What Exactly is Debouncing in JavaScript?
Table of contents Debouncing in electrical engineering Adaptation in software engineering Debouncing in JavaScript A general debouncing utility Things to note regarding debouncing Choose a debounce delay wisely Make sure that debouncing really helps As a web developer, performance optimization is not just a nice thing for you to know but rather a clear-cut must. No second questions in that. The web is a fragile medium. Bad performance could quickly — or perhaps, very quickly — backfire and leave the user of the web app in dismay. Performance optimization is such a large and complex topic that there could be a whole book on it (and there even are many). There are numerous ways of optimizing the performance of webpages, one of which that we shall cover here is debouncing. In this article, we'll get to learn what exactly is debouncing in JavaScrip; where did the concept originally come from; why we need it; how to implement a general debouncing utility; and some important things to note when debouncing. Let's get into it. Debouncing in electrical engineering Debouncing is originally a concept from electrical engineering. It helps to understand the whole intuition behind it in this field and then relate it back to software engineering. At the core, the issue is that when a push button (any kind) is pressed, instead of generating one push signal, as we typically expect, it produces many consecutive push signals before coming to a final, resting position. Imagine dropping a ball on the floor. As the ball hits the floor, it of course doesn't immediately come to a rest; rather, it bounces off the floor to a lesser height than before, then falls back again, then bounces off again, and so on and so forth. This constant back and forth motion of the ball, and even of the button, before coming to a final, resting position is referred to as bouncing. Bouncing in buttons has the undesirable consequence that one button press causes multiple, weaker and weaker signals. The circuitry, without any remedy, doesn't treat these signals any differently from the initial press but rather as normal signals too. The ultimate result is that during this phase of back and forth button oscillations, the circuitry completes and then breaks, causing swift and short-lived on/off signals in connected appliances. This sequence of fluctuations often tends to deteriorate electronic appliances or lead to falsey outcomes, and so we must rectify it. In simple words: As soon as we perform an action here (a button press), we get a quick burst of related actions (weaker presses), i.e. bouncing, that we do NOT want to respond to. The remedy is to prevent the bouncing, or as it's commonly known, to implement debouncing. Debouncing in electrical circuitry is performed using different kinds of components — RCs, Schmitt triggers, flip-flops — and ensures that only one signal ever reaches the end appliance for one button press. Thanks to debouncing, any signals generated immediately following an initial button press are effectively canceled out. Adaptation in software engineering The concept of debouncing has been extended and adapted accordingly into software engineering, especially in event-driven programming, where we deal with events. In event-driven programs, it's often the case that we have certain events that intrinsically fire at a rapid rate, for e.g. a key held down, sending rapid key-press events, or the mouse being moved, sending rapid mouse-move events, etc. These rapid events assimilate the 'bouncing' described above in that they form something that we want to ignore for the end program. A simple fix, i.e. debouncing, is to introduce a short delay, only a fraction of a second, during which any event is ignored. Only after this delay passes without any event firing is any subsequent event considered. For example, if a key on the keyboard is pressed quickly twice, with an interval of 80ms in between the presses, we'll ignore the second event given that we've asserted that there must be a delay greater than 100ms in order for any subsequent event to be considered as discrete. Debouncing in JavaScript Debouncing certainly wasn't pioneered by JavaScript; other languages have implemented it in the past before JavaScript, especially those that deal with event-driven programming and GUI development. But probably because of the widespread use of JavaScript, and the fact that JavaScript also works around an event-driven programming model, debouncing became a well-known concept amongst developers. Let's consider a quick example of debouncing in action in JavaScript. Let's say we have a search input field on a 3D modeling software's documentation page to search into the entire documentation (which is quite huge). As soon as a character is input into this input field, the user is provided with a list of suggestions. The entered data is submitted to the server and t
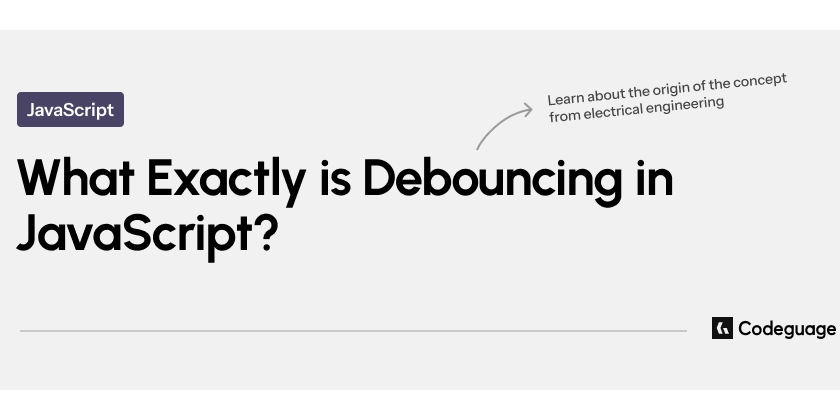
Table of contents
- Debouncing in electrical engineering
- Adaptation in software engineering
- Debouncing in JavaScript
- A general debouncing utility
-
Things to note regarding debouncing
- Choose a debounce delay wisely
- Make sure that debouncing really helps
As a web developer, performance optimization is not just a nice thing for you to know but rather a clear-cut must. No second questions in that. The web is a fragile medium. Bad performance could quickly — or perhaps, very quickly — backfire and leave the user of the web app in dismay.
