10 Powerful CSS Tips and Tricks That Will Elevate Your Web Design
10 Powerful CSS Tips and Tricks That Will Elevate Your Web Design After 13+ years as a graphic designer and several years diving into web development, I've collected numerous CSS techniques that have consistently helped me create more polished, efficient, and visually appealing websites. Today, I'm sharing my top 10 CSS tips and tricks that can immediately elevate your web design projects. 1. Use CSS Custom Properties for Theme Switching Custom properties (CSS variables) are perfect for implementing theme switching with minimal code: :root { --primary-color: #2a3990; --secondary-color: #e6f0ff; --text-color: #333333; --background-color: #ffffff; } [data-theme="dark"] { --primary-color: #4e5ed3; --secondary-color: #1a1f3d; --text-color: #f0f0f0; --background-color: #121212; } body { background-color: var(--background-color); color: var(--text-color); } .button { background-color: var(--primary-color); color: var(--secondary-color); } Theme switching becomes as simple as toggling a data attribute: document.documentElement.setAttribute('data-theme', 'dark'); 2. Perfect Centered Elements with Grid Forget about the old margin/transform hacks. CSS Grid makes centering elements incredibly simple: .container { display: grid; place-items: center; min-height: 100vh; } This three-line solution works for any content, regardless of its dimensions or whether its height/width is known. 3. Create Responsive Typography with clamp() The clamp() function allows your typography to scale smoothly between viewport sizes without media queries: h1 { font-size: clamp(2rem, 5vw + 1rem, 5rem); } p { font-size: clamp(1rem, 1vw + 0.75rem, 1.5rem); } This ensures text is never too small on mobile or too large on desktop, all with one line of CSS. 4. Use aspect-ratio for Responsive Media Maintain perfect aspect ratios for images, videos, and other media: .video-container { aspect-ratio: 16 / 9; width: 100%; } .profile-image { aspect-ratio: 1 / 1; width: 250px; object-fit: cover; } This prevents layout shifts and removes the need for padding-based hacks. 5. Create Smooth Animations with CSS Variables Combine CSS variables with transitions for dynamic, interactive animations: .button { --scale: 1; --rotation: 0deg; transform: scale(var(--scale)) rotate(var(--rotation)); transition: transform 0.3s ease; } .button:hover { --scale: 1.1; --rotation: 5deg; } This approach gives you more flexibility than hardcoded values and makes complex animations easier to manage. 6. Master the :is() Selector for Cleaner Code The :is() pseudo-class can dramatically reduce CSS selector repetition: /* Instead of this */ header a:hover, main a:hover, footer a:hover { text-decoration: underline; } /* Use this */ :is(header, main, footer) a:hover { text-decoration: underline; } This makes your stylesheets more maintainable and easier to read. 7. Create Custom Scrollbars Customize scrollbars to match your site's design system: /* For Webkit browsers (Chrome, Safari) */ .custom-scrollbar::-webkit-scrollbar { width: 10px; } .custom-scrollbar::-webkit-scrollbar-track { background: #f1f1f1; border-radius: 10px; } .custom-scrollbar::-webkit-scrollbar-thumb { background: #888; border-radius: 10px; } .custom-scrollbar::-webkit-scrollbar-thumb:hover { background: #555; } /* For Firefox */ .custom-scrollbar { scrollbar-width: thin; scrollbar-color: #888 #f1f1f1; } This subtle detail can significantly enhance your UI's coherence and polish. 8. Use gap with Flexbox for Clean Layouts The gap property now works with flexbox, not just grid: .card-container { display: flex; flex-wrap: wrap; gap: 20px; } This eliminates the need for margin hacks and makes your spacing consistent. 9. Create Diagonal Layouts with CSS Clip-Path Use clip-path to create modern, angular section dividers: .diagonal-section { clip-path: polygon(0 0, 100% 0, 100% 85%, 0 100%); padding: 100px 20px; } .diagonal-card { clip-path: polygon(0 0, 100% 0, 95% 100%, 5% 100%); } This technique adds visual interest and creates a dynamic flow between page sections. 10. Optimize Animations with will-change Improve performance for animated elements by hinting to the browser: .animated-element { will-change: transform, opacity; transition: transform 0.3s ease, opacity 0.3s ease; } Use sparingly and only for elements that actually change, as overuse can negatively impact performance. Bonus: Debug Layouts with One Line When something breaks in your layout, add this temporary CSS to quickly identify issues: * { outline: 1px solid red !important; } This highlights every element on the page, making it easier to spot alignment problems, overflow issues, or unexpected margin
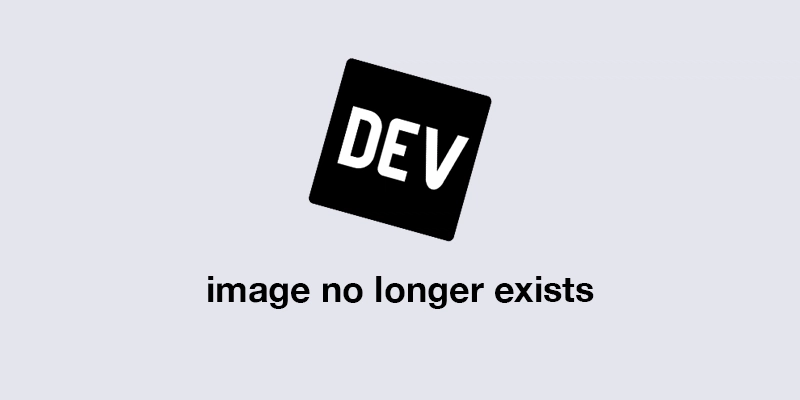
10 Powerful CSS Tips and Tricks That Will Elevate Your Web Design
After 13+ years as a graphic designer and several years diving into web development, I've collected numerous CSS techniques that have consistently helped me create more polished, efficient, and visually appealing websites. Today, I'm sharing my top 10 CSS tips and tricks that can immediately elevate your web design projects.
