Customizing Material UI Themes Without Losing Accessibility
Material UI is a premier component library for React, widely appreciated for its comprehensive theming capabilities that enhance UI consistency and scalability. It is particularly valuable for developers aiming to implement customizable and dynamic design systems in their applications. In this blog, we’ll explore the integration of Material UI theming with Next.js, a framework that supports advanced features like server-side rendering. This combination not only improves application performance but also boosts SEO, making it crucial for modern web development. We will guide you through setting up, customizing, and effectively utilizing Material UI themes react with in a Next.js project, ensuring you can tailor your app to meet specific design needs. Setting Up the Environment To begin using Material UI with Next.js, you first need to set up your development environment. Here’s how you can get started: Create a Next.js Project: Start by creating a new Next.js application if you haven’t already. Use the command line to run the following command, which utilizes the create-next-app utility: npx create-next-app my-next-material-ui-appcd my-next-material-ui-app This sets up a new Next.js project in the directory my-next-material-ui-app and prepares it for further development. Install Material-UI: Next, add Material UI to your project. Run the following command to install the core components and icons of Material UI: npm install @mui/material @emotion/react @emotion/styled @mui/icons-material These packages include the Material UI component library and the necessary styling engines (@emotion/react and @emotion/styled) that Material UI uses for styling components. Initial Configuration: After installing the necessary packages, configure Material UI in your Next.js project. Start by creating a theme: Create a new file called theme.js in the root of your project or under a specific directory like styles. Inside theme.js, set up a basic theme configuration using Material UI createTheme function: import { createTheme } from "@mui/material/styles"; const theme = createTheme({ palette: { primary: { main: "#556cd6", }, secondary: { main: "#19857b", }, }, typography: { fontFamily: '"Roboto", "Helvetica", "Arial", sans-serif', fontSize: 14, button: { textTransform: "none", }, }, }); export default theme; This script defines a custom theme with specified colors for primary and secondary palettes, and some basic typography settings. You can customize these values based on your design requirements. By completing these steps, your Next.js project will be fully set up to use Material UI, allowing you to proceed with further customization and development of your application. Understanding Material-UI Theming Material UI theming is a powerful feature that enables developers to apply consistent styles across an entire application. Here’s what you need to know to effectively work with Material-UI theming: Default Theme Object: Material UI provides a default theme object that includes a wide range of styling options such as colors, typography, and breakpoints. This object is highly customizable and serves as the foundation for theme customization. To see what’s included in the default theme, you can log it to the console in your application: import { createTheme } from "@mui/material/styles"; const theme = createTheme(); console.log(theme); // View the default theme structure and values Exploring the default theme can help you understand what can be customized. How Theming Works: The core of Material-UI theming lies in its ThemeProvider component, which is used to wrap your application or specific parts of it with a theme. The ThemeProvider injects the theme into your components via context, allowing any child component to access the theme properties automatically. Here’s a simple example of how to use ThemeProvider: import { ThemeProvider } from "@mui/material/styles"; import theme from "./theme"; // Assuming you've created a theme.js file function App() { return ( {/* Your component tree goes here */} ); } export default App This setup ensures that any Material-UI component used within the ThemeProvider will inherit the theme’s styles. Importance of Theming: Effective theming can drastically improve the user experience by providing a uniform look and feel across your application. It also simplifies the management of the app’s visual aspects, allowing changes to be propagated throughout the app by adjusting a single theme configuration file. This central control over styling is especially beneficial in larger projects where maintaining consistency can become challenging. Customizing the Theme Creating a custom theme in Material-UI allows you to define a distinct visual identity for your application. Here’s how to customize your theme step-by-step: Cre
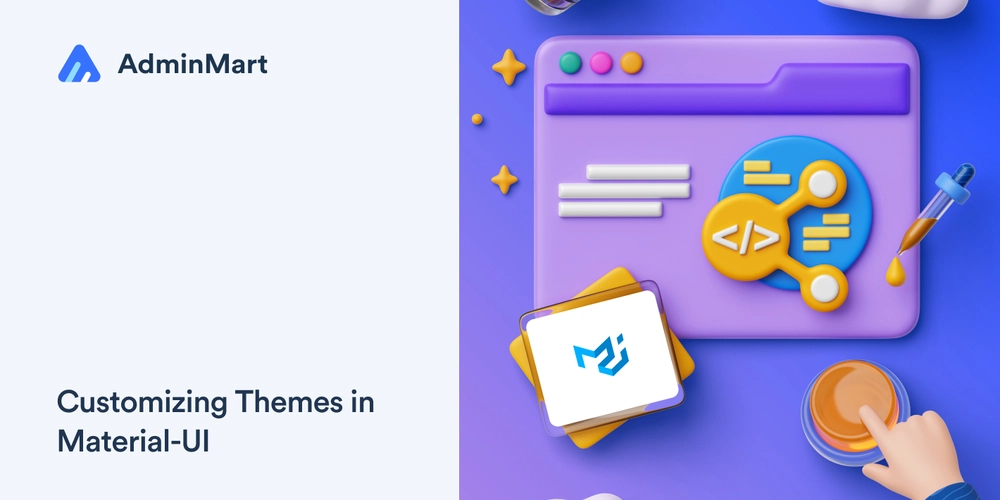
Material UI is a premier component library for React, widely appreciated for its comprehensive theming capabilities that enhance UI consistency and scalability. It is particularly valuable for developers aiming to implement customizable and dynamic design systems in their applications.
