Zod vs Yup: Choosing the Right Validation Library for Your Frontend Project
In the world of frontend development, data validation plays a crucial role in building robust and user-friendly applications. It ensures data integrity, improves user experience, enhances server efficiency, strengthens security, and ultimately contributes to better user retention. By validating data against predefined schemas, we can prevent unexpected errors, maintain consistency, and safeguard our applications from vulnerabilities. Two prominent JavaScript libraries, Zod and Yup, have gained popularity as powerful tools for schema validation. This article provides a comprehensive comparison of Zod and Yup, exploring their core functionalities, key features, and common validation scenarios, empowering you to make an informed decision for your frontend project. Introduction to Zod and Yup Both Zod and Yup are schema validation libraries that offer a structured and declarative approach to defining and enforcing data validation rules. They provide a range of features to validate various data types, from simple primitives like strings and numbers to complex nested objects and arrays. However, they differ in their design philosophies and TypeScript integration. Zod is a schema declaration and validation library designed with a strong emphasis on TypeScript. It leverages TypeScript's type system to automatically infer types from your schema definitions, eliminating the need for separate type declarations. This feature makes Zod particularly appealing to TypeScript developers, as it ensures type safety and reduces code duplication. Yup, on the other hand, is a JavaScript-centric library that offers a more flexible and extensible approach to schema validation. While it is compatible with TypeScript, it doesn't provide the same level of automatic type inference as Zod. Key Features Comparison Feature Zod Yup Schema Definition Concise and expressive syntax with a focus on immutability and composability. Provides a fluent API for defining schemas. Declarative syntax with a focus on chaining validation methods. Offers a wide range of built-in validation methods. Validation Methods Offers a comprehensive set of built-in validation methods for common scenarios, including primitives, objects, arrays, unions, and more. Supports datetimes, dates, times, IP addresses, and IP ranges. Provides a rich set of validation methods, including those for required fields, string length, number ranges, regular expressions, and more. Key features include a concise schema interface, strong TypeScript support, async validation support, extensibility, and rich error details. Error Handling Provides detailed error objects with information about the validation failures, including error codes, paths, and messages. Offers custom error messages and supports asynchronous validation for complex scenarios. Customization Options Allows for custom validation rules through refinements and transformations. Supports asynchronous validation and conditional validation. Supports custom validation rules and conditional validation based on other field values. Community Support Has a growing and active community with good documentation and a variety of third-party integrations. Has a larger and more established community with extensive documentation and resources available. Performance Highly performant due to a lightweight structure and zero dependencies. Offers more features, but these may come with performance costs in larger applications. Integrations with Frontend Frameworks Both Zod and Yup integrate well with popular frontend frameworks, simplifying the process of incorporating validation into your applications. Zod offers integrations with form libraries such as React Hook Form and Formik through dedicated adapters. These integrations streamline the validation process by providing pre-built components and utilities that work seamlessly with Zod schemas. For example, the react-hook-form library provides a Zod resolver that automatically handles validation and error handling for your forms. Yup also has strong support for React form libraries, including Formik and React Hook Form. These integrations allow you to use Yup schemas directly within your form components, simplifying validation logic and ensuring data integrity. Code Examples Here are some code examples demonstrating how Zod and Yup can be used for common validation scenarios: Email Validation: This example demonstrates how to validate an email address using both Zod and Yup. Zod: import { z } from 'zod'; const emailSchema = z.string().email(); const result = emailSchema.safeParse('test@example.com'); if (result.success) { console.log('Valid email:', result.data); } else { console.error('Invalid email:', result.error); } Yup: import * as yup from 'yup'; const emailSchema = yup.string().email('Invalid email address').required('Email is required'); emailSchema.validate('test@example.com') .th
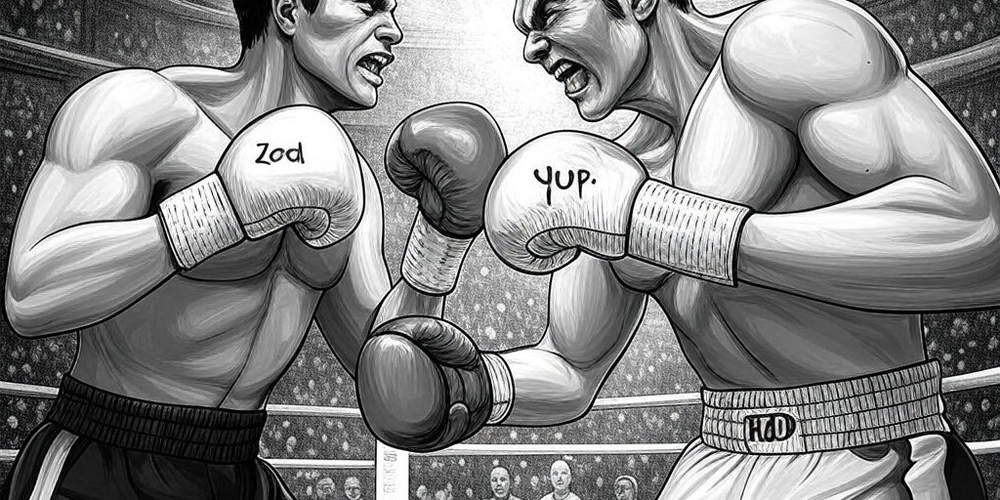
In the world of frontend development, data validation plays a crucial role in building robust and user-friendly applications. It ensures data integrity, improves user experience, enhances server efficiency, strengthens security, and ultimately contributes to better user retention. By validating data against predefined schemas, we can prevent unexpected errors, maintain consistency, and safeguard our applications from vulnerabilities. Two prominent JavaScript libraries, Zod and Yup, have gained popularity as powerful tools for schema validation. This article provides a comprehensive comparison of Zod and Yup, exploring their core functionalities, key features, and common validation scenarios, empowering you to make an informed decision for your frontend project.
