5 Python Techniques to Master Kubernetes Container Orchestration
As a best-selling author, I invite you to explore my books on Amazon. Don't forget to follow me on Medium and show your support. Thank you! Your support means the world! Python techniques have revolutionized how I interact with Kubernetes for container orchestration. After working with numerous enterprise deployments, I've identified five powerful approaches that significantly improve development workflows and operational efficiency. Kubernetes Python Client The official Kubernetes Python client provides a comprehensive API for interacting with clusters programmatically. I've found this approach particularly valuable for automation tasks and CI/CD pipelines. Working with the client begins with establishing cluster connectivity: from kubernetes import client, config # Load configuration from default location (~/.kube/config) config.load_kube_config() # For in-cluster configuration (when running in a pod) # config.load_incluster_config() # Initialize the API clients core_v1 = client.CoreV1Api() apps_v1 = client.AppsV1Api() Resource management becomes straightforward with the client. For example, to list all pods across namespaces: def list_all_pods(): print("Listing pods across all namespaces:") pods = core_v1.list_pod_for_all_namespaces(watch=False) for pod in pods.items: print(f"Namespace: {pod.metadata.namespace}, Name: {pod.metadata.name}") print(f" Status: {pod.status.phase}") print(f" IP: {pod.status.pod_ip}") # Print container details for container in pod.spec.containers: print(f" Container: {container.name}, Image: {container.image}") print("---") Creating resources programmatically offers significant flexibility. Here's how I create deployments: def create_deployment(name, image, namespace="default", replicas=1, labels=None): labels = labels or {"app": name} # Define container container = client.V1Container( name=name, image=image, ports=[client.V1ContainerPort(container_port=80)], resources=client.V1ResourceRequirements( requests={"cpu": "100m", "memory": "200Mi"}, limits={"cpu": "500m", "memory": "500Mi"} ) ) # Create pod template template = client.V1PodTemplateSpec( metadata=client.V1ObjectMeta(labels=labels), spec=client.V1PodSpec(containers=[container]) ) # Create deployment spec spec = client.V1DeploymentSpec( replicas=replicas, selector=client.V1LabelSelector(match_labels=labels), template=template ) # Create deployment deployment = client.V1Deployment( api_version="apps/v1", kind="Deployment", metadata=client.V1ObjectMeta(name=name, namespace=namespace), spec=spec ) # Submit the deployment return apps_v1.create_namespaced_deployment(namespace=namespace, body=deployment) Watching resources provides real-time updates on cluster changes: def watch_pods(namespace="default", timeout_seconds=60): w = watch.Watch() for event in w.stream(core_v1.list_namespaced_pod, namespace=namespace, timeout_seconds=timeout_seconds): print(f"Event: {event['type']}") print(f"Pod: {event['object'].metadata.name}") print(f"Status: {event['object'].status.phase}") print("---") Custom Kubernetes Operators with Kopf I've built several custom controllers using Kopf (Kubernetes Operator Pythonic Framework), which simplifies creating operators that extend Kubernetes functionality. A basic operator structure looks like this: import kopf import kubernetes import yaml @kopf.on.create('example.com', 'v1', 'myresources') def create_fn(spec, name, namespace, logger, **kwargs): logger.info(f"Creating MyResource: {name} in {namespace}") # Extract information from the custom resource spec size = spec.get('size', 1) image = spec.get('image', 'nginx:latest') # Define the deployment to create deployment = { 'apiVersion': 'apps/v1', 'kind': 'Deployment', 'metadata': { 'name': name, 'namespace': namespace }, 'spec': { 'replicas': size, 'selector': { 'matchLabels': { 'app': name } }, 'template': { 'metadata': { 'labels': { 'app': name } }, 'spec': { 'containers': [{ 'name': name, 'image': image }] } } } } # Create the deployment api = kubernetes.client.AppsV1Api() api.create_namespaced_deployment( namespace=namespace, body=deployment ) # Return information to be stored in status return {'depl
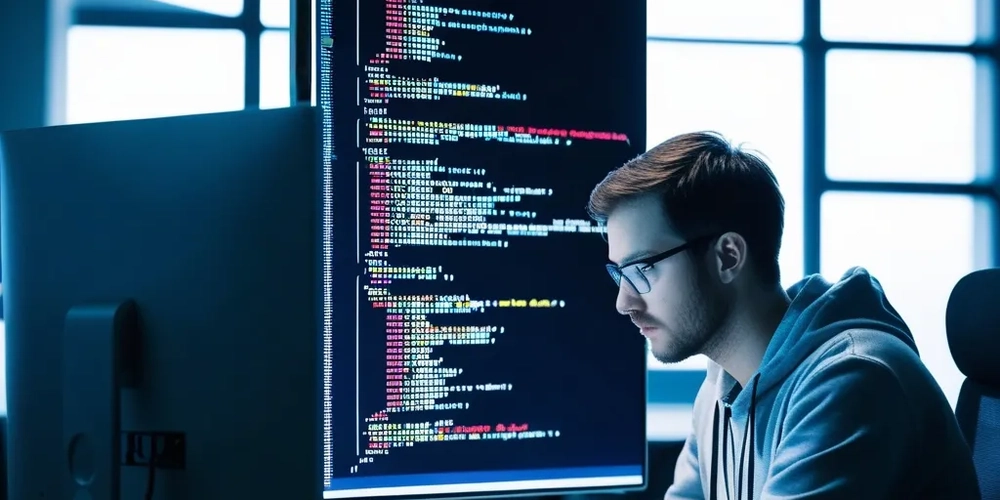
As a best-selling author, I invite you to explore my books on Amazon. Don't forget to follow me on Medium and show your support. Thank you! Your support means the world!
