Using Laravel Event Listeners for Cleaner, Decoupled Code
As your application grows, it's common to see controllers doing too much — saving data, sending emails, updating reports… all in the same place. That makes your code harder to maintain and reuse. A simple and powerful way to fix this is by using Events and Listeners in Laravel. Why use Events and Listeners? Events allow you to decouple secondary actions from your main logic. Instead of calling everything derectly in the controller, you trigger an event, and listeners handle the rest. Basic Structure php artisan make:event OrderPlaced php artisan make:listener SendConfirmationEmail Laravel will create two classes: one for the event and one for the listener. Example Order Placed class OrderPlaced { public function __construct( public Order $order ) {} } Listener: SendConfirmationEmail class SendConfirmationEmail { public function handle(OrderPlaced $event) { Mail::to($event->order->customer->email) ->send(new OrderConfirmationEmail($event->order)); } } Triggering the Event Inside a controller or service: event(new OrderPlaced($order)); Benefits Decoupling: each listener does only one job. Reusability: you can reuse listeners in different places. Scalability: add new listeners without changing existing code. Bonus: Async Events If you want the listener to run in the background (for example, to send emails or make API calls): class SendConfirmationEmail implements ShouldQueue { use InteractsWithQueue, Queueable; } Conclusion Using Event Listeners in Laravel is a great way to keep your code clean, organized, and scalable. Start with simple use cases like sending emails or logging, and grow from there. Are you already using events in your Laravel projects? What’s the biggest benefit you’ve seen?
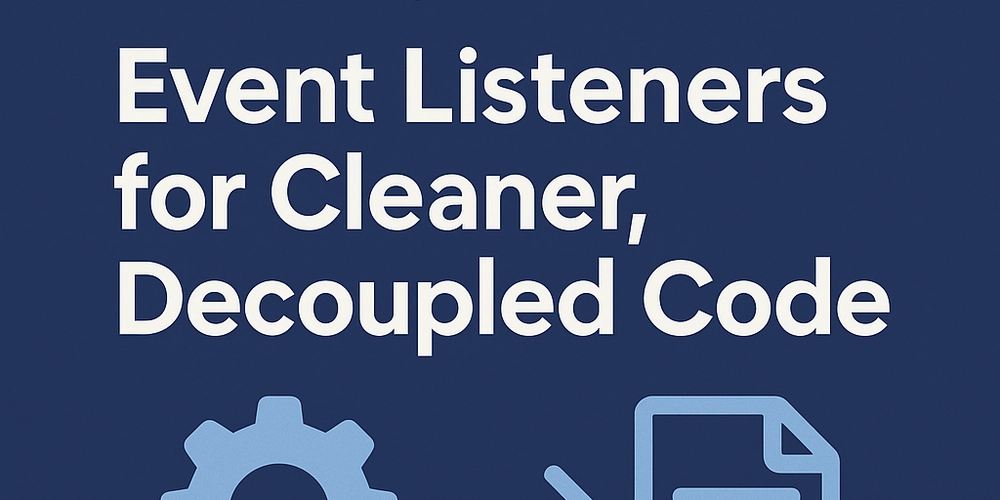
As your application grows, it's common to see controllers doing too much — saving data, sending emails, updating reports… all in the same place. That makes your code harder to maintain and reuse.
A simple and powerful way to fix this is by using Events and Listeners in Laravel.
Why use Events and Listeners?
Events allow you to decouple secondary actions from your main logic. Instead of calling everything derectly in the controller, you trigger an event, and listeners handle the rest.
Basic Structure
php artisan make:event OrderPlaced
php artisan make:listener SendConfirmationEmail
Laravel will create two classes: one for the event and one for the listener.
Example
Order Placed
class OrderPlaced
{
public function __construct(
public Order $order
) {}
}
Listener: SendConfirmationEmail
class SendConfirmationEmail
{
public function handle(OrderPlaced $event)
{
Mail::to($event->order->customer->email)
->send(new OrderConfirmationEmail($event->order));
}
}
Triggering the Event
Inside a controller or service:
event(new OrderPlaced($order));
Benefits
Decoupling: each listener does only one job.
Reusability: you can reuse listeners in different places.
Scalability: add new listeners without changing existing code.
Bonus: Async Events
If you want the listener to run in the background (for example, to send emails or make API calls):
class SendConfirmationEmail implements ShouldQueue
{
use InteractsWithQueue, Queueable;
}
Conclusion
Using Event Listeners in Laravel is a great way to keep your code clean, organized, and scalable. Start with simple use cases like sending emails or logging, and grow from there.
Are you already using events in your Laravel projects? What’s the biggest benefit you’ve seen?