(self, item):
for i in range(self.hash_count):
index = hash(f"{item}{i}") % self.size
if self.bit_array[index] == 0:
return False
return True
# Testing the Bloom Filter
if __name__ == "__main__":
bloom = BloomFilter(size=100, hash_count=3)
words = ["developer", "engineer", "python", "javascript"]
# Adding words to the bloom filter
for word in words:
bloom.add(word)
# Testing membership
test_words = ["developer", "golang", "python", "ruby"]
for word in test_words:
result = bloom.is_member(word)
print(f"'{word}' is in Bloom Filter: {result}")
Expected Output:
'developer' is in Bloom Filter: True
'golang' is in Bloom Filter: False
'python' is in Bloom Filter: True
'ruby' is in Bloom Filter: False
JavaScript Example
Here’s a JavaScript version of our Bloom Filter. This example uses a simple hash function for demonstration purposes. In real applications, you might use libraries or more sophisticated hash algorithms.
class BloomFilter {
constructor(size, hashCount) {
this.size = size;
this.hashCount = hashCount;
this.bitArray = new Array(size).fill(0);
}
// Simple hash function for demonstration (not for production use)
hash(item, seed) {
let hash = 0;
const str = item + seed;
for (let i = 0; i < str.length; i++) {
hash = (hash << 5) - hash + str.charCodeAt(i);
hash |= 0; // Convert to 32bit integer
}
return Math.abs(hash) % this.size;
}
add(item) {
for (let i = 0; i < this.hashCount; i++) {
let index = this.hash(item, i);
this.bitArray[index] = 1;
}
}
isMember(item) {
for (let i = 0; i < this.hashCount; i++) {
let index = this.hash(item, i);
if (this.bitArray[index] === 0) return false;
}
return true;
}
}
// Testing the Bloom Filter
const bloom = new BloomFilter(100, 3);
const words = ["developer", "engineer", "python", "javascript"];
// Adding words to the Bloom Filter
words.forEach(word => bloom.add(word));
// Testing membership
const testWords = ["developer", "golang", "python", "ruby"];
testWords.forEach(word => {
const result = bloom.isMember(word);
console.log(`'${word}' is in Bloom Filter: ${result}`);
});
Expected Output:
'developer' is in Bloom Filter: true
'golang' is in Bloom Filter: false
'python' is in Bloom Filter: true
'ruby' is in Bloom Filter: false
Both examples illustrate the core ideas behind Bloom Filters—using a bit array and multiple hash functions to manage membership queries efficiently.
Real World Applications
Bloom Filters are widely used in scenarios where performance and memory are critical:
Caching: Quickly checking if a cached item exists to reduce expensive disk lookups.
Databases: Helping to efficiently determine whether a record might exist in distributed systems.
Spam Filtering: Rapidly checking the legitimacy of emails.
Networking: Routing and packet filtering where false positives are tolerable.
By understanding and implementing Bloom Filters, you gain a powerful tool for improving application performance and scalability in many domains.
Conclusion
Bloom Filters represent a potent technology for any software engineer or web developer who is interested in efficient data processing. They strike a balance between speed, memory usage, and misclassification risk—making them invaluable in real-world applications. Whether you're developing a caching layer for a high-traffic website or designing a mobile app with constrained resources, Bloom Filters can enhance your system's performance.
Now that you have a solid foundation, it’s time to experiment. Try integrating Bloom Filters into your own projects and see firsthand how they can optimize your data queries. Consider exploring variations like Counted Bloom Filters if deletion is necessary for your application design.
Further Resources
- An In-Depth Explanation of Bloom Filters – For a more theoretical background.
Feel free to explore these additional resources to enrich your understanding and application of Bloom Filters in your next project.
Now that you've seen the foundational principles and practical implementations of Bloom Filters, imagine how you can adapt this knowledge to optimize everything from caching mechanisms to robust spam detection systems. What additional challenges or scenarios do you foresee Bloom Filters being invaluable in? Happy coding!