Introduction to Zod and Yup
Both Zod and Yup are schema validation libraries that offer a structured and declarative approach to defining and enforcing data validation rules. They provide a range of features to validate various data types, from simple primitives like strings and numbers to complex nested objects and arrays. However, they differ in their design philosophies and TypeScript integration.
Zod is a schema declaration and validation library designed with a strong emphasis on TypeScript. It leverages TypeScript's type system to automatically infer types from your schema definitions, eliminating the need for separate type declarations. This feature makes Zod particularly appealing to TypeScript developers, as it ensures type safety and reduces code duplication.
Yup, on the other hand, is a JavaScript-centric library that offers a more flexible and extensible approach to schema validation. While it is compatible with TypeScript, it doesn't provide the same level of automatic type inference as Zod.
Key Features Comparison
Feature | Zod | Yup |
---|---|---|
Schema Definition | Concise and expressive syntax with a focus on immutability and composability. Provides a fluent API for defining schemas. | Declarative syntax with a focus on chaining validation methods. Offers a wide range of built-in validation methods. |
Validation Methods | Offers a comprehensive set of built-in validation methods for common scenarios, including primitives, objects, arrays, unions, and more. Supports datetimes, dates, times, IP addresses, and IP ranges. | Provides a rich set of validation methods, including those for required fields, string length, number ranges, regular expressions, and more. Key features include a concise schema interface, strong TypeScript support, async validation support, extensibility, and rich error details. |
Error Handling | Provides detailed error objects with information about the validation failures, including error codes, paths, and messages. | Offers custom error messages and supports asynchronous validation for complex scenarios. |
Customization Options | Allows for custom validation rules through refinements and transformations. Supports asynchronous validation and conditional validation. | Supports custom validation rules and conditional validation based on other field values. |
Community Support | Has a growing and active community with good documentation and a variety of third-party integrations. | Has a larger and more established community with extensive documentation and resources available. |
Performance | Highly performant due to a lightweight structure and zero dependencies. | Offers more features, but these may come with performance costs in larger applications. |
Integrations with Frontend Frameworks
Both Zod and Yup integrate well with popular frontend frameworks, simplifying the process of incorporating validation into your applications.
Zod offers integrations with form libraries such as React Hook Form and Formik through dedicated adapters. These integrations streamline the validation process by providing pre-built components and utilities that work seamlessly with Zod schemas. For example, the react-hook-form
library provides a Zod resolver that automatically handles validation and error handling for your forms.
Yup also has strong support for React form libraries, including Formik and React Hook Form. These integrations allow you to use Yup schemas directly within your form components, simplifying validation logic and ensuring data integrity.
Code Examples
Here are some code examples demonstrating how Zod and Yup can be used for common validation scenarios:
Email Validation:
This example demonstrates how to validate an email address using both Zod and Yup.
Zod:
import { z } from 'zod';
const emailSchema = z.string().email();
const result = emailSchema.safeParse('test@example.com');
if (result.success) {
console.log('Valid email:', result.data);
} else {
console.error('Invalid email:', result.error);
}
Yup:
import * as yup from 'yup';
const emailSchema = yup.string().email('Invalid email address').required('Email is required');
emailSchema.validate('test@example.com')
.then((validEmail) => {
console.log('Valid email:', validEmail);
})
.catch((err) => {
console.error('Validation failed:', err.errors);
});
Password Validation:
This example shows how to validate a password, ensuring it meets certain criteria such as minimum length.
Zod:
import { z } from 'zod';
const passwordSchema = z.string().min(8, 'Password must be at least 8 characters long');
const result = passwordSchema.safeParse('password123');
if (result.success) {
console.log('Valid password:', result.data);
} else {
console.error('Invalid password:', result.error);
}
Yup:
import * as yup from 'yup';
const passwordSchema = yup.string().min(8, 'Password must be at least 8 characters long').required('Password is required');
passwordSchema.validate('password123')
.then((validPassword) => {
console.log('Valid password:', validPassword);
})
.catch((err) => {
console.error('Validation failed:', err.errors);
});
Validating User Input with Nested Objects:
This example demonstrates how to validate user input with nested objects, ensuring the correct data types and structure.
