Unveiling: How to Build Elegant API Interfaces with Gin Framework
From Repetitive Code to Elegant Encapsulation In Gin framework development, have you ever encountered this scenario: each API endpoint requires repetitive code for parameter binding, error handling, and response formatting? This not only increases code volume but also reduces development efficiency and code maintainability. Let's look at a typical example: func getUser(ctx *gin.Context) { var in UserInput if err := ctx.ShouldBindQuery(&in); err != nil { ctx.JSON(400, gin.H{"error": err.Error()}) return } user, err := userService.GetUser(in.ID) if err != nil { ctx.JSON(500, gin.H{"error": "Server error"}) return } ctx.JSON(200, user) } This code pattern repeatedly appears in projects, leading to: Large amounts of boilerplate code Scattered error handling logic Inconsistent response formats Business logic buried in technical details This article will introduce how to solve these problems through the elegant encapsulation solution of web.WarpH. Elegant Solution web.WarpH is a generic-based encapsulation function that solves the above problems through: Automatic parameter binding Unified error handling Standardized response formatting Allowing developers to focus on business logic Core Implementation web.WarpH's implementation is based on the generic feature introduced in Go 1.18, using type parameters I and O to represent input and output types respectively: func WarpH[I any, O any](fn func(*gin.Context, *I) (O, error)) gin.HandlerFunc { return func(c *gin.Context) { var in I if unsafe.Sizeof(in) != 0 { switch c.Request.Method { case http.MethodGet: if err := c.ShouldBindQuery(&in); err != nil { Fail(c, ErrBadRequest.With(HanddleJSONErr(err).Error())) return } case http.MethodPost, http.MethodPut, http.MethodDelete, http.MethodPatch: if c.Request.ContentLength > 0 { if err := c.ShouldBindJSON(&in); err != nil { Fail(c, ErrBadRequest.With(HanddleJSONErr(err).Error())) return } } } } out, err := fn(c, &in) if err != nil { Fail(c, err) return } Success(c, out) } } Key points of this implementation: Using generics to support arbitrary input and output types Automatically selecting parameter binding methods based on request method Unified error handling and response formatting Skipping binding for zero-value parameters Usage Example With web.WarpH, the code becomes exceptionally concise: func getUser(ctx *gin.Context, in *UserInput) (*UserOutput, error) { return userService.GetUser(in.ID) } Advantage Analysis web.WarpH allows developers to focus on business logic implementation rather than repetitive boilerplate code. Through generics and unified error handling mechanisms, it achieves code conciseness and maintainability. At compile time, generics ensure type safety, prevent runtime type errors, and provide better IDE support. Practical Application Scenarios Scenario 1: Query Single User // Route definition router.GET("/users/:id", web.WarpH(getUser)) // Handler function, using `*struct{}` empty value to avoid parameter binding func getUser(ctx *gin.Context, in *struct{}) (*UserOutput, error) { id := ctx.Param("id") return userService.GetUser(id) } This scenario demonstrates how to handle GET requests without request parameters, using ctx.Param to get path parameters. Scenario 2: Query User List // Route definition router.GET("/users", web.WarpH(listUsers)) // Request parameters (defined in user package) type FindUsersInput struct { Page int `form:"page"` Size int `form:"size"` Name string `form:"name"` } // Handler function func findUsers(ctx *gin.Context, in *FindUsersInput) (*FindUsersOutput, error) { return userService.ListUsers(in) } This scenario demonstrates how to use form tags to handle query parameters. Scenario 3: Update User Information // Route definition router.PUT("/users/:id", web.WarpH(updateUser)) // Request parameters type UpdateUserInput struct { Name string `json:"name"` Email string `json:"email"` Age int `json:"age"` } // Handler function func updateUser(ctx *gin.Context, in *UpdateUserInput) (*UserOutput, error) { id := ctx.Param("id") return userService.UpdateUser(in, id) } This scenario demonstrates how to handle PUT requests with both path parameters and request body parameters. Scenario 4: Delete User // Route definition router.DELETE("/users/:id", web.WarpH(deleteUser)) // Handler function func deleteUser(ctx *gin.Context, in *struct{}) (any, error) { id :
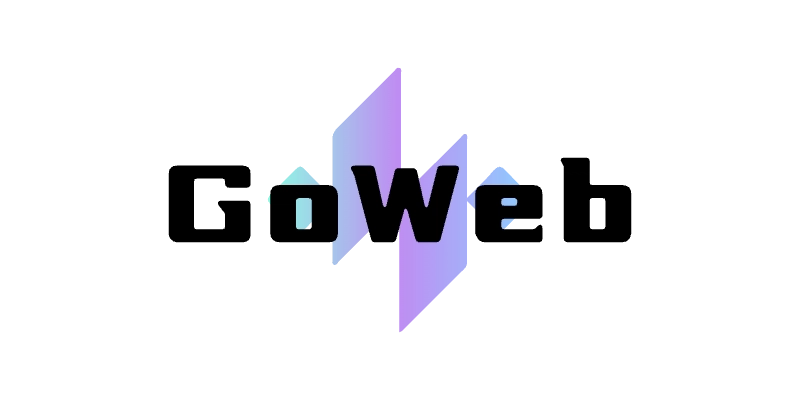
From Repetitive Code to Elegant Encapsulation
In Gin framework development, have you ever encountered this scenario: each API endpoint requires repetitive code for parameter binding, error handling, and response formatting? This not only increases code volume but also reduces development efficiency and code maintainability.
