Virtual DOM vs Direct DOM
Why do JS Framework introduce the concept of Virtual DOM? While there are many reasons, lets focus on one reason: Separating application logic with DOM handling logic. Virtual DOM abstracts the concept of handling with DOM so that developers can focus on logic that matters for end user Let's compare between declarative method of interacting with DOM vs the imperative approach. What better way to compare with building a todo app :D This is just your normal todo app with these features: Add todo Render todo/empty state Mark done Delete todo Heres how declarative method using Virtual DOM on Add todo featur: const addTodo = (e: React.FormEvent) => { e.preventDefault(); if (input.trim()) { setTodos([ ...todos, { id: Date.now(), text: input.trim(), completed: false }, ]); setInput(""); } }; Heres an imperative way to achieve the same thing: addTodo(e) { e.preventDefault(); const text = this.input.value.trim(); if (text) { this.todos.push({ id: Date.now(), text, completed: false }); this.input.value = ""; this.saveAndRender(); // notice this method } } Whats this saveAndRender method below? Its a method that's responsible with interacting with DOM directly: saveAndRender() { localStorage.setItem("todos", JSON.stringify(this.todos)); this.render(); } render() { // Clear existing list while (this.list.firstChild) { this.list.removeChild(this.list.firstChild); } // Create and append todo elements this.todos.forEach((todo) => { // Create main container const todoDiv = document.createElement("div"); todoDiv.className = `flex items-center justify-between p-4 rounded-lg ${ todo.completed ? "bg-gray-50" : "bg-white" } border border-gray-200 shadow-sm transition-all duration-200`; // Create left section container const leftSection = document.createElement("div"); leftSection.className = "flex items-center gap-3 flex-1"; // Create toggle button const toggleButton = document.createElement("button"); toggleButton.className = `focus:outline-none transition-colors duration-200 ${ todo.completed ? "text-green-500" : "text-gray-400 hover:text-gray-500" }`; toggleButton.addEventListener("click", () => this.toggleTodo(todo.id)); // Create SVG for toggle button const toggleSvg = document.createElementNS( "http://www.w3.org/2000/svg", "svg" ); toggleSvg.setAttribute("width", "24"); toggleSvg.setAttribute("height", "24"); toggleSvg.setAttribute("viewBox", "0 0 24 24"); toggleSvg.setAttribute("fill", "none"); toggleSvg.setAttribute("stroke", "currentColor"); toggleSvg.setAttribute("stroke-width", "2"); if (todo.completed) { // Checkmark icon toggleSvg.innerHTML = ` `; } else { // X icon toggleSvg.innerHTML = ` `; } toggleButton.appendChild(toggleSvg); // Create text span const textSpan = document.createElement("span"); textSpan.className = `flex-1 text-gray-800 ${ todo.completed ? "line-through text-gray-500" : "" }`; textSpan.textContent = todo.text; // Create delete button const deleteButton = document.createElement("button"); deleteButton.className = "text-red-500 hover:text-red-600 focus:outline-none transition-colors duration-200"; deleteButton.addEventListener("click", () => this.deleteTodo(todo.id)); // Create SVG for delete button const deleteSvg = document.createElementNS( "http://www.w3.org/2000/svg", "svg" ); deleteSvg.setAttribute("width", "20"); deleteSvg.setAttribute("height", "20"); deleteSvg.setAttribute("viewBox", "0 0 24 24"); deleteSvg.setAttribute("fill", "none"); deleteSvg.setAttribute("stroke", "currentColor"); deleteSvg.setAttribute("stroke-width", "2"); deleteSvg.innerHTML = ` `; deleteButton.appendChild(deleteSvg); // Assemble the components leftSection.appendChild(toggleButton); leftSection.appendChild(textSpan); todoDiv.appendChild(leftSection); todoDiv.appendChild(deleteButton); // Add to the list this.list.appendChild(todoDiv); }); // Toggle empty state visibility this.emptyState.style.display = this.todos.length ? "none" : "block"; } You can see that on the imperative approach, there needs to be a code that handles interacting with the DOM. With Virtual DOM, this burden is lifted from the developer, they can focus on just writing application logic Besides separating application logic with DOM manipulation code, what other reasons does Virtual DOM exists? See you later on the next one
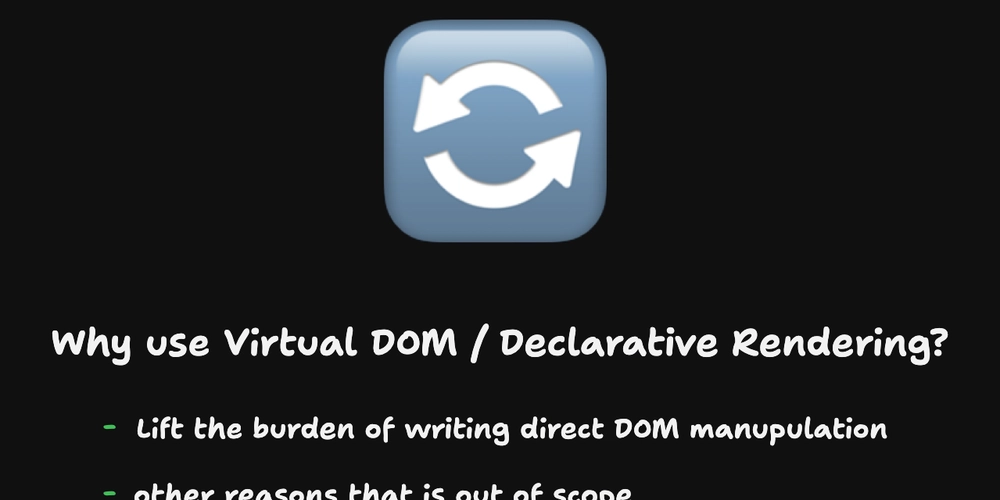
Why do JS Framework introduce the concept of Virtual DOM?
