A Guide to SOLID Principles in Software Development
What is SOLID Principles? SOLID is a set of five design principles that improve software maintainability, scalability, and flexibility. These principles help developers write clean, robust, and scalable code. 1. Single Responsibility Principle (SRP) A class should have only one reason to change. Each class should focus on a single responsibility. If a class is handling multiple concerns, it's harder to maintain and modify. ✅ Example: A User class should handle only user-related logic, while a UserRepository class should manage database operations. 2. Open/Closed Principle (OCP) Software entities should be open for extension but closed for modification. Instead of modifying existing code, extend it through inheritance or interfaces. ✅ Example: Use polymorphism to add new functionality without altering existing code. 3. Liskov Substitution Principle (LSP) Objects of a superclass should be replaceable with objects of a subclass without breaking the system. A subclass should enhance, not alter, the expected behavior of the parent class. ✅ Example: If Rectangle is a parent class and Square is a subclass, overriding width or height independently should not break calculations. 4. Interface Segregation Principle (ISP) A class should not be forced to implement interfaces it doesn’t use. Instead of one large interface, create smaller, more specific ones. ✅ Example: Instead of a MultifunctionPrinter interface with Print(), Scan(), and Fax(), split it into separate IPrinter, IScanner, and IFax interfaces. 5. Dependency Inversion Principle (DIP) High-level modules should not depend on low-level modules. Both should depend on abstractions. Use dependency injection to decouple components. ✅ Example: Instead of a Service class directly instantiating a Database, inject an IDatabase interface that different database implementations can follow. Why SOLID Matters? Makes code easier to maintain and extend Reduces bugs and improves testability Enhances collaboration in large teams By applying SOLID, you’ll write cleaner, more efficient, and scalable software. Which of these principles do you struggle with the most? Let’s discuss in the comments!
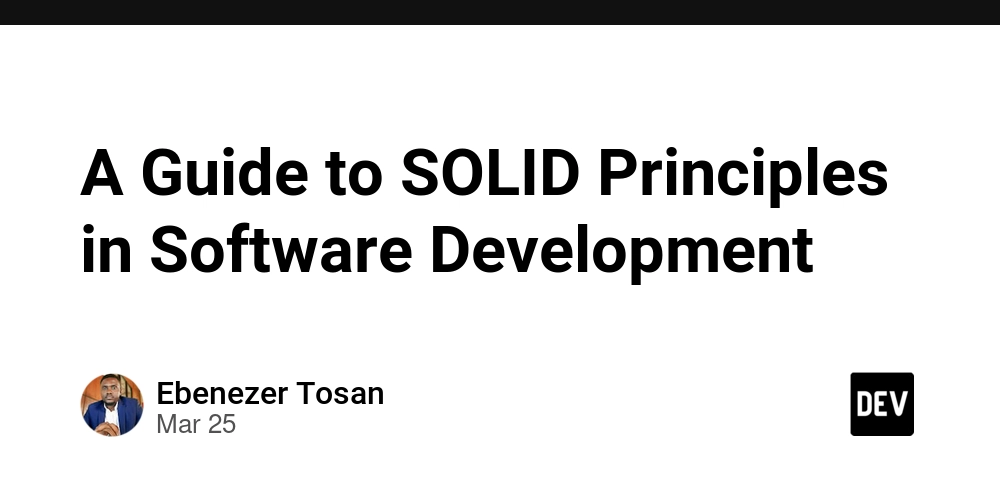
What is SOLID Principles?
SOLID is a set of five design principles that improve software maintainability, scalability, and flexibility. These principles help developers write clean, robust, and scalable code.
1. Single Responsibility Principle (SRP)
A class should have only one reason to change.
Each class should focus on a single responsibility. If a class is handling multiple concerns, it's harder to maintain and modify.
✅ Example: A User class should handle only user-related logic, while a UserRepository class should manage database operations.
2. Open/Closed Principle (OCP)
Software entities should be open for extension but closed for modification.
Instead of modifying existing code, extend it through inheritance or interfaces.
✅ Example: Use polymorphism to add new functionality without altering existing code.
3. Liskov Substitution Principle (LSP)
Objects of a superclass should be replaceable with objects of a subclass without breaking the system.
A subclass should enhance, not alter, the expected behavior of the parent class.
✅ Example: If Rectangle is a parent class and Square is a subclass, overriding width or height independently should not break calculations.
4. Interface Segregation Principle (ISP)
A class should not be forced to implement interfaces it doesn’t use.
Instead of one large interface, create smaller, more specific ones.
✅ Example: Instead of a MultifunctionPrinter interface with Print(), Scan(), and Fax(), split it into separate IPrinter, IScanner, and IFax interfaces.
5. Dependency Inversion Principle (DIP)
High-level modules should not depend on low-level modules. Both should depend on abstractions.
Use dependency injection to decouple components.
✅ Example: Instead of a Service class directly instantiating a Database, inject an IDatabase interface that different database implementations can follow.
Why SOLID Matters?
Makes code easier to maintain and extend
Reduces bugs and improves testability
Enhances collaboration in large teams
By applying SOLID, you’ll write cleaner, more efficient, and scalable software. Which of these principles do you struggle with the most? Let’s discuss in the comments!