Codestarter: another fase 3 update
I think I'm in the end run. But I made a few diversions, which I will highlight here. Extendibility In the first iteration I used environment variables to get the custom flow classes, the class cache file and some other things. For the people who didn't read the previous posts, the flow is the hook to create a custom questions flow. At some point I got the idea to use the namespace Xwero\Codestarter\Dev in the composer to get the directory. That way the custom flow classes have a standard namespace and the users of the application can choose the directory. An extra benefit is that the directory can contain other files, like config.php The current default file structure is: dev/ ├── Flows/ │ ├── Php/ │ │ ├── TypeFlow.php │ │ ├── config.php │ ├── Templates/ │ │ ├── config.php My initial thought was to have a list with all the types. But that could cause name conflicts very fast. The first option/question is now what is the group the file belongs to. I also decided the flow should always be called TypeFlow.php. Like using a default namespace this makes it easier to discover the classes. For multiple php flows I check the Php/Templates/CustomName child directories. protected function getGroupCustomFlowClasses(string $group): array { $classes = []; if (file_exists($this->devPath . "/Flows/$group/TypeFlow.php")) { $classes[] = "Xwero\Codestarter\Flows\\$group\TypeFlow"; } foreach(glob($this->devPath . "/Flows/$group/*/TypeFlow.php") as $file) { $parent = str_replace(dirname($file, 2), "", dirname($file)); $classes[] = "Xwero\Codestarter\Flows\\$group\$parent\TypeFlow"; } return $classes; } The config files are separated because the it is very likely the content will differ from group to group. A smaller extendibility change I made is to add the following to the command. // in configure method $this->addArgument('other', InputArgument::IS_ARRAY); // in execute method $extraOptions = array_diff(array_slice($GLOBALS['argv'], array_search('--', $GLOBALS['argv'])+1), ['--to-cli']); I got the idea to create a custom flow with the Symfony or Laravel commands that takes the options from the cli. A command can now look like bin/codestarter -- model Flight --controller --resource --requests. Everything after -- will be in the $extraOptions variable, that is a part of the flow generate method arguments. Templates In my previous post I only had the intention to generate other files. But because I had to rework the AbstractFlow class to separate the PHP specific methods. I figured it would not take long to add the templates type. class TypeFlow extends AbstractFlow { public array $types = ['twig', 'blade']; public function generate(GenerateDTO $props): NewFileDTO { return $this->{$props->type . 'Flow'}(); } protected function twigFlow(): NewFileDTO { return new NewFileDTO('',''); } protected function bladeFlow(): NewFileDTO { return new NewFileDTO('',''); } } For now the class is very empty. Once I fill the twigFlow and bladeFlow methods the types will be usable. Next tasks I'm still writing tests. But the tests I already wrote are helping me to make the changes faster and with more confidence. I'm also busy with the code that is needed to have the application as a package.
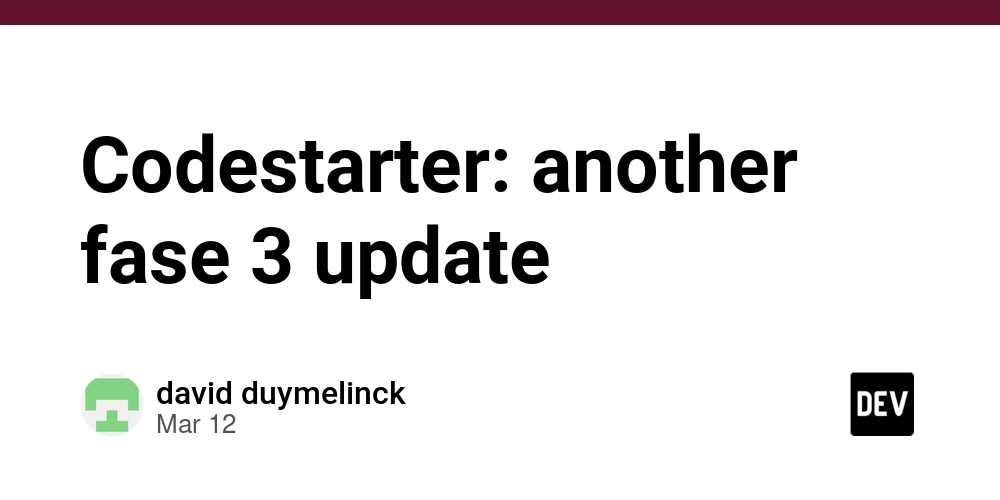
I think I'm in the end run. But I made a few diversions, which I will highlight here.
Extendibility
In the first iteration I used environment variables to get the custom flow classes, the class cache file and some other things.
For the people who didn't read the previous posts, the flow is the hook to create a custom questions flow.
At some point I got the idea to use the namespace Xwero\Codestarter\Dev in the composer to get the directory. That way the custom flow classes have a standard namespace and the users of the application can choose the directory.
An extra benefit is that the directory can contain other files, like config.php
The current default file structure is:
dev/
├── Flows/
│ ├── Php/
│ │ ├── TypeFlow.php
│ │ ├── config.php
│ ├── Templates/
│ │ ├── config.php
My initial thought was to have a list with all the types. But that could cause name conflicts very fast. The first option/question is now what is the group the file belongs to.
I also decided the flow should always be called TypeFlow.php. Like using a default namespace this makes it easier to discover the classes.
For multiple php flows I check the Php/Templates/CustomName child directories.
protected function getGroupCustomFlowClasses(string $group): array
{
$classes = [];
if (file_exists($this->devPath . "/Flows/$group/TypeFlow.php")) {
$classes[] = "Xwero\Codestarter\Flows\\$group\TypeFlow";
}
foreach(glob($this->devPath . "/Flows/$group/*/TypeFlow.php") as $file) {
$parent = str_replace(dirname($file, 2), "", dirname($file));
$classes[] = "Xwero\Codestarter\Flows\\$group\$parent\TypeFlow";
}
return $classes;
}
The config files are separated because the it is very likely the content will differ from group to group.
A smaller extendibility change I made is to add the following to the command.
// in configure method
$this->addArgument('other', InputArgument::IS_ARRAY);
// in execute method
$extraOptions = array_diff(array_slice($GLOBALS['argv'], array_search('--', $GLOBALS['argv'])+1), ['--to-cli']);
I got the idea to create a custom flow with the Symfony or Laravel commands that takes the options from the cli. A command can now look like bin/codestarter -- model Flight --controller --resource --requests
. Everything after -- will be in the $extraOptions
variable, that is a part of the flow generate
method arguments.
Templates
In my previous post I only had the intention to generate other files. But because I had to rework the AbstractFlow
class to separate the PHP specific methods. I figured it would not take long to add the templates type.
class TypeFlow extends AbstractFlow
{
public array $types = ['twig', 'blade'];
public function generate(GenerateDTO $props): NewFileDTO
{
return $this->{$props->type . 'Flow'}();
}
protected function twigFlow(): NewFileDTO
{
return new NewFileDTO('','');
}
protected function bladeFlow(): NewFileDTO
{
return new NewFileDTO('','');
}
}
For now the class is very empty. Once I fill the twigFlow
and bladeFlow
methods the types will be usable.
Next tasks
I'm still writing tests. But the tests I already wrote are helping me to make the changes faster and with more confidence.
I'm also busy with the code that is needed to have the application as a package.