Why I Never Use Optimistic Updates (And Why You Might Regret It Too)
If you’ve built anything with React, Vue, or any modern frontend framework, you’ve probably come across the idea of optimistic UI updates. It sounds fancy. It sounds fast. It even feels good. Until it doesn’t. Let me tell you why I almost never update state optimistically anymore, and why you might want to think twice before doing it too. ⚡ What Is an Optimistic Update? Optimistic updates mean changing the UI before you know if the backend operation actually succeeded. For example, you click "like" on a post, and the heart icon turns red immediately, before the API call finishes. setLiked(true) // optimistic update await api.likePost(postId) If something fails, you roll it back: catch (error) { setLiked(false) // rollback } Seems harmless, right? ❌ Why I Stopped Using Optimistic Updates 1. Edge Cases Multiply Fast Every time you write an optimistic update, you're creating an alternate reality. Now you have to write logic to reconcile the optimistic state with the real state if: The request fails The data changes from another tab or user The user undoes the action before the request finishes The server responds with something unexpected like validation or sanitization That’s four edge cases for one button. Multiply that across a large app and your UI becomes a ticking time bomb. 2. Rollbacks Are a UX Nightmare Users hate seeing the UI revert. Imagine typing a message, seeing it appear instantly, and then it disappears because the server threw a 500. Yes, you can show toasts like “Action failed,” but most people don’t read those. It just feels like your app is broken or buggy. 3. It Breaks Consistency Your UI state and your backend state go out of sync on purpose when you use optimistic updates. That’s dangerous. Consistency between client and server is everything in multi-user apps. If you break that contract, you introduce bugs that are extremely hard to debug, especially when multiple users are involved. 4. You’re Not Facebook Optimistic updates are mostly popularized by companies that can afford massive infra and logic to handle them properly. You probably have: A few devs, maybe just you No conflict resolution backend No retry queue No live event stream syncing the UI Without all of that, optimism is a luxury you can’t afford.
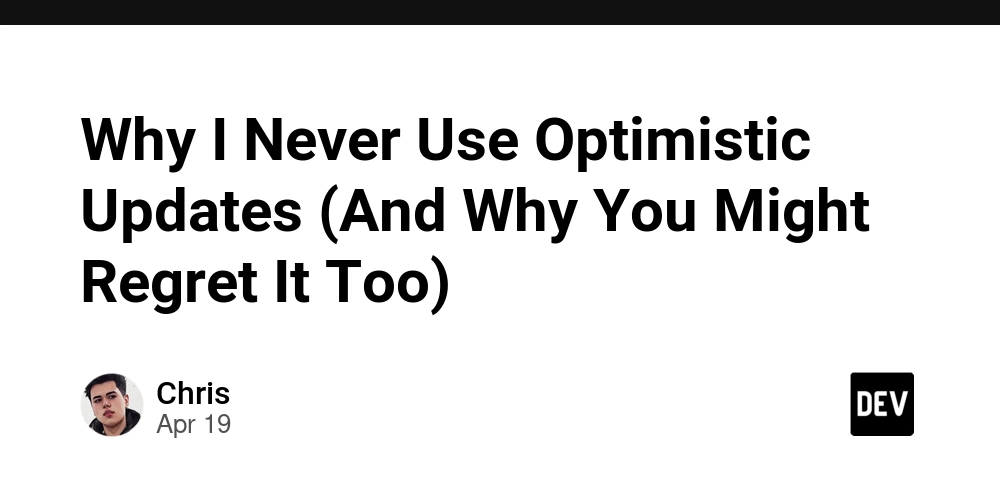
If you’ve built anything with React, Vue, or any modern frontend framework, you’ve probably come across the idea of optimistic UI updates. It sounds fancy. It sounds fast. It even feels good. Until it doesn’t.
Let me tell you why I almost never update state optimistically anymore, and why you might want to think twice before doing it too.
⚡ What Is an Optimistic Update?
Optimistic updates mean changing the UI before you know if the backend operation actually succeeded. For example, you click "like" on a post, and the heart icon turns red immediately, before the API call finishes.
setLiked(true) // optimistic update
await api.likePost(postId)
If something fails, you roll it back:
catch (error) {
setLiked(false) // rollback
}
Seems harmless, right?
❌ Why I Stopped Using Optimistic Updates
1. Edge Cases Multiply Fast
Every time you write an optimistic update, you're creating an alternate reality. Now you have to write logic to reconcile the optimistic state with the real state if:
- The request fails
- The data changes from another tab or user
- The user undoes the action before the request finishes
- The server responds with something unexpected like validation or sanitization
That’s four edge cases for one button. Multiply that across a large app and your UI becomes a ticking time bomb.
2. Rollbacks Are a UX Nightmare
Users hate seeing the UI revert. Imagine typing a message, seeing it appear instantly, and then it disappears because the server threw a 500.
Yes, you can show toasts like “Action failed,” but most people don’t read those. It just feels like your app is broken or buggy.
3. It Breaks Consistency
Your UI state and your backend state go out of sync on purpose when you use optimistic updates. That’s dangerous.
Consistency between client and server is everything in multi-user apps. If you break that contract, you introduce bugs that are extremely hard to debug, especially when multiple users are involved.
4. You’re Not Facebook
Optimistic updates are mostly popularized by companies that can afford massive infra and logic to handle them properly.
You probably have:
- A few devs, maybe just you
- No conflict resolution backend
- No retry queue
- No live event stream syncing the UI
Without all of that, optimism is a luxury you can’t afford.