Python techniques have revolutionized how I interact with Kubernetes for container orchestration. After working with numerous enterprise deployments, I've identified five powerful approaches that significantly improve development workflows and operational efficiency.
Kubernetes Python Client
The official Kubernetes Python client provides a comprehensive API for interacting with clusters programmatically. I've found this approach particularly valuable for automation tasks and CI/CD pipelines.
Working with the client begins with establishing cluster connectivity:
from kubernetes import client, config
# Load configuration from default location (~/.kube/config)
config.load_kube_config()
# For in-cluster configuration (when running in a pod)
# config.load_incluster_config()
# Initialize the API clients
core_v1 = client.CoreV1Api()
apps_v1 = client.AppsV1Api()
Resource management becomes straightforward with the client. For example, to list all pods across namespaces:
def list_all_pods():
print("Listing pods across all namespaces:")
pods = core_v1.list_pod_for_all_namespaces(watch=False)
for pod in pods.items:
print(f"Namespace: {pod.metadata.namespace}, Name: {pod.metadata.name}")
print(f" Status: {pod.status.phase}")
print(f" IP: {pod.status.pod_ip}")
# Print container details
for container in pod.spec.containers:
print(f" Container: {container.name}, Image: {container.image}")
print("---")
Creating resources programmatically offers significant flexibility. Here's how I create deployments:
def create_deployment(name, image, namespace="default", replicas=1, labels=None):
labels = labels or {"app": name}
# Define container
container = client.V1Container(
name=name,
image=image,
ports=[client.V1ContainerPort(container_port=80)],
resources=client.V1ResourceRequirements(
requests={"cpu": "100m", "memory": "200Mi"},
limits={"cpu": "500m", "memory": "500Mi"}
)
)
# Create pod template
template = client.V1PodTemplateSpec(
metadata=client.V1ObjectMeta(labels=labels),
spec=client.V1PodSpec(containers=[container])
)
# Create deployment spec
spec = client.V1DeploymentSpec(
replicas=replicas,
selector=client.V1LabelSelector(match_labels=labels),
template=template
)
# Create deployment
deployment = client.V1Deployment(
api_version="apps/v1",
kind="Deployment",
metadata=client.V1ObjectMeta(name=name, namespace=namespace),
spec=spec
)
# Submit the deployment
return apps_v1.create_namespaced_deployment(namespace=namespace, body=deployment)
Watching resources provides real-time updates on cluster changes:
def watch_pods(namespace="default", timeout_seconds=60):
w = watch.Watch()
for event in w.stream(core_v1.list_namespaced_pod, namespace=namespace, timeout_seconds=timeout_seconds):
print(f"Event: {event['type']}")
print(f"Pod: {event['object'].metadata.name}")
print(f"Status: {event['object'].status.phase}")
print("---")
Custom Kubernetes Operators with Kopf
I've built several custom controllers using Kopf (Kubernetes Operator Pythonic Framework), which simplifies creating operators that extend Kubernetes functionality.