Zod:
import { z } from 'zod';
const userSchema = z.object({
name: z.string().min(3),
age: z.number().positive(),
email: z.string().email(),
address: z.object({
street: z.string(),
city: z.string(),
country: z.string(),
}),
});
const userInput = {
name: 'Alice',
age: 29,
email: 'alice@example.com',
address: {
street: '123 Main St',
city: 'Anytown',
country: 'USA',
},
};
const validatedUser = userSchema.safeParse(userInput);
if (!validatedUser.success) {
console.error(validatedUser.error.format());
} else {
console.log('User input is valid:', validatedUser.data);
}
Yup:
import * as yup from 'yup';
const userSchema = yup.object().shape({
name: yup.string().required(),
age: yup.number().positive().integer(),
email: yup.string().email(),
address: yup.object().shape({
street: yup.string().required(),
city: yup.string().required(),
country: yup.string().required(),
}),
});
const userInput = {
name: 'Alice',
age: 29,
email: 'alice@example.com',
address: {
street: '123 Main St',
city: 'Anytown',
country: 'USA',
},
};
userSchema.validate(userInput)
.then((validInput) => {
console.log('Valid input:', validInput);
})
.catch((err) => {
console.error('Validation failed:', err.errors);
});
Conditional Validation:
This example shows how to perform conditional validation, where the validation rules for one field depend on the value of another field.
Zod:
import { z } from 'zod';
const schema = z.object({
hasDiscount: z.boolean(),
discountCode: z.string().optional(),
})
.refine((data) => !data.hasDiscount || data.discountCode !== undefined, {
message: 'Discount code is required if hasDiscount is true',
path: ['discountCode'],
});
const result = schema.safeParse({ hasDiscount: true });
if (result.success) {
console.log('Valid data:', result.data);
} else {
console.error('Invalid data:', result.error);
}
Yup:
import * as yup from 'yup';
const schema = yup.object().shape({
hasDiscount: yup.boolean(),
discountCode: yup.string().when('hasDiscount', {
is: true,
then: yup.string().required('Discount code is required'),
otherwise: yup.string().notRequired(),
}),
});
schema.validate({ hasDiscount: true })
.then((validData) => {
console.log('Valid data:', validData);
})
.catch((err) => {
console.error('Validation failed:', err.errors);
});
These examples illustrate how both Zod and Yup provide mechanisms for handling various validation scenarios, including nested objects and conditional validation.
Having seen how Zod and Yup work in practice, let's now weigh their advantages and disadvantages.
Pros and Cons
Zod:
Pros:
Excellent TypeScript integration: Zod's seamless integration with TypeScript provides strong type safety and eliminates the need for redundant type declarations, a major advantage for TypeScript developers.
Concise and expressive syntax: Zod's API is designed to be developer-friendly and easy to use, allowing for clear and readable schema definitions.
Lightweight and performant: Zod has a small bundle size and is optimized for performance, making it suitable for frontend applications. It also has zero dependencies, further contributing to its lightweight nature.
Cons:
- Smaller community compared to Yup: While Zod's community is growing rapidly, it is still smaller than Yup's, which may result in fewer readily available resources and third-party integrations.
Yup:
Pros:
Large and active community: Yup has a mature and active community, providing extensive documentation, tutorials, and support.
Extensive validation methods: Yup offers a wide range of built-in validation methods, covering a variety of common scenarios.
Flexible and extensible: Yup allows for custom validation rules and supports asynchronous validation, making it suitable for complex validation needs.
Cons:
Less robust TypeScript support: While Yup is compatible with TypeScript, it requires manual type definitions and doesn't offer the same level of automatic type inference as Zod.
Can be more verbose than Zod: Yup's syntax can be more verbose than Zod's, especially for complex schemas.
Best Practices
When using Yup for validation, it's essential to follow best practices to ensure code maintainability and efficiency.
Centralize Your Validation Schemas: Create a central location for your validation schemas to maintain consistency and reduce duplication. This approach promotes code reusability and simplifies updates.
Optimize Performance: For large forms, consider performance optimization techniques such as validation debouncing and field-level validation. These techniques can help improve the responsiveness of your forms and prevent unnecessary validation checks.
Conclusion
Choosing between Zod and Yup depends on your project's specific needs and priorities. If you're working on a TypeScript project and prioritize type safety and code conciseness, Zod is an excellent choice. Its seamless integration with TypeScript, developer-friendly API, and lightweight nature make it a powerful tool for ensuring data integrity. Zod's functional API design also promotes composability and reusability of validation logic, allowing you to create more maintainable and scalable code.
However, if you need a library with a wider range of built-in validation methods, a larger community, and greater flexibility, Yup might be a better fit. Its extensive features and mature ecosystem make it a reliable choice for complex validation scenarios.
Here's a more specific breakdown of recommendations:
For small to medium-sized TypeScript projects: Zod is generally recommended due to its type safety, conciseness, and performance benefits.
For larger TypeScript projects: Zod is still a strong contender, but carefully consider whether its smaller community and potentially limited built-in validation methods might pose challenges.
For JavaScript projects or projects where TypeScript is not a primary concern: Yup might be a better choice due to its larger community, extensive features, and flexibility.
Ultimately, the best way to decide is to experiment with both libraries and see which one best suits your development style and project requirements.