1. Use CSS Custom Properties for Theme Switching
Custom properties (CSS variables) are perfect for implementing theme switching with minimal code:
:root {
--primary-color: #2a3990;
--secondary-color: #e6f0ff;
--text-color: #333333;
--background-color: #ffffff;
}
[data-theme="dark"] {
--primary-color: #4e5ed3;
--secondary-color: #1a1f3d;
--text-color: #f0f0f0;
--background-color: #121212;
}
body {
background-color: var(--background-color);
color: var(--text-color);
}
.button {
background-color: var(--primary-color);
color: var(--secondary-color);
}
Theme switching becomes as simple as toggling a data attribute:
document.documentElement.setAttribute('data-theme', 'dark');
2. Perfect Centered Elements with Grid
Forget about the old margin/transform hacks. CSS Grid makes centering elements incredibly simple:
.container {
display: grid;
place-items: center;
min-height: 100vh;
}
This three-line solution works for any content, regardless of its dimensions or whether its height/width is known.
3. Create Responsive Typography with clamp()
The clamp()
function allows your typography to scale smoothly between viewport sizes without media queries:
h1 {
font-size: clamp(2rem, 5vw + 1rem, 5rem);
}
p {
font-size: clamp(1rem, 1vw + 0.75rem, 1.5rem);
}
This ensures text is never too small on mobile or too large on desktop, all with one line of CSS.
4. Use aspect-ratio for Responsive Media
Maintain perfect aspect ratios for images, videos, and other media:
.video-container {
aspect-ratio: 16 / 9;
width: 100%;
}
.profile-image {
aspect-ratio: 1 / 1;
width: 250px;
object-fit: cover;
}
This prevents layout shifts and removes the need for padding-based hacks.
5. Create Smooth Animations with CSS Variables
Combine CSS variables with transitions for dynamic, interactive animations:
.button {
--scale: 1;
--rotation: 0deg;
transform: scale(var(--scale)) rotate(var(--rotation));
transition: transform 0.3s ease;
}
.button:hover {
--scale: 1.1;
--rotation: 5deg;
}
This approach gives you more flexibility than hardcoded values and makes complex animations easier to manage.
6. Master the :is() Selector for Cleaner Code
The :is()
pseudo-class can dramatically reduce CSS selector repetition:
/* Instead of this */
header a:hover,
main a:hover,
footer a:hover {
text-decoration: underline;
}
/* Use this */
:is(header, main, footer) a:hover {
text-decoration: underline;
}
This makes your stylesheets more maintainable and easier to read.
7. Create Custom Scrollbars
Customize scrollbars to match your site's design system:
/* For Webkit browsers (Chrome, Safari) */
.custom-scrollbar::-webkit-scrollbar {
width: 10px;
}
.custom-scrollbar::-webkit-scrollbar-track {
background: #f1f1f1;
border-radius: 10px;
}
.custom-scrollbar::-webkit-scrollbar-thumb {
background: #888;
border-radius: 10px;
}
.custom-scrollbar::-webkit-scrollbar-thumb:hover {
background: #555;
}
/* For Firefox */
.custom-scrollbar {
scrollbar-width: thin;
scrollbar-color: #888 #f1f1f1;
}
This subtle detail can significantly enhance your UI's coherence and polish.
8. Use gap with Flexbox for Clean Layouts
The gap
property now works with flexbox, not just grid:
.card-container {
display: flex;
flex-wrap: wrap;
gap: 20px;
}
This eliminates the need for margin hacks and makes your spacing consistent.
9. Create Diagonal Layouts with CSS Clip-Path
Use clip-path
to create modern, angular section dividers:
.diagonal-section {
clip-path: polygon(0 0, 100% 0, 100% 85%, 0 100%);
padding: 100px 20px;
}
.diagonal-card {
clip-path: polygon(0 0, 100% 0, 95% 100%, 5% 100%);
}
This technique adds visual interest and creates a dynamic flow between page sections.
10. Optimize Animations with will-change
Improve performance for animated elements by hinting to the browser:
.animated-element {
will-change: transform, opacity;
transition: transform 0.3s ease, opacity 0.3s ease;
}
Use sparingly and only for elements that actually change, as overuse can negatively impact performance.
Bonus: Debug Layouts with One Line
When something breaks in your layout, add this temporary CSS to quickly identify issues:
* {
outline: 1px solid red !important;
}
This highlights every element on the page, making it easier to spot alignment problems, overflow issues, or unexpected margins.
Conclusion
These CSS techniques have consistently helped me create more polished and professional websites. What makes these tricks particularly valuable is how they combine simplicity with power—they're easy to implement but can dramatically improve both the aesthetics and functionality of your projects.
The best part is that these techniques work with modern browsers, letting you create sophisticated designs without relying on heavy JavaScript libraries or frameworks.
What are your favorite CSS tips and tricks? Share in the comments below!
[This post was written by Italo Germando, Graphic Designer and Web Developer with over 13 years of experience in visual design and interface creation.]