A basic operator structure looks like this:
import kopf
import kubernetes
import yaml
@kopf.on.create('example.com', 'v1', 'myresources')
def create_fn(spec, name, namespace, logger, **kwargs):
logger.info(f"Creating MyResource: {name} in {namespace}")
# Extract information from the custom resource spec
size = spec.get('size', 1)
image = spec.get('image', 'nginx:latest')
# Define the deployment to create
deployment = {
'apiVersion': 'apps/v1',
'kind': 'Deployment',
'metadata': {
'name': name,
'namespace': namespace
},
'spec': {
'replicas': size,
'selector': {
'matchLabels': {
'app': name
}
},
'template': {
'metadata': {
'labels': {
'app': name
}
},
'spec': {
'containers': [{
'name': name,
'image': image
}]
}
}
}
}
# Create the deployment
api = kubernetes.client.AppsV1Api()
api.create_namespaced_deployment(
namespace=namespace,
body=deployment
)
# Return information to be stored in status
return {'deployment_created': True}
@kopf.on.update('example.com', 'v1', 'myresources')
def update_fn(spec, status, name, namespace, logger, **kwargs):
logger.info(f"Updating MyResource: {name} in {namespace}")
# Check if we need to update the deployment
if not status.get('create_fn', {}).get('deployment_created', False):
logger.warning(f"Deployment not found for: {name}")
return
# Get updated values
size = spec.get('size', 1)
# Update the deployment
api = kubernetes.client.AppsV1Api()
deployment = api.read_namespaced_deployment(name=name, namespace=namespace)
deployment.spec.replicas = size
api.patch_namespaced_deployment(
name=name,
namespace=namespace,
body=deployment
)
return {'deployment_updated': True}
@kopf.on.delete('example.com', 'v1', 'myresources')
def delete_fn(spec, name, namespace, logger, **kwargs):
logger.info(f"Deleting MyResource: {name} from {namespace}")
# Delete the deployment
api = kubernetes.client.AppsV1Api()
api.delete_namespaced_deployment(
name=name,
namespace=namespace
)
Complex operators can implement advanced reconciliation loops and handle various Kubernetes events:
@kopf.on.field('example.com', 'v1', 'myresources', field='spec.template')
def template_changed(old, new, name, namespace, logger, **kwargs):
logger.info(f"Template changed for {name}: {old} -> {new}")
# Implement custom logic for template changes
# ...
@kopf.timer('example.com', 'v1', 'myresources', interval=60.0)
def periodic_check(spec, name, namespace, logger, **kwargs):
logger.info(f"Periodic check for {name}")
# Implement health checks or other periodic tasks
# ...
Dynamic Manifest Generation with Jinja2
Template-based manifest generation has improved my consistency across environments. Using Jinja2 with Python, I create dynamic Kubernetes configurations:
from jinja2 import Environment, FileSystemLoader
import yaml
import os
def generate_manifests(template_dir, template_name, output_dir, values):
# Create Jinja2 environment
env = Environment(loader=FileSystemLoader(template_dir))
template = env.get_template(template_name)
# Render template with values
rendered_content = template.render(**values)
# Parse YAML documents
documents = yaml.safe_load_all(rendered_content)
# Create output directory if needed
os.makedirs(output_dir, exist_ok=True)
# Write each document to a file
for doc in documents:
if not doc: # Skip empty documents
continue
kind = doc.get('kind', 'Unknown')
name = doc.get('metadata', {}).get('name', 'unnamed')
filename = f"{kind.lower()}-{name}.yaml"
with open(os.path.join(output_dir, filename), 'w') as file:
yaml.dump(doc, file)
return True
A typical template might look like:
# deployment.yaml.j2
apiVersion: apps/v1
kind: Deployment
metadata:
name: {{ app_name }}
namespace: {{ namespace }}
labels:
app: {{ app_name }}
spec:
replicas: {{ replicas }}
selector:
matchLabels:
app: {{ app_name }}
template:
metadata:
labels:
app: {{ app_name }}
spec:
containers:
- name: {{ app_name }}
image: {{ image }}:{{ tag }}
ports:
- containerPort: {{ port }}
resources:
requests:
cpu: {{ resources.requests.cpu }}
memory: {{ resources.requests.memory }}
limits:
cpu: {{ resources.limits.cpu }}
memory: {{ resources.limits.memory }}
{% if env_vars %}
env:
{% for key, value in env_vars.items() %}
- name: {{ key }}
value: "{{ value }}"
{% endfor %}
{% endif %}
Generating environment-specific manifests becomes straightforward:
# Values for different environments
environments = {
'dev': {
'app_name': 'myapp',
'namespace': 'development',
'replicas': 1,
'image': 'myapp',
'tag': 'latest',
'port': 8080,
'resources': {
'requests': {'cpu': '100m', 'memory': '128Mi'},
'limits': {'cpu': '200m', 'memory': '256Mi'}
},
'env_vars': {
'DEBUG': 'true',
'LOG_LEVEL': 'debug'
}
},
'prod': {
'app_name': 'myapp',
'namespace': 'production',
'replicas': 3,
'image': 'myapp',
'tag': 'v1.2.3',
'port': 8080,
'resources': {
'requests': {'cpu': '500m', 'memory': '512Mi'},
'limits': {'cpu': '1000m', 'memory': '1Gi'}
},
'env_vars': {
'DEBUG': 'false',
'LOG_LEVEL': 'info'
}
}
}
# Generate manifests for each environment
for env_name, values in environments.items():
generate_manifests(
template_dir='templates',
template_name='deployment.yaml.j2',
output_dir=f'manifests/{env_name}',
values=values
)
Kubernetes Resource Monitoring and Analytics
Monitoring is critical for production Kubernetes clusters. I've implemented Python-based solutions that collect metrics and provide insights:
import time
from kubernetes import client, config
import pandas as pd
import matplotlib.pyplot as plt
from datetime import datetime
# Configure Kubernetes client
config.load_kube_config()
v1 = client.CoreV1Api()
apps_v1 = client.AppsV1Api()
metrics_api = client.CustomObjectsApi()
def collect_pod_metrics(namespace=None):
"""Collect pod metrics from the Kubernetes Metrics API."""
