Better input method for C#
Well when you read a string from the user in C# language, you don't really have control of the string while the user is typing, now don't worry! Buffer package for C# solves the problem. Discovery of the Problem I was coding an CLI based chat client and server application and I was using multi-threading for receiving the messages from other users (clients). But what if I was typing and another user also sent the message, so it appeared broken like this, I did not have control of the value entered by the user, since we can only get the value after the user have pressed enter, so to fix it I have created this package. With this package you can improve your console input while having full control. How to use its maximum potential? We have to use buffer and multi-threading. Buffer and multi-threading go hand in hand! Here is an code example : using prad; class sample_program { static prad_buffer buffer = new prad_buffer(); static void Main(string[] args) { Thread thread = new Thread(invoker); thread.Start(); buffer.get_input("command > "); string value = buffer.get_buffer_as_string(); buffer.clear_buffer(); Console.WriteLine(value); } static void invoker(){ if(buffer.length > 0) Console.WriteLine(buffer.get_buffer_as_string()); Thread.Sleep(1000); invoker(); } } In this program, we get what user have entered BEFORE they even press enter. We cannot do that in Console.ReadLine()! Buffer management We are responsible for our input, so we are also responsible in clearing the buffer, providing even more flexibility! The clear_buffer() method clears the buffer! Example code to get input from user The below code shows how you can get input from user! prad_buffer buffer = new prad_buffer(); buffer.get_input("command > "); string value = buffer.get_buffer_as_string(); buffer.clear_buffer(); Console.WriteLine(value); How to get the package? You can visit the following links to add the package! nuget.org Github (source code) or just run the following command in your project, dotnet add package buffer Conclusion Hey if you still didn't know, I am the author of the blog post and this package! If you like my project or this blog, please put a ❤️. Have any questions? Comment below and I will try to hit you up as soon as possible!
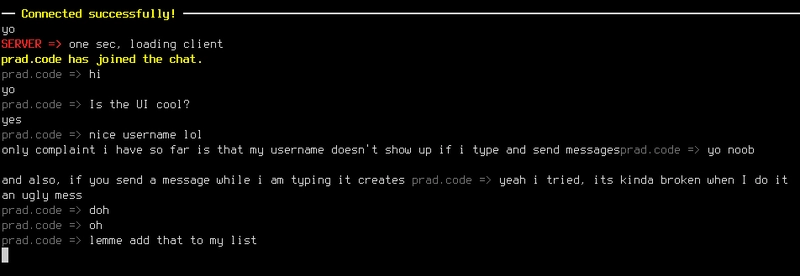
Well when you read a string from the user in C# language, you don't really have control of the string while the user is typing, now don't worry! Buffer package for C# solves the problem.
Discovery of the Problem
I was coding an CLI based chat client and server application and I was using multi-threading for receiving the messages from other users (clients). But what if I was typing and another user also sent the message, so it appeared broken like this,
I did not have control of the value entered by the user, since we can only get the value after the user have pressed enter, so to fix it I have created this package. With this package you can improve your console input while having full control.
How to use its maximum potential?
We have to use buffer and multi-threading. Buffer and multi-threading go hand in hand!
Here is an code example :
using prad;
class sample_program {
static prad_buffer buffer = new prad_buffer();
static void Main(string[] args) {
Thread thread = new Thread(invoker);
thread.Start();
buffer.get_input("command > ");
string value = buffer.get_buffer_as_string();
buffer.clear_buffer();
Console.WriteLine(value);
}
static void invoker(){
if(buffer.length > 0)
Console.WriteLine(buffer.get_buffer_as_string());
Thread.Sleep(1000);
invoker();
}
}
In this program, we get what user have entered BEFORE they even press enter.
We cannot do that in Console.ReadLine()
!
Buffer management
We are responsible for our input, so we are also responsible in clearing the buffer, providing even more flexibility!
The clear_buffer()
method clears the buffer!
Example code to get input from user
The below code shows how you can get input from user!
prad_buffer buffer = new prad_buffer();
buffer.get_input("command > ");
string value = buffer.get_buffer_as_string();
buffer.clear_buffer();
Console.WriteLine(value);
How to get the package?
You can visit the following links to add the package!
or just run the following command in your project,
dotnet add package buffer
Conclusion
Hey if you still didn't know, I am the author of the blog post and this package! If you like my project or this blog, please put a ❤️. Have any questions? Comment below and I will try to hit you up as soon as possible!