Variables in Java
Static Variables in Java Static variables in Java are class-level variables that are shared among all instances of the class. Here are the key characteristics: Key Features Class-level storage: Belongs to the class rather than any specific instance Single copy: Only one copy exists regardless of how many objects are created Shared access: All instances share the same static variable Memory allocation: Created when the class is loaded and destroyed when the class is unloaded Declaration Syntax class MyClass { static int count; // static variable // ... } When to Use Static Variables When you need a variable common to all objects of a class For constants (usually combined with final) For maintaining counts or other shared states across instances Important Notes Access: Can be accessed using either: Class name: MyClass.count Instance reference: obj.count (not recommended) Initialization: Default values (0, null, false etc.) if not initialized Can be initialized at declaration or in static blocks Thread Safety: Static variables are not thread-safe by default - need synchronization in multi-threaded environments Example public class Employee { static int employeeCount = 0; // static variable public Employee() { employeeCount++; // increments for each new Employee created } public static void main(String[] args) { new Employee(); new Employee(); System.out.println("Total employees: " + Employee.employeeCount); // Output: 2 } } Static variables are useful for maintaining shared state across all instances of a class, but should be used judiciously to avoid unintended side effects.
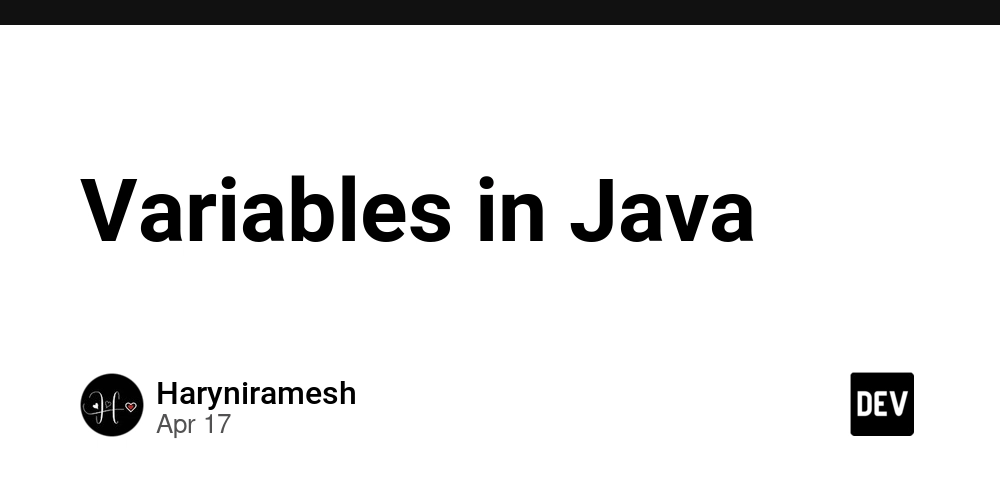
Static Variables in Java
Static variables in Java are class-level variables that are shared among all instances of the class. Here are the key characteristics:
Key Features
- Class-level storage: Belongs to the class rather than any specific instance
- Single copy: Only one copy exists regardless of how many objects are created
- Shared access: All instances share the same static variable
- Memory allocation: Created when the class is loaded and destroyed when the class is unloaded
Declaration Syntax
class MyClass {
static int count; // static variable
// ...
}
When to Use Static Variables
- When you need a variable common to all objects of a class
- For constants (usually combined with
final
) - For maintaining counts or other shared states across instances
Important Notes
-
Access: Can be accessed using either:
- Class name:
MyClass.count
- Instance reference:
obj.count
(not recommended)
- Class name:
-
Initialization:
- Default values (0, null, false etc.) if not initialized
- Can be initialized at declaration or in static blocks
Thread Safety: Static variables are not thread-safe by default - need synchronization in multi-threaded environments
Example
public class Employee {
static int employeeCount = 0; // static variable
public Employee() {
employeeCount++; // increments for each new Employee created
}
public static void main(String[] args) {
new Employee();
new Employee();
System.out.println("Total employees: " + Employee.employeeCount); // Output: 2
}
}
Static variables are useful for maintaining shared state across all instances of a class, but should be used judiciously to avoid unintended side effects.