if namespace:
metrics = metrics_api.list_namespaced_custom_object(
group="metrics.k8s.io",
version="v1beta1",
namespace=namespace,
plural="pods"
)
else:
metrics = metrics_api.list_cluster_custom_object(
group="metrics.k8s.io",
version="v1beta1",
plural="pods"
)
return metrics
def collect_node_metrics():
"""Collect node metrics from the Kubernetes Metrics API."""
metrics = metrics_api.list_cluster_custom_object(
group="metrics.k8s.io",
version="v1beta1",
plural="nodes"
)
return metrics
def analyze_pod_resource_usage(namespace=None, sample_count=10, interval=30):
"""Analyze pod resource usage over time."""
all_data = []
print(f"Collecting {sample_count} samples at {interval}s intervals...")
for i in range(sample_count):
timestamp = datetime.now()
metrics = collect_pod_metrics(namespace)
for pod in metrics['items']:
pod_name = pod['metadata']['name']
pod_namespace = pod['metadata']['namespace']
for container in pod['containers']:
container_name = container['name']
cpu_usage = container['usage']['cpu']
memory_usage = container['usage']['memory']
# Convert CPU usage to millicores
if cpu_usage.endswith('n'):
cpu_millicores = float(cpu_usage[:-1]) / 1000000
else:
cpu_millicores = float(cpu_usage)
# Convert memory usage to Mi
if memory_usage.endswith('Ki'):
memory_mi = float(memory_usage[:-2]) / 1024
elif memory_usage.endswith('Mi'):
memory_mi = float(memory_usage[:-2])
elif memory_usage.endswith('Gi'):
memory_mi = float(memory_usage[:-2]) * 1024
else:
memory_mi = float(memory_usage) / (1024 * 1024)
all_data.append({
'timestamp': timestamp,
'namespace': pod_namespace,
'pod': pod_name,
'container': container_name,
'cpu_millicores': cpu_millicores,
'memory_mi': memory_mi
})
if i < sample_count - 1:
time.sleep(interval)
# Convert to DataFrame for analysis
df = pd.DataFrame(all_data)
# Aggregate by pod
pod_stats = df.groupby(['namespace', 'pod']).agg({
'cpu_millicores': ['mean', 'max', 'std'],
'memory_mi': ['mean', 'max', 'std']
}).reset_index()
# Plot resource usage over time
for pod_name in df['pod'].unique():
pod_data = df[df['pod'] == pod_name]
fig, ax = plt.subplots(2, 1, figsize=(12, 8))
# CPU usage
ax[0].plot(pod_data['timestamp'], pod_data['cpu_millicores'])
ax[0].set_title(f'CPU Usage for Pod: {pod_name}')
ax[0].set_ylabel('CPU (millicores)')
ax[0].grid(True)
# Memory usage
ax[1].plot(pod_data['timestamp'], pod_data['memory_mi'])
ax[1].set_title(f'Memory Usage for Pod: {pod_name}')
ax[1].set_ylabel('Memory (Mi)')
ax[1].grid(True)
plt.tight_layout()
plt.savefig(f"{pod_name.replace('/', '_')}_resources.png")
return df, pod_stats
This analysis informs resource optimization decisions and capacity planning.