In this blog, we’ll explore the integration of Material UI theming with Next.js, a framework that supports advanced features like server-side rendering. This combination not only improves application performance but also boosts SEO, making it crucial for modern web development. We will guide you through setting up, customizing, and effectively utilizing Material UI themes react with in a Next.js project, ensuring you can tailor your app to meet specific design needs.
Setting Up the Environment
To begin using Material UI with Next.js, you first need to set up your development environment. Here’s how you can get started:
Create a Next.js Project: Start by creating a new Next.js application if you haven’t already. Use the command line to run the following command, which utilizes the create-next-app
utility:
npx create-next-app my-next-material-ui-app
cd my-next-material-ui-app
This sets up a new Next.js project in the directory my-next-material-ui-app
and prepares it for further development.
Install Material-UI: Next, add Material UI to your project. Run the following command to install the core components and icons of Material UI:
npm install @mui/material @emotion/react @emotion/styled @mui/icons-material
These packages include the Material UI component library and the necessary styling engines (@emotion/react
and @emotion/styled
) that Material UI uses for styling components.
Initial Configuration: After installing the necessary packages, configure Material UI in your Next.js project. Start by creating a theme:
- Create a new file called
theme.js
in the root of your project or under a specific directory likestyles
. - Inside
theme.js
, set up a basic theme configuration using Material UIcreateTheme
function:
import { createTheme } from "@mui/material/styles";
const theme = createTheme({
palette: {
primary: {
main: "#556cd6",
},
secondary: {
main: "#19857b",
},
},
typography: {
fontFamily: '"Roboto", "Helvetica", "Arial", sans-serif',
fontSize: 14,
button: {
textTransform: "none",
},
},
});
export default theme;
This script defines a custom theme with specified colors for primary and secondary palettes, and some basic typography settings. You can customize these values based on your design requirements.
By completing these steps, your Next.js project will be fully set up to use Material UI, allowing you to proceed with further customization and development of your application.
Understanding Material-UI Theming
Material UI theming is a powerful feature that enables developers to apply consistent styles across an entire application. Here’s what you need to know to effectively work with Material-UI theming:
Default Theme Object: Material UI provides a default theme object that includes a wide range of styling options such as colors, typography, and breakpoints. This object is highly customizable and serves as the foundation for theme customization.
To see what’s included in the default theme, you can log it to the console in your application:
import { createTheme } from "@mui/material/styles";
const theme = createTheme();
console.log(theme); // View the default theme structure and values
Exploring the default theme can help you understand what can be customized.
How Theming Works: The core of Material-UI theming lies in its ThemeProvider
component, which is used to wrap your application or specific parts of it with a theme. The ThemeProvider
injects the theme into your components via context, allowing any child component to access the theme properties automatically.
Here’s a simple example of how to use ThemeProvider
:
import { ThemeProvider } from "@mui/material/styles";
import theme from "./theme"; // Assuming you've created a theme.js file
function App() {
return (
<ThemeProvider theme={theme}>
{/* Your component tree goes here */}
</ThemeProvider>
);
}
export default App
This setup ensures that any Material-UI component used within the ThemeProvider
will inherit the theme’s styles.
Importance of Theming: Effective theming can drastically improve the user experience by providing a uniform look and feel across your application. It also simplifies the management of the app’s visual aspects, allowing changes to be propagated throughout the app by adjusting a single theme configuration file. This central control over styling is especially beneficial in larger projects where maintaining consistency can become challenging.
Customizing the Theme
Creating a custom theme in Material-UI allows you to define a distinct visual identity for your application. Here’s how to customize your theme step-by-step:
Creating a Custom Theme: Use the createTheme
function provided by Material-UI to override the default theme settings according to your design requirements. You can customize various aspects such as colors, typography, and breakpoints.
