Beginner’s Guide to Recursion in Java
Note: This is a summary of Beginner’s Guide to Recursion in Java by Maaike van Putten. For full details and examples, check out the original post on Zero To Mastery. In This Guide What is recursion? How recursion works in Java (and what’s happening behind the scenes) The two golden rules of recursion Simple examples to get it into your brain When (and when not) to use recursion What to remember so recursion finally makes sense What is recursion? Recursion is when a method calls itself to solve a problem by breaking it down into smaller, self-similar pieces. Each call handles a smaller version of the problem, continuing until it reaches a point (the “base case”) where it can return a direct answer and stop. Analogy: Think of Russian nesting dolls—each doll contains a smaller one inside, just like recursive calls continue until you hit the smallest one. How recursion works in Java (and what’s happening behind the scenes) To write a recursive method in Java, you need: A way for the method to call itself. A condition that tells it when to stop (the base case). Each recursive call creates its own block of memory on the call stack. Java keeps track of these calls until the base case is reached, at which point the stack unwinds. Example: public void sayAlright(int count) { if (count == 0) { return; // base case } System.out.println("Alright"); sayAlright(count - 1); // recursive call } Call sayAlright(3) and it prints “Alright” three times, unwinding when it reaches the base case. The two golden rules of recursion 1. Always have a base case: This condition tells your method when to stop calling itself. 2. Always move toward the base case: Each recursive call must operate on a simpler or smaller problem, so you eventually hit the base case. Simple examples to get it into your brain Example 1: Countdown public void countdown(int number) { if (number
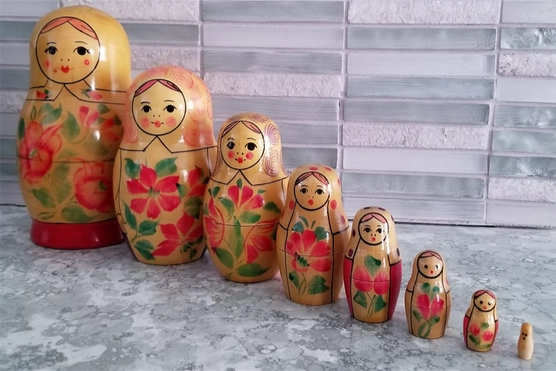
Note: This is a summary of Beginner’s Guide to Recursion in Java by Maaike van Putten. For full details and examples, check out the original post on Zero To Mastery.
In This Guide
- What is recursion?
- How recursion works in Java (and what’s happening behind the scenes)
- The two golden rules of recursion
- Simple examples to get it into your brain
- When (and when not) to use recursion
- What to remember so recursion finally makes sense
What is recursion?
Recursion is when a method calls itself to solve a problem by breaking it down into smaller, self-similar pieces. Each call handles a smaller version of the problem, continuing until it reaches a point (the “base case”) where it can return a direct answer and stop.
Analogy:
Think of Russian nesting dolls—each doll contains a smaller one inside, just like recursive calls continue until you hit the smallest one.
How recursion works in Java (and what’s happening behind the scenes)
To write a recursive method in Java, you need:
- A way for the method to call itself.
- A condition that tells it when to stop (the base case).
Each recursive call creates its own block of memory on the call stack. Java keeps track of these calls until the base case is reached, at which point the stack unwinds.
Example:
public void sayAlright(int count) {
if (count == 0) {
return; // base case
}
System.out.println("Alright");
sayAlright(count - 1); // recursive call
}
Call sayAlright(3)
and it prints “Alright” three times, unwinding when it reaches the base case.
The two golden rules of recursion
1. Always have a base case:
This condition tells your method when to stop calling itself.
2. Always move toward the base case:
Each recursive call must operate on a simpler or smaller problem, so you eventually hit the base case.
Simple examples to get it into your brain
Example 1: Countdown
public void countdown(int number) {
if (number < 0) {
return;
}
System.out.println(number);
countdown(number - 1);
}
Call countdown(5)
, and it prints numbers from 5 down to 0.
Example 2: Factorial
public int factorial(int n) {
if (n == 1) {
return 1;
}
return n * factorial(n - 1);
}
Factorial of 5 (factorial(5)
) computes as 5 * 4 * 3 * 2 * 1 = 120.
Example 3: Fibonacci (with a warning)
public int fibonacci(int n) {
if (n <= 1) {
return n;
}
return fibonacci(n - 1) + fibonacci(n - 2);
}
While this technically works, it’s very inefficient for large numbers due to repeated calculations.
When (and when not) to use recursion
Use recursion when:
- Problems can be broken into smaller, self-similar pieces (like trees or divide-and-conquer algorithms)
- The problem’s structure fits a recursive approach (e.g., traversing trees, recursive backtracking)
Avoid recursion when:
- The input size may create too many recursive calls (risking a StackOverflowError)
- An iterative solution is simpler or more readable
- Efficiency is a concern, especially for problems like naive Fibonacci calculation
Rule of thumb:
Use recursion for naturally recursive problems and manageable input sizes. Otherwise, loops may be better.
What to remember so recursion finally makes sense
Recursion isn’t magic—each call is just another method on the call stack, waiting its turn to finish. Remember the two golden rules: have a base case, and always move toward it.
Practice with small, real problems to make recursion feel logical and natural!