Let's look at a typical example:
func getUser(ctx *gin.Context) {
var in UserInput
if err := ctx.ShouldBindQuery(&in); err != nil {
ctx.JSON(400, gin.H{"error": err.Error()})
return
}
user, err := userService.GetUser(in.ID)
if err != nil {
ctx.JSON(500, gin.H{"error": "Server error"})
return
}
ctx.JSON(200, user)
}
This code pattern repeatedly appears in projects, leading to:
- Large amounts of boilerplate code
- Scattered error handling logic
- Inconsistent response formats
- Business logic buried in technical details
This article will introduce how to solve these problems through the elegant encapsulation solution of web.WarpH
.
Elegant Solution
web.WarpH
is a generic-based encapsulation function that solves the above problems through:
- Automatic parameter binding
- Unified error handling
- Standardized response formatting
- Allowing developers to focus on business logic
Core Implementation
web.WarpH
's implementation is based on the generic feature introduced in Go 1.18, using type parameters I
and O
to represent input and output types respectively:
func WarpH[I any, O any](fn func(*gin.Context, *I) (O, error)) gin.HandlerFunc {
return func(c *gin.Context) {
var in I
if unsafe.Sizeof(in) != 0 {
switch c.Request.Method {
case http.MethodGet:
if err := c.ShouldBindQuery(&in); err != nil {
Fail(c, ErrBadRequest.With(HanddleJSONErr(err).Error()))
return
}
case http.MethodPost, http.MethodPut, http.MethodDelete, http.MethodPatch:
if c.Request.ContentLength > 0 {
if err := c.ShouldBindJSON(&in); err != nil {
Fail(c, ErrBadRequest.With(HanddleJSONErr(err).Error()))
return
}
}
}
}
out, err := fn(c, &in)
if err != nil {
Fail(c, err)
return
}
Success(c, out)
}
}
Key points of this implementation:
- Using generics to support arbitrary input and output types
- Automatically selecting parameter binding methods based on request method
- Unified error handling and response formatting
- Skipping binding for zero-value parameters
Usage Example
With web.WarpH
, the code becomes exceptionally concise:
func getUser(ctx *gin.Context, in *UserInput) (*UserOutput, error) {
return userService.GetUser(in.ID)
}
Advantage Analysis
web.WarpH
allows developers to focus on business logic implementation rather than repetitive boilerplate code. Through generics and unified error handling mechanisms, it achieves code conciseness and maintainability. At compile time, generics ensure type safety, prevent runtime type errors, and provide better IDE support.
Practical Application Scenarios
Scenario 1: Query Single User
// Route definition
router.GET("/users/:id", web.WarpH(getUser))
// Handler function, using `*struct{}` empty value to avoid parameter binding
func getUser(ctx *gin.Context, in *struct{}) (*UserOutput, error) {
id := ctx.Param("id")
return userService.GetUser(id)
}
This scenario demonstrates how to handle GET requests without request parameters, using ctx.Param
to get path parameters.
Scenario 2: Query User List
// Route definition
router.GET("/users", web.WarpH(listUsers))
// Request parameters (defined in user package)
type FindUsersInput struct {
Page int `form:"page"`
Size int `form:"size"`
Name string `form:"name"`
}
// Handler function
func findUsers(ctx *gin.Context, in *FindUsersInput) (*FindUsersOutput, error) {
return userService.ListUsers(in)
}
This scenario demonstrates how to use form
tags to handle query parameters.
Scenario 3: Update User Information
// Route definition
router.PUT("/users/:id", web.WarpH(updateUser))
// Request parameters
type UpdateUserInput struct {
Name string `json:"name"`
Email string `json:"email"`
Age int `json:"age"`
}
// Handler function
func updateUser(ctx *gin.Context, in *UpdateUserInput) (*UserOutput, error) {
id := ctx.Param("id")
return userService.UpdateUser(in, id)
}
This scenario demonstrates how to handle PUT requests with both path parameters and request body parameters.
Scenario 4: Delete User
// Route definition
router.DELETE("/users/:id", web.WarpH(deleteUser))
// Handler function
func deleteUser(ctx *gin.Context, in *struct{}) (any, error) {
id := ctx.Param("id")
return userService.DeleteUser(id)
}
This scenario demonstrates how to handle DELETE requests and handle cases with no return value.
For complex scenarios like file downloads or non-CRUD operations, you can use gin.HandlerFunc
instead of web.WarpH
.
Best Practices
- Use structs to define clear input and output parameters, improving code readability
- Use tags appropriately, such as
json
,form
, etc. - Add necessary parameter validation
- Use pointer types to avoid unnecessary memory allocation
- Design APIs following RESTful style
Summary
By using web.WarpH
, we can:
- Significantly reduce repetitive code
- Improve code maintainability
- Unify error handling
- Enhance development efficiency
This encapsulation approach is particularly suitable for team collaboration, helping teams quickly build high-quality API services.
About goddd
The web.WarpH
introduced in this article is a core component of the goddd project. goddd is a Go project based on DDD (Domain-Driven Design) principles, providing a series of tools and best practices to help developers build maintainable and extensible applications.
If you're interested in the content introduced in this article, welcome to visit the goddd project for more details. The project provides complete example code and detailed documentation to help you get started quickly.