What is `ssh` in Web Development?
Definition: SSH (Secure Shell) is a cryptographic network protocol used for secure remote access to systems, typically servers, over an unsecured network. It allows developers to log into a remote server, execute commands, transfer files, and manage web applications securely. Purpose: In web development, SSH is used to interact with remote servers hosting websites, APIs, or databases, enabling tasks like deploying code, configuring servers, or debugging issues. Why Use SSH in Web Development? Secure Remote Access: SSH encrypts all communication, ensuring that sensitive data (e.g., passwords, API keys) and commands are protected from interception. Essential for managing production servers securely. Server Management: Allows developers to configure web servers (e.g., Nginx, Apache), manage databases (e.g., MySQL, PostgreSQL), or install dependencies. Code Deployment: Used to push code updates to servers, either manually or as part of automated deployment pipelines (e.g., via Git or CI/CD tools). File Transfer: SSH-based tools like SCP (Secure Copy) or SFTP (SSH File Transfer Protocol) enable secure file transfers between local machines and servers. Debugging and Monitoring: Developers can access server logs, monitor performance, or troubleshoot issues directly on the server. How Does SSH Work? Basic Mechanism: SSH establishes a secure, encrypted connection between a client (your local machine) and a remote server using public-key cryptography or password-based authentication. The client runs an SSH client (e.g., OpenSSH on Linux/macOS, PuTTY on Windows), and the server runs an SSH daemon (e.g., sshd). Authentication: Password-Based: You provide a username and password to log in (less secure, often disabled on servers). Key-Based: You generate a public-private key pair. The public key is stored on the server, and the private key remains on your local machine. This is more secure and commonly used. Common Commands: Connect to a server: ssh user@server-ip (e.g., ssh john@192.168.1.100). Run a command remotely: ssh user@server-ip command (e.g., ssh john@192.168.1.100 ls). Transfer files: scp localfile user@server-ip:/remote/path or use SFTP. Configuration: SSH configurations are stored in ~/.ssh/config on the client side, allowing shortcuts for hosts, usernames, and keys. Server-side settings are in /etc/ssh/sshd_config, where admins can configure ports, authentication methods, etc. When is SSH Used in Web Development? Server Setup and Configuration: Initial setup of a web server (e.g., installing Node.js, setting up Nginx, configuring SSL certificates). Example: Logging into a cloud server (AWS EC2, DigitalOcean) to install dependencies. Code Deployment: Manually pushing code to a server via Git (git push) or copying files with SCP/SFTP. Example: ssh user@server 'cd /var/www && git pull' to update a website. Database Management: Accessing a remote database server to run queries, back up data, or restore databases. Example: ssh user@server 'mysqldump database > backup.sql'. Debugging and Maintenance: Checking server logs (e.g., /var/log/nginx/error.log) or restarting services (e.g., sudo systemctl restart nginx). Automation: Used in CI/CD pipelines (e.g., Jenkins, GitHub Actions) to deploy code to servers. Example: A GitHub Action uses SSH to connect to a server and run a deployment script. Tunneling: SSH tunneling allows secure access to services on a remote server (e.g., accessing a database on a private network). Example: ssh -L 3306:localhost:3306 