GitOps Automation with Python
I've implemented GitOps workflows using Python to automate the deployment pipeline:
import os
import git
import yaml
import subprocess
import logging
from kubernetes import client, config
# Setup logging
logging.basicConfig(level=logging.INFO,
format='%(asctime)s - %(name)s - %(levelname)s - %(message)s')
logger = logging.getLogger('gitops-controller')
def clone_or_pull_repository(repo_url, branch='main', target_dir='./repo'):
"""Clone or pull the latest changes from the Git repository."""
if os.path.exists(target_dir):
# Pull latest changes
logger.info(f"Pulling latest changes from {repo_url}")
repo = git.Repo(target_dir)
origin = repo.remotes.origin
origin.pull()
else:
# Clone repository
logger.info(f"Cloning repository {repo_url}")
git.Repo.clone_from(repo_url, target_dir, branch=branch)
# Return the repository object
return git.Repo(target_dir)
def scan_for_manifests(directory):
"""Scan directory for Kubernetes manifests."""
manifests = []
# Walk through all files in the directory
for root, _, files in os.walk(directory):
for file in files:
if file.endswith(('.yaml', '.yml')):
file_path = os.path.join(root, file)
logger.info(f"Found manifest: {file_path}")
manifests.append(file_path)
return manifests
def apply_manifest(manifest_path):
"""Apply Kubernetes manifest using kubectl."""
try:
logger.info(f"Applying manifest: {manifest_path}")
result = subprocess.run(['kubectl', 'apply', '-f', manifest_path],
capture_output=True, text=True, check=True)
logger.info(f"Successfully applied: {result.stdout}")
return True
except subprocess.CalledProcessError as e:
logger.error(f"Failed to apply manifest {manifest_path}: {e.stderr}")
return False
def gitops_sync(repo_url, manifests_dir='kubernetes', branch='main'):
"""Synchronize the Git repository with the Kubernetes cluster."""
target_dir = f"./repos/{repo_url.split('/')[-1].replace('.git', '')}"
# Clone or pull the repository
repo = clone_or_pull_repository(repo_url, branch, target_dir)
# Get the full path to the manifests directory
manifests_path = os.path.join(target_dir, manifests_dir)
# Ensure the directory exists
if not os.path.exists(manifests_path):
logger.error(f"Manifests directory {manifests_path} does not exist")
return False
# Scan for manifests
manifests = scan_for_manifests(manifests_path)
# Sort manifests to apply in a specific order (CRDs first, etc.)
# This is a simple implementation, you might want to enhance it
sorted_manifests = sorted(manifests)
# Apply manifests
success_count = 0
for manifest in sorted_manifests:
if apply_manifest(manifest):
success_count += 1
logger.info(f"Applied {success_count}/{len(sorted_manifests)} manifests successfully")
return success_count == len(sorted_manifests)
def watch_repository_and_sync(repo_url, interval=300, manifests_dir='kubernetes', branch='main'):
"""Watch a Git repository and sync changes to the Kubernetes cluster."""
import time
while True:
logger.info(f"Syncing repository {repo_url}")
gitops_sync(repo_url, manifests_dir, branch)
logger.info(f"Sleeping for {interval} seconds")
time.sleep(interval)
# Example usage
if __name__ == "__main__":
# Load Kubernetes configuration
config.load_kube_config()
# Start watching a repository
watch_repository_and_sync(
repo_url="https://github.com/example/kubernetes-manifests.git",
interval=300, # 5 minutes
manifests_dir="k8s",
branch="main"
)
This approach ensures configuration consistency and enables version-controlled infrastructure with proper audit trails.
My experience with these Python techniques has transformed how I manage Kubernetes environments. The combination of automation, programmatic APIs, and templating provides both flexibility and robust control. These approaches have enabled me to handle clusters with hundreds of workloads efficiently while maintaining high reliability standards.
The power of Python for Kubernetes orchestration is in simplifying complex workflows and providing consistent, repeatable operations. By implementing these techniques, you can modernize your container management approach and achieve better operational outcomes.
101 Books
101 Books is an AI-driven publishing company co-founded by author Aarav Joshi. By leveraging advanced AI technology, we keep our publishing costs incredibly low—some books are priced as low as $4—making quality knowledge accessible to everyone.
Check out our book Golang Clean Code available on Amazon.
Stay tuned for updates and exciting news. When shopping for books, search for Aarav Joshi to find more of our titles. Use the provided link to enjoy special discounts!
Our Creations
Be sure to check out our creations:
Investor Central | Investor Central Spanish | Investor Central German | Smart Living | Epochs & Echoes | Puzzling Mysteries | Hindutva | Elite Dev | JS Schools
We are on Medium
Tech Koala Insights | Epochs & Echoes World | Investor Central Medium | Puzzling Mysteries Medium | Science & Epochs Medium | Modern Hindutva