Using Streams and Buffers in Node.js
Using Streams and Buffers in Node.js Introduction: Node.js employs streams and buffers for efficient handling of large amounts of data without loading it all into memory at once. Streams are abstract interfaces allowing sequential data processing, while buffers are used to store raw data in memory. Mastering these concepts is crucial for building scalable and performant Node.js applications. Prerequisites: Basic understanding of Node.js, JavaScript, and asynchronous programming is necessary. Features: Streams come in four types: Readable (data source), Writable (data destination), Duplex (both read and write), and Transform (modifies data). Buffers are used to hold binary data, often chunks of a stream. They provide methods for manipulation like toString() (to convert to a string) and write() (to append data). Advantages: Memory Efficiency: Streams process data in chunks, minimizing memory usage, crucial for handling large files or network streams. Improved Performance: Asynchronous operations prevent blocking, allowing concurrent processing and faster application response. Modularity: Streams are composable, enabling chaining multiple operations (e.g., reading from a file, transforming data, writing to another file). Disadvantages: Complexity: Working with streams can be more complex than traditional synchronous approaches, requiring asynchronous programming techniques. Error Handling: Asynchronous nature necessitates careful error handling using catch blocks and event listeners (like 'error' event). Debugging: Debugging stream-based applications can be more challenging compared to synchronous code. Code Snippet (Readable Stream): const fs = require('fs'); const readStream = fs.createReadStream('myFile.txt'); readStream.on('data', (chunk) => { console.log(`Received ${chunk.length} bytes`); // Process the chunk }); readStream.on('end', () => { console.log('File reading complete'); }); readStream.on('error', (err) => { console.error('Error reading file:', err); }); Conclusion: Streams and buffers are fundamental tools in Node.js for handling data efficiently. While they introduce complexities, their advantages in memory management and performance significantly outweigh these challenges for applications dealing with substantial data volumes. Mastering these concepts is essential for building robust and scalable Node.js applications.
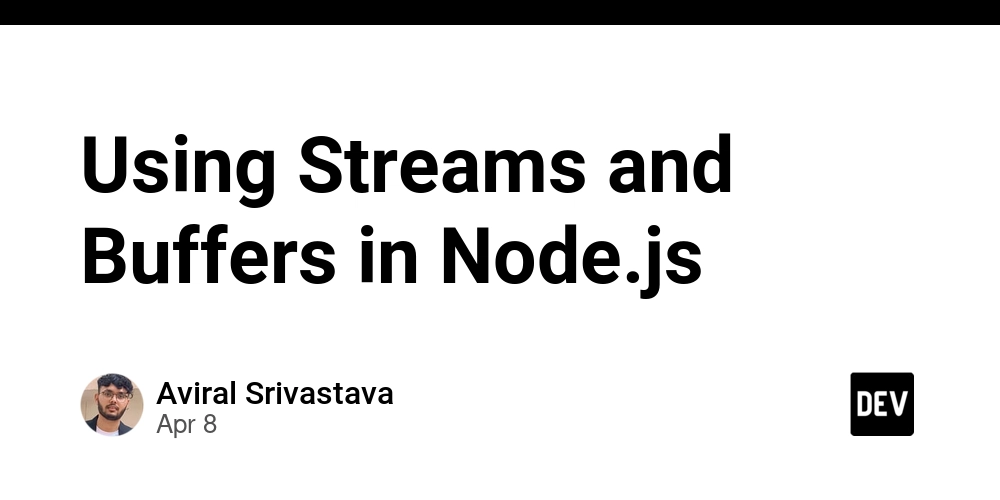
Using Streams and Buffers in Node.js
Introduction:
Node.js employs streams and buffers for efficient handling of large amounts of data without loading it all into memory at once. Streams are abstract interfaces allowing sequential data processing, while buffers are used to store raw data in memory. Mastering these concepts is crucial for building scalable and performant Node.js applications.
Prerequisites:
Basic understanding of Node.js, JavaScript, and asynchronous programming is necessary.
Features:
Streams come in four types: Readable (data source), Writable (data destination), Duplex (both read and write), and Transform (modifies data). Buffers are used to hold binary data, often chunks of a stream. They provide methods for manipulation like toString()
(to convert to a string) and write()
(to append data).
Advantages:
- Memory Efficiency: Streams process data in chunks, minimizing memory usage, crucial for handling large files or network streams.
- Improved Performance: Asynchronous operations prevent blocking, allowing concurrent processing and faster application response.
- Modularity: Streams are composable, enabling chaining multiple operations (e.g., reading from a file, transforming data, writing to another file).
Disadvantages:
- Complexity: Working with streams can be more complex than traditional synchronous approaches, requiring asynchronous programming techniques.
-
Error Handling: Asynchronous nature necessitates careful error handling using
catch
blocks and event listeners (like 'error' event). - Debugging: Debugging stream-based applications can be more challenging compared to synchronous code.
Code Snippet (Readable Stream):
const fs = require('fs');
const readStream = fs.createReadStream('myFile.txt');
readStream.on('data', (chunk) => {
console.log(`Received ${chunk.length} bytes`);
// Process the chunk
});
readStream.on('end', () => {
console.log('File reading complete');
});
readStream.on('error', (err) => {
console.error('Error reading file:', err);
});
Conclusion:
Streams and buffers are fundamental tools in Node.js for handling data efficiently. While they introduce complexities, their advantages in memory management and performance significantly outweigh these challenges for applications dealing with substantial data volumes. Mastering these concepts is essential for building robust and scalable Node.js applications.