user@server to access a remote MySQL database locally. How to Use SSH in Web Development (Practical Steps) Install an SSH Client: Linux/macOS: OpenSSH is usually pre-installed (ssh command). Windows: Use PowerShell, WSL, or tools like PuTTY or Git Bash. Generate SSH Keys (if using key-based authentication): Run ssh-keygen -t rsa -b 4096 to create a key pair (~/.ssh/id_rsa and ~/.ssh/id_rsa.pub). Copy the public key to the server: ssh-copy-id user@server-ip. Connect to a Server: Use ssh user@server-ip or configure ~/.ssh/config for easier access: Host myserver HostName 192.168.1.100 User john IdentityFile ~/.ssh/id_rsa Then connect with ssh myserver. Common Web Dev Tasks: Update a website: ssh user@server 'cd /var/www/html && git pull && npm install && pm2 restart app'. Transfer files: scp ./build.zip user@server:/var/www/. Check logs: ssh user@server 'tail -f /var/log/nginx/access.log'. Best Practices for SSH in Web Development Use Key-Based Authentication: Disable password logins in /etc/ssh/sshd_config for security. Change Default SSH Port: Modify the port (e.g., from 22 to 2222) to reduce automated attacks. Use Strong Keys: Prefer ED25519 or RSA with 4096 bits for key generation. Limit User Access: Restrict SSH access to specific users or IP addresses via /e
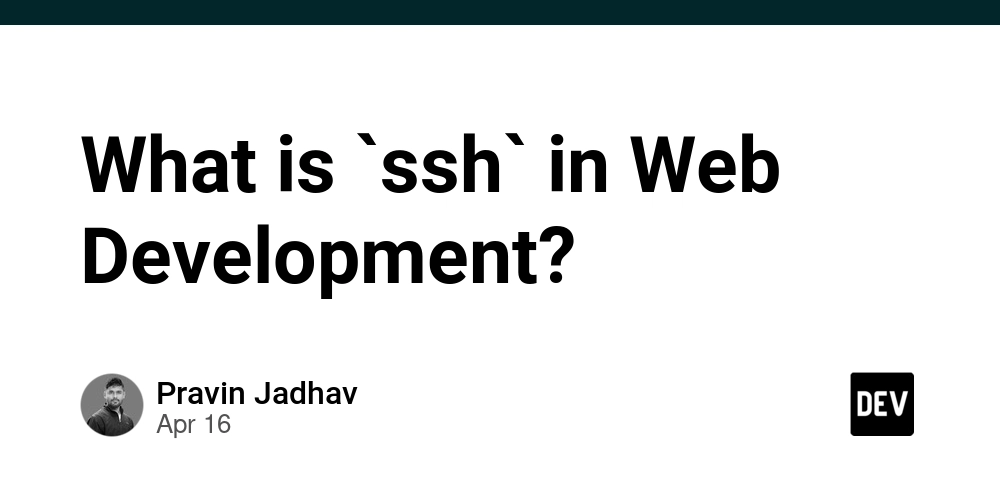
- Definition: SSH (Secure Shell) is a cryptographic network protocol used for secure remote access to systems, typically servers, over an unsecured network. It allows developers to log into a remote server, execute commands, transfer files, and manage web applications securely.
- Purpose: In web development, SSH is used to interact with remote servers hosting websites, APIs, or databases, enabling tasks like deploying code, configuring servers, or debugging issues.
Why Use SSH in Web Development?
-
Secure Remote Access:
- SSH encrypts all communication, ensuring that sensitive data (e.g., passwords, API keys) and commands are protected from interception.
- Essential for managing production servers securely.
-
Server Management:
- Allows developers to configure web servers (e.g., Nginx, Apache), manage databases (e.g., MySQL, PostgreSQL), or install dependencies.
-
Code Deployment:
- Used to push code updates to servers, either manually or as part of automated deployment pipelines (e.g., via Git or CI/CD tools).
-
File Transfer:
- SSH-based tools like SCP (Secure Copy) or SFTP (SSH File Transfer Protocol) enable secure file transfers between local machines and servers.
-
Debugging and Monitoring:
- Developers can access server logs, monitor performance, or troubleshoot issues directly on the server.
How Does SSH Work?
-
Basic Mechanism:
- SSH establishes a secure, encrypted connection between a client (your local machine) and a remote server using public-key cryptography or password-based authentication.
- The client runs an SSH client (e.g., OpenSSH on Linux/macOS, PuTTY on Windows), and the server runs an SSH daemon (e.g.,
sshd
).
-
Authentication:
- Password-Based: You provide a username and password to log in (less secure, often disabled on servers).
- Key-Based: You generate a public-private key pair. The public key is stored on the server, and the private key remains on your local machine. This is more secure and commonly used.
-
Common Commands:
- Connect to a server:
ssh user@server-ip
(e.g.,ssh john@192.168.1.100
). - Run a command remotely:
ssh user@server-ip command
(e.g.,ssh john@192.168.1.100 ls
). - Transfer files:
scp localfile user@server-ip:/remote/path
or use SFTP.
