Understanding Higher-Order Functions in Go
What is a Higher-Order Function? A Higher-Order Function (HOF) is a function that either takes another function as an argument or returns a function as its result. This makes functions in Go more flexible and powerful by enabling concepts like callbacks, function composition, and functional programming patterns. Characteristics of Higher-Order Functions ✅ Takes a function as an argument ✅ Returns a function as a result ✅ Enhances code reusability and modularity ✅ Common in functional programming patterns Examples of Higher-Order Functions in Go 1️⃣ Passing a Function as an Argument A function that accepts another function as a parameter and applies it to two integers. package main import "fmt" // Higher-order function: Takes a function as an argument func operate(a int, b int, op func(int, int) int) int { return op(a, b) } // Function to add two numbers func add(x, y int) int { return x + y } func main() { result := operate(4, 5, add) fmt.Println("Result:", result) // Output: Result: 9 } ✅ Why Higher-Order? operate takes another function (op) as an argument. This makes operate flexible, as it can apply different functions like add, subtract, multiply, etc. 2️⃣ Returning a Function from Another Function A function that returns another function instead of a direct value. package main import "fmt" // Higher-order function: Returns a function func multiplier(factor int) func(int) int { return func(n int) int { return n * factor } } func main() { double := multiplier(2) // double now holds a function that multiplies by 2 fmt.Println(double(5)) // Output: 10 } ✅ Why Higher-Order? multiplier(2) returns a function that multiplies any number by 2. The returned function is stored in double, which can be used later. 3️⃣ Using Anonymous Functions in Higher-Order Functions Instead of defining a separate function, we can pass an anonymous function directly. package main import "fmt" func operate(a int, b int, op func(int, int) int) int { return op(a, b) } func main() { result := operate(10, 3, func(x, y int) int { return x - y }) fmt.Println("Result:", result) // Output: Result: 7 } ✅ Why Higher-Order? The operate function takes an anonymous function as a parameter. No need to define a separate function like subtract. First-Order vs. Higher-Order Functions Feature First-Order Function Higher-Order Function Accepts another function? ❌ No ✅ Yes Returns another function? ❌ No ✅ Yes Example sumArray([]int) operate(4, 2, func(x, y int) int { return x * y }) When to Use Higher-Order Functions? ✅ When writing generic, reusable functions ✅ When using callbacks or function composition ✅ When implementing custom sorting, filtering, or mapping operations ✅ When applying functional programming patterns
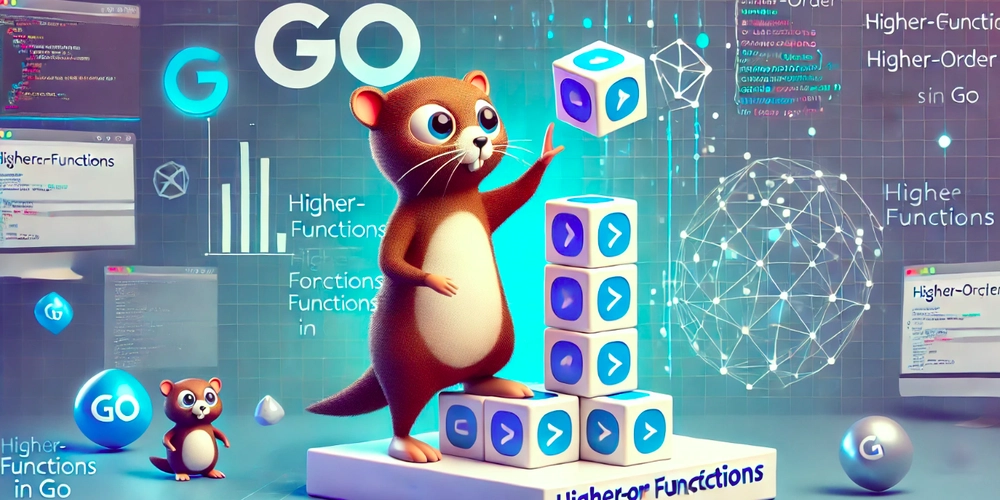
What is a Higher-Order Function?
A Higher-Order Function (HOF) is a function that either takes another function as an argument or returns a function as its result.
This makes functions in Go more flexible and powerful by enabling concepts like callbacks, function composition, and functional programming patterns.
Characteristics of Higher-Order Functions
✅ Takes a function as an argument
✅ Returns a function as a result
✅ Enhances code reusability and modularity
✅ Common in functional programming patterns
Examples of Higher-Order Functions in Go
1️⃣ Passing a Function as an Argument
A function that accepts another function as a parameter and applies it to two integers.
package main
import "fmt"
// Higher-order function: Takes a function as an argument
func operate(a int, b int, op func(int, int) int) int {
return op(a, b)
}
// Function to add two numbers
func add(x, y int) int {
return x + y
}
func main() {
result := operate(4, 5, add)
fmt.Println("Result:", result) // Output: Result: 9
}
✅ Why Higher-Order?
-
operate
takes another function (op
) as an argument. - This makes
operate
flexible, as it can apply different functions likeadd
,subtract
,multiply
, etc.
2️⃣ Returning a Function from Another Function
A function that returns another function instead of a direct value.
package main
import "fmt"
// Higher-order function: Returns a function
func multiplier(factor int) func(int) int {
return func(n int) int {
return n * factor
}
}
func main() {
double := multiplier(2) // double now holds a function that multiplies by 2
fmt.Println(double(5)) // Output: 10
}
✅ Why Higher-Order?
-
multiplier(2)
returns a function that multiplies any number by 2. - The returned function is stored in
double
, which can be used later.
3️⃣ Using Anonymous Functions in Higher-Order Functions
Instead of defining a separate function, we can pass an anonymous function directly.
package main
import "fmt"
func operate(a int, b int, op func(int, int) int) int {
return op(a, b)
}
func main() {
result := operate(10, 3, func(x, y int) int {
return x - y
})
fmt.Println("Result:", result) // Output: Result: 7
}
✅ Why