Understanding State Updates in React with a Simple Marble Game.
The Game Setup Imagine you're playing a game where you have a bag of marbles. Each time it's your turn, you can add one more marble to your bag. Let's compare this to a React component where you have a state variable called slider, representing the number of marbles in your bag. Scenario 1: Direct State Update When you use the state update like setSlider(slider + 1), it’s similar to looking into your bag, counting the marbles you have, say five, and then deciding you will have six marbles next. You then pass this message along to a friend who is responsible for updating the marble count. If you repeat this command multiple times quickly, without waiting for confirmation from your friend, you always start from the last counted number, which was five. This means, even if you issue the update three times in a row, you might still end up thinking you should have six marbles because your friend didn’t have time to update the count between your commands. Scenario 2: Functional State Update On the other hand, using setSlider(slider => slider + 1) is like telling your friend, Please check how many marbles are currently in the bag and add one more. In this case, you don’t do the counting; your friend does. Your friend checks the current count right before adding a new marble, ensuring each addition is based on the most recent count.This method is particularly useful in React because it handles rapid or multiple state updates based on the most current state value. It ensures that every update is accurate, no matter how quickly they are fired off. Why This Matters In web development, especially when using React, components might update frequently, and state values can change rapidly. By using the functional update form slider => slider + 1, you ensure that each update is dependent on the most recent state. This is crucial for avoiding bugs where your UI might not reflect the correct state due to outdated values. Conclusion Understanding the difference between direct and functional state updates can greatly enhance your ability to manage state in React effectively. It helps prevent bugs and ensures that your application behaves as expected, even under rapid state changes. This simple marble game analogy helps illustrate why updating state functionally can be a better choice in many scenarios, ensuring your application remains robust and reliable.
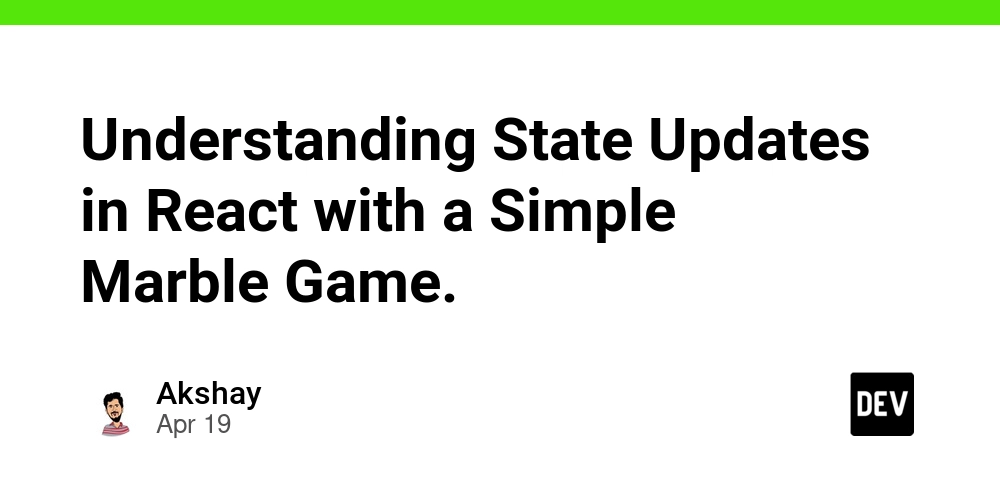
The Game Setup
Imagine you're playing a game where you have a bag of marbles. Each time it's your turn, you can add one more marble to your bag. Let's compare this to a React component where you have a state variable called slider, representing the number of marbles in your bag.
Scenario 1: Direct State Update
When you use the state update like setSlider(slider + 1)
, it’s similar to looking into your bag, counting the marbles you have, say five, and then deciding you will have six marbles next. You then pass this message along to a friend who is responsible for updating the marble count. If you repeat this command multiple times quickly, without waiting for confirmation from your friend, you always start from the last counted number, which was five. This means, even if you issue the update three times in a row, you might still end up thinking you should have six marbles because your friend didn’t have time to update the count between your commands.
Scenario 2: Functional State Update
On the other hand, using setSlider(slider => slider + 1)
is like telling your friend, Please check how many marbles are currently in the bag and add one more. In this case, you don’t do the counting; your friend does. Your friend checks the current count right before adding a new marble, ensuring each addition is based on the most recent count.This method is particularly useful in React because it handles rapid or multiple state updates based on the most current state value. It ensures that every update is accurate, no matter how quickly they are fired off.
Why This Matters
In web development, especially when using React, components might update frequently, and state values can change rapidly. By using the functional update form slider => slider + 1
, you ensure that each update is dependent on the most recent state. This is crucial for avoiding bugs where your UI might not reflect the correct state due to outdated values.
Conclusion
Understanding the difference between direct and functional state updates can greatly enhance your ability to manage state in React effectively. It helps prevent bugs and ensures that your application behaves as expected, even under rapid state changes. This simple marble game analogy helps illustrate why updating state functionally can be a better choice in many scenarios, ensuring your application remains robust and reliable.