- Connect to a server:
-
Configuration:
- SSH configurations are stored in
~/.ssh/config
on the client side, allowing shortcuts for hosts, usernames, and keys. - Server-side settings are in
/etc/ssh/sshd_config
, where admins can configure ports, authentication methods, etc.
- SSH configurations are stored in
When is SSH Used in Web Development?
-
Server Setup and Configuration:
- Initial setup of a web server (e.g., installing Node.js, setting up Nginx, configuring SSL certificates).
- Example: Logging into a cloud server (AWS EC2, DigitalOcean) to install dependencies.
-
Code Deployment:
- Manually pushing code to a server via Git (
git push
) or copying files with SCP/SFTP. - Example:
ssh user@server 'cd /var/www && git pull'
to update a website.
- Manually pushing code to a server via Git (
-
Database Management:
- Accessing a remote database server to run queries, back up data, or restore databases.
- Example:
ssh user@server 'mysqldump database > backup.sql'
.
-
Debugging and Maintenance:
- Checking server logs (e.g.,
/var/log/nginx/error.log
) or restarting services (e.g.,sudo systemctl restart nginx
).
- Checking server logs (e.g.,
-
Automation:
- Used in CI/CD pipelines (e.g., Jenkins, GitHub Actions) to deploy code to servers.
- Example: A GitHub Action uses SSH to connect to a server and run a deployment script.
-
Tunneling:
- SSH tunneling allows secure access to services on a remote server (e.g., accessing a database on a private network).
- Example:
ssh -L 3306:localhost:3306 user@server
to access a remote MySQL database locally.
How to Use SSH in Web Development (Practical Steps)
-
Install an SSH Client:
- Linux/macOS: OpenSSH is usually pre-installed (
ssh
command). - Windows: Use PowerShell, WSL, or tools like PuTTY or Git Bash.
- Linux/macOS: OpenSSH is usually pre-installed (
-
Generate SSH Keys (if using key-based authentication):
- Run
ssh-keygen -t rsa -b 4096
to create a key pair (~/.ssh/id_rsa
and~/.ssh/id_rsa.pub
). - Copy the public key to the server:
ssh-copy-id user@server-ip
.
- Run
-
Connect to a Server:
- Use
ssh user@server-ip
or configure~/.ssh/config
for easier access:
Host myserver HostName 192.168.1.100 User john IdentityFile ~/.ssh/id_rsa
Then connect with
ssh myserver
. - Use
-
Common Web Dev Tasks:
- Update a website:
ssh user@server 'cd /var/www/html && git pull && npm install && pm2 restart app'
. - Transfer files:
scp ./build.zip user@server:/var/www/
. - Check logs:
ssh user@server 'tail -f /var/log/nginx/access.log'
.
- Update a website:
Best Practices for SSH in Web Development
-
Use Key-Based Authentication: Disable password logins in
/etc/ssh/sshd_config
for security. - Change Default SSH Port: Modify the port (e.g., from 22 to 2222) to reduce automated attacks.
- Use Strong Keys: Prefer ED25519 or RSA with 4096 bits for key generation.
-
Limit User Access: Restrict SSH access to specific users or IP addresses via
/etc/ssh/sshd_config
. - Backup Keys: Store your private key securely and never share it.
- Automate with Tools: Use SSH in combination with tools like Ansible, Docker, or CI/CD systems for streamlined workflows.
Common Tools and Alternatives
- SCP/SFTP: For file transfers.
- rsync: For efficient file synchronization over SSH.
- PuTTY: A Windows SSH client with a GUI.
- MobaXterm: A Windows tool for SSH and server management.
- Cloud Platforms: AWS, Google Cloud, and DigitalOcean provide SSH access to their instances, often with browser-based terminals as an alternative.
-
Alternatives to SSH:
- For deployment: Use managed services like Vercel, Netlify, or Heroku, which abstract SSH.
- For file transfers: Use FTP (less secure) or cloud storage (e.g., S3).
When Not to Use SSH
- Managed Hosting: If you’re using platforms like WordPress.com or Squarespace, SSH isn’t needed, as they handle server management.
-
Local Development: SSH is irrelevant for local tasks (e.g., running a dev server with
npm start
). - Frontend-Only Projects: If your project doesn’t involve server-side management (e.g., static sites on GitHub Pages), SSH may not be necessary.