React Native Debugging Tools (2025)
Introduction Debugging React Native applications can be challenging due to the hybrid nature of mobile development—bridging JavaScript and native code. However, with the right tools, developers can efficiently diagnose issues, optimize performance, and streamline their workflow. In this guide, we’ll explore the best React Native debugging tools in 2025, their key features, setup processes, and best practices for effective debugging. 1. Flipper: Meta’s Advanced Debugging Platform What is Flipper? Flipper is a powerful desktop debugging tool developed by Meta (formerly Facebook) for inspecting iOS and Android apps. It supports React Native through a plugin-based architecture, enabling real-time debugging across multiple aspects of an application. Key Features Layout Inspector: Visualize component hierarchies. Network Inspector: Monitor API requests and responses. Database Browser: View and modify local storage (SQLite, AsyncStorage). Log Viewer: Centralized logging for JavaScript and native logs. React DevTools Integration: Inspect React component trees and hooks. Setup Guide Install Flipper from fbflipper.com Add the React Native plugin: npm install react-native-flipper For Android, update android/app/build.gradle: debugImplementation("com.facebook.flipper:flipper:0.182.0") { exclude group: 'com.facebook.fbjni' } debugImplementation("com.facebook.flipper:flipper-network-plugin:0.182.0") Ensure debuggability in debug/AndroidManifest.xml (never in release builds): For iOS, run: cd ios && pod install Common Issues & Fixes Connection Problems: Ensure both device and machine are on the same network. Plugin Mismatch: Keep Flipper and plugin versions in sync. Slow Performance: Disable unused plugins (e.g., remove flipper-network-plugin if unused). 2. React Native Debugger (All-in-One Solution) Why Use It? This standalone tool combines: React DevTools (component inspection) Redux DevTools (state management) JavaScript Debugger (breakpoints, console) Installation brew install --cask react-native-debugger # macOS # Or download from https://github.com/jhen0409/react-native-debugger/releases Key Features Time-travel debugging for Redux. Network request inspection. Performance monitoring (FPS, memory usage). Pro Tip Best for Redux/MobX apps. Configure it as your default debugger in package.json: "scripts": { "start:debug": "REACT_DEBUGGER='react-native-debugger' react-native start" } 3. Chrome Developer Tools (Quick & Reliable) How to Use Start Metro bundler: npx react-native start Press d in the terminal and select Debug in Chrome. Features JavaScript debugging with breakpoints. Console logging (console.log, console.error). Network request inspection. Limitations Slower than Hermes debugger. Not ideal for native debugging. 4. Reactotron (State & API Debugging) Best For Redux/MobX state inspection. API request/response tracking. Setup npm install reactotron-react-native Configure in your app: import Reactotron from 'reactotron-react-native'; Reactotron .configure() .useReactNative() .connect(); 5. Hermes Debugger (Optimized for Performance) Why Hermes? Faster startup and execution. Direct debugging without Chrome. Setup Enable Hermes in android/app/build.gradle: project.ext.react = [ enableHermes: true ] // RN 0.70+ For iOS, edit ios/Podfile: use_react_native!(hermes_enabled: true) Debug via Flipper or chrome://inspect (Hermes engine). Comparison Table: Choosing the Right Tool Tool Best For Performance Impact Setup Complexity Flipper Comprehensive debugging Medium Medium RN Debugger Redux & React DevTools Low Low Chrome DevTools Quick JS debugging High Very Low Reactotron State & API inspection Low Medium Hermes Debugger Production-like debugging Very Low High Best Practices for Debugging in 2025 Disable debug tools in production (use conditional imports). Standardize tools across the team for consistency. Profile regularly, not just when issues arise. Combine native & JS debugging (Xcode/Android Studio + Flipper). Conclusion React Native’s debugging ecosystem in 2025 offers powerful tools for every scenario—whether you need deep native inspection (Flipper), state management debugging (Reactotron), or performance profiling (Hermes). By mastering these tools, developers can reduce debugging time, improve app stability, and deliver smoother user experiences.
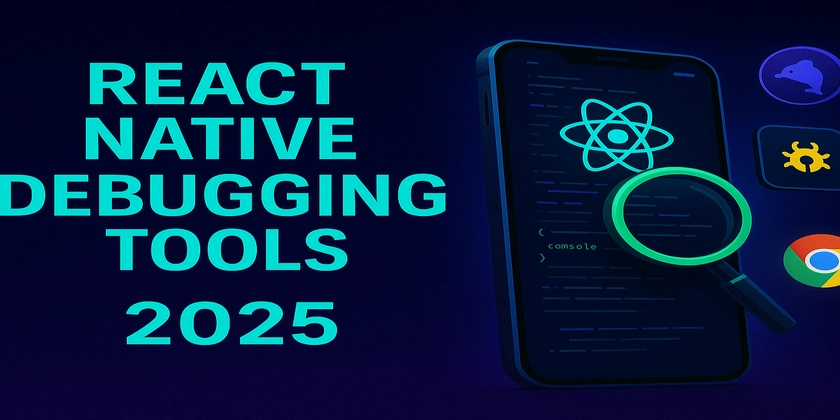
Introduction
Debugging React Native applications can be challenging due to the hybrid nature of mobile development—bridging JavaScript and native code. However, with the right tools, developers can efficiently diagnose issues, optimize performance, and streamline their workflow.