Performance optimization is such a large and complex topic that there could be a whole book on it (and there even are many). There are numerous ways of optimizing the performance of webpages, one of which that we shall cover here is debouncing.
In this article, we'll get to learn what exactly is debouncing in JavaScrip; where did the concept originally come from; why we need it; how to implement a general debouncing utility; and some important things to note when debouncing.
Let's get into it.
Debouncing in electrical engineering
Debouncing is originally a concept from electrical engineering. It helps to understand the whole intuition behind it in this field and then relate it back to software engineering.
At the core, the issue is that when a push button (any kind) is pressed, instead of generating one push signal, as we typically expect, it produces many consecutive push signals before coming to a final, resting position.
Imagine dropping a ball on the floor. As the ball hits the floor, it of course doesn't immediately come to a rest; rather, it bounces off the floor to a lesser height than before, then falls back again, then bounces off again, and so on and so forth.
This constant back and forth motion of the ball, and even of the button, before coming to a final, resting position is referred to as bouncing.
Bouncing in buttons has the undesirable consequence that one button press causes multiple, weaker and weaker signals. The circuitry, without any remedy, doesn't treat these signals any differently from the initial press but rather as normal signals too.
The ultimate result is that during this phase of back and forth button oscillations, the circuitry completes and then breaks, causing swift and short-lived on/off signals in connected appliances.
This sequence of fluctuations often tends to deteriorate electronic appliances or lead to falsey outcomes, and so we must rectify it.
In simple words:
As soon as we perform an action here (a button press), we get a quick burst of related actions (weaker presses), i.e. bouncing, that we do NOT want to respond to.
The remedy is to prevent the bouncing, or as it's commonly known, to implement debouncing.
Debouncing in electrical circuitry is performed using different kinds of components — RCs, Schmitt triggers, flip-flops — and ensures that only one signal ever reaches the end appliance for one button press.
Thanks to debouncing, any signals generated immediately following an initial button press are effectively canceled out.
Adaptation in software engineering
The concept of debouncing has been extended and adapted accordingly into software engineering, especially in event-driven programming, where we deal with events.
In event-driven programs, it's often the case that we have certain events that intrinsically fire at a rapid rate, for e.g. a key held down, sending rapid key-press events, or the mouse being moved, sending rapid mouse-move events, etc.
These rapid events assimilate the 'bouncing' described above in that they form something that we want to ignore for the end program.
A simple fix, i.e. debouncing, is to introduce a short delay, only a fraction of a second, during which any event is ignored. Only after this delay passes without any event firing is any subsequent event considered.
For example, if a key on the keyboard is pressed quickly twice, with an interval of 80ms in between the presses, we'll ignore the second event given that we've asserted that there must be a delay greater than 100ms in order for any subsequent event to be considered as discrete.
Debouncing in JavaScript
Debouncing certainly wasn't pioneered by JavaScript; other languages have implemented it in the past before JavaScript, especially those that deal with event-driven programming and GUI development.
But probably because of the widespread use of JavaScript, and the fact that JavaScript also works around an event-driven programming model, debouncing became a well-known concept amongst developers.
Let's consider a quick example of debouncing in action in JavaScript.
Let's say we have a search input field on a 3D modeling software's documentation page to search into the entire documentation (which is quite huge).
As soon as a character is input into this input field, the user is provided with a list of suggestions. The entered data is submitted to the server and the resulting suggestions are returned back in the response.