While there are many reasons, lets focus on one reason: Separating application logic with DOM handling logic. Virtual DOM abstracts the concept of handling with DOM so that developers can focus on logic that matters for end user
Let's compare between declarative method of interacting with DOM vs the imperative approach. What better way to compare with building a todo app :D
This is just your normal todo app with these features:
- Add todo
- Render todo/empty state
- Mark done
- Delete todo
Heres how declarative method using Virtual DOM on Add todo featur:
const addTodo = (e: React.FormEvent) => {
e.preventDefault();
if (input.trim()) {
setTodos([
...todos,
{ id: Date.now(), text: input.trim(), completed: false },
]);
setInput("");
}
};
Heres an imperative way to achieve the same thing:
addTodo(e) {
e.preventDefault();
const text = this.input.value.trim();
if (text) {
this.todos.push({ id: Date.now(), text, completed: false });
this.input.value = "";
this.saveAndRender(); // notice this method
}
}
Whats this saveAndRender method below? Its a method that's responsible with interacting with DOM directly:
saveAndRender() {
localStorage.setItem("todos", JSON.stringify(this.todos));
this.render();
}
render() {
// Clear existing list
while (this.list.firstChild) {
this.list.removeChild(this.list.firstChild);
}
// Create and append todo elements
this.todos.forEach((todo) => {
// Create main container
const todoDiv = document.createElement("div");
todoDiv.className = `flex items-center justify-between p-4 rounded-lg ${
todo.completed ? "bg-gray-50" : "bg-white"
} border border-gray-200 shadow-sm transition-all duration-200`;
// Create left section container
const leftSection = document.createElement("div");
leftSection.className = "flex items-center gap-3 flex-1";
// Create toggle button
const toggleButton = document.createElement("button");
toggleButton.className = `focus:outline-none transition-colors duration-200 ${
todo.completed ? "text-green-500" : "text-gray-400 hover:text-gray-500"
}`;
toggleButton.addEventListener("click", () => this.toggleTodo(todo.id));
// Create SVG for toggle button
const toggleSvg = document.createElementNS(
"http://www.w3.org/2000/svg",
"svg"
);
toggleSvg.setAttribute("width", "24");
toggleSvg.setAttribute("height", "24");
toggleSvg.setAttribute("viewBox", "0 0 24 24");
toggleSvg.setAttribute("fill", "none");
toggleSvg.setAttribute("stroke", "currentColor");
toggleSvg.setAttribute("stroke-width", "2");
if (todo.completed) {
// Checkmark icon
toggleSvg.innerHTML = `
`;
} else {
// X icon
toggleSvg.innerHTML = `
`;
}
toggleButton.appendChild(toggleSvg);
// Create text span
const textSpan = document.createElement("span");
textSpan.className = `flex-1 text-gray-800 ${
todo.completed ? "line-through text-gray-500" : ""
}`;
textSpan.textContent = todo.text;
// Create delete button
const deleteButton = document.createElement("button");
deleteButton.className =
"text-red-500 hover:text-red-600 focus:outline-none transition-colors duration-200";
deleteButton.addEventListener("click", () => this.deleteTodo(todo.id));
// Create SVG for delete button
const deleteSvg = document.createElementNS(
"http://www.w3.org/2000/svg",
"svg"
);
deleteSvg.setAttribute("width", "20");
deleteSvg.setAttribute("height", "20");
deleteSvg.setAttribute("viewBox", "0 0 24 24");
deleteSvg.setAttribute("fill", "none");
deleteSvg.setAttribute("stroke", "currentColor");
deleteSvg.setAttribute("stroke-width", "2");
deleteSvg.innerHTML = `
`;
deleteButton.appendChild(deleteSvg);
// Assemble the components
leftSection.appendChild(toggleButton);
leftSection.appendChild(textSpan);
todoDiv.appendChild(leftSection);
todoDiv.appendChild(deleteButton);
// Add to the list
this.list.appendChild(todoDiv);
});
// Toggle empty state visibility
this.emptyState.style.display = this.todos.length ? "none" : "block";
}
You can see that on the imperative approach, there needs to be a code that handles interacting with the DOM. With Virtual DOM, this burden is lifted from the developer, they can focus on just writing application logic
Besides separating application logic with DOM manipulation code, what other reasons does Virtual DOM exists?
See you later on the next one