In this guide, we’ll explore the best React Native debugging tools in 2025, their key features, setup processes, and best practices for effective debugging.
1. Flipper: Meta’s Advanced Debugging Platform
What is Flipper?
Flipper is a powerful desktop debugging tool developed by Meta (formerly Facebook) for inspecting iOS and Android apps. It supports React Native through a plugin-based architecture, enabling real-time debugging across multiple aspects of an application.
Key Features
- Layout Inspector: Visualize component hierarchies.
- Network Inspector: Monitor API requests and responses.
- Database Browser: View and modify local storage (SQLite, AsyncStorage).
- Log Viewer: Centralized logging for JavaScript and native logs.
- React DevTools Integration: Inspect React component trees and hooks.
Setup Guide
Install Flipper from fbflipper.com
Add the React Native plugin:
npm install react-native-flipper
- For Android, update android/app/build.gradle:
debugImplementation("com.facebook.flipper:flipper:0.182.0") {
exclude group: 'com.facebook.fbjni'
}
debugImplementation("com.facebook.flipper:flipper-network-plugin:0.182.0")
- Ensure debuggability in debug/AndroidManifest.xml (never in release builds):
android:debuggable="true" ... />
- For iOS, run:
cd ios && pod install
Common Issues & Fixes
- Connection Problems: Ensure both device and machine are on the same network.
- Plugin Mismatch: Keep Flipper and plugin versions in sync.
-
Slow Performance: Disable unused plugins (e.g., remove
flipper-network-plugin
if unused).
2. React Native Debugger (All-in-One Solution)
Why Use It?
This standalone tool combines:
- React DevTools (component inspection)
- Redux DevTools (state management)
- JavaScript Debugger (breakpoints, console)
Installation
brew install --cask react-native-debugger # macOS
# Or download from https://github.com/jhen0409/react-native-debugger/releases
Key Features
- Time-travel debugging for Redux.
- Network request inspection.
- Performance monitoring (FPS, memory usage).
Pro Tip
Best for Redux/MobX apps. Configure it as your default debugger in package.json:
"scripts": {
"start:debug": "REACT_DEBUGGER='react-native-debugger' react-native start"
}
3. Chrome Developer Tools (Quick & Reliable)
How to Use
- Start Metro bundler:
npx react-native start
- Press d in the terminal and select Debug in Chrome.
Features
- JavaScript debugging with breakpoints.
- Console logging (
console.log
,console.error
). - Network request inspection.
Limitations
- Slower than Hermes debugger.
- Not ideal for native debugging.
4. Reactotron (State & API Debugging)
Best For
- Redux/MobX state inspection.
- API request/response tracking.
Setup
npm install reactotron-react-native
Configure in your app:
import Reactotron from 'reactotron-react-native';
Reactotron
.configure()
.useReactNative()
.connect();
5. Hermes Debugger (Optimized for Performance)
Why Hermes?
- Faster startup and execution.
- Direct debugging without Chrome.
Setup
- Enable Hermes in android/app/build.gradle:
project.ext.react = [ enableHermes: true ] // RN 0.70+
- For iOS, edit ios/Podfile:
use_react_native!(hermes_enabled: true)
- Debug via Flipper or chrome://inspect (Hermes engine).
Comparison Table: Choosing the Right Tool
Tool | Best For | Performance Impact | Setup Complexity |
---|---|---|---|
Flipper | Comprehensive debugging | Medium | Medium |
RN Debugger | Redux & React DevTools | Low | Low |
Chrome DevTools | Quick JS debugging | High | Very Low |
Reactotron | State & API inspection | Low | Medium |
Hermes Debugger | Production-like debugging | Very Low | High |
Best Practices for Debugging in 2025
- Disable debug tools in production (use conditional imports).
- Standardize tools across the team for consistency.
- Profile regularly, not just when issues arise.
- Combine native & JS debugging (Xcode/Android Studio + Flipper).
Conclusion
React Native’s debugging ecosystem in 2025 offers powerful tools for every scenario—whether you need deep native inspection (Flipper), state management debugging (Reactotron), or performance profiling (Hermes).
By mastering these tools, developers can reduce debugging time, improve app stability, and deliver smoother user experiences.