Here’s an example of how to modify the color palette and typography in your theme configuration:
import { createTheme } from "@mui/material/styles";
const theme = createTheme({
palette: {
primary: {
main: "#334ACC", // A custom blue shade
},
secondary: {
main: "#F50057", // A vibrant pink shade
},
},
typography: {
fontFamily: '"Arial", sans-serif',
h1: {
fontSize: "2.2rem",
fontWeight: 500,
},
body1: {
fontSize: "1rem",
fontWeight: 400,
},
},
});
Applying the Custom Theme Globally: To make your custom theme effective throughout your application, wrap your component tree with the ThemeProvider
from Material-UI. This should be done at the top level of your app, typically in files like layout.js
for Next.js projects.
import { Inter } from "next/font/google";
import { ThemeProvider } from "@mui/material/styles";
const inter = Inter({ subsets: ["latin"] });
export default function RootLayout({ children }) {
return (
<html lang="en">
<body className={inter.className}>
<ThemeProvider theme={theme}>{children}</ThemeProvider>
</body>
</html>
);
}
Code Snippets for Theme Customization: It’s often helpful to include practical examples in your code to show the customization in action. For instance, you might want to adjust the Button component’s color based on your theme:
import Button from "@mui/material/Button";
function CustomButton() {
return (
<Button color="primary">Click Me</Button> // This button uses the primary color from your theme
);
}
By following these steps, you can create a tailored experience in your application that aligns perfectly with your brand’s identity or the desired aesthetics.
Advanced Theming Techniques
To further enhance the visual impact and flexibility of your application, let’s explore some advanced theming techniques with Material-UI. These methods allow for dynamic theming, use of multiple themes, and specific component styling.
Dynamic Theming: Dynamic theming enables the application to switch themes based on user preferences or system settings, which can significantly improve user experience, especially in terms of accessibility and personalization.
Here’s an example of implementing dynamic theming in a React component using state:
import { useState } from "react";
import { ThemeProvider, createTheme } from "@mui/material/styles";
import Button from "@mui/material/Button";
function DynamicTheme() {
const [darkMode, setDarkMode] = useState(false);
const theme = createTheme({
palette: {
mode: darkMode ? "dark" : "light",
},
});
return (
<ThemeProvider theme={theme}>
<Button onClick={() => setDarkMode(!darkMode)}>Toggle Theme</Button>
{/* Rest of your component */}
</ThemeProvider>
);
}
This component allows users to toggle between light and dark modes, showcasing the flexibility of dynamic theming.
Using Multiple Themes: Sometimes, different sections of your application might require distinct themes. Material-UI supports the use of multiple themes simultaneously.
Here’s how you might apply different themes to different parts of your application:
import { ThemeProvider, createTheme } from "@mui/material/styles";
import Layout from "./layout";
import Profile from "./Profile";
import Dashboard from "./Dashboard";
const dashboardTheme = createTheme({
palette: {
primary: {
main: "#007BFF",
},
},
});
const profileTheme = createTheme({
palette: {
primary: {
main: "#004D40",
},
},
});
function App() {
return (
<Layout>
<ThemeProvider theme={dashboardTheme}>
<Dashboard />
</ThemeProvider>
<ThemeProvider theme={profileTheme}>
<Profile />
</ThemeProvider>
</Layout>
);
}
This approach provides visual diversity and helps maintain a cohesive look within specific contexts.
Overriding Styles: Material-UI allows for fine-grained control over component styles via the styled
utility or the makeStyles
function. You can target specific components to customize their styles beyond what is available through theming.
Here’s an example using the styled
API:
import { styled } from "@mui/material/styles";
import Button from "@mui/material/Button";
const CustomButton = styled(Button)({
backgroundColor: "gold",
color: "black",
"&:hover": {
backgroundColor: "goldenrod",
},
});
function StyledComponent() {
return <CustomButton>Custom Style Button</CustomButton>;
}
This customization applies specific styles to the Button component, making it stand out from other elements styled by the global theme.
Also read - Top 5 Books to learn Web Development.
By leveraging these advanced theming techniques, developers can provide a richer, more engaging user experience that caters to a broader range of user preferences and requirements.
Conclusion
In this blog, we’ve explored how to effectively utilize Material UI theming capabilities within a Next.js environment. The ability to customize themes allows you to align your application with specific branding and user preferences, enhancing both aesthetics and functionality.
We encourage you to experiment with the various theming techniques covered here. Share your findings and experiences with the community to foster innovation and collaboration. By pushing the boundaries of UI design, you can create more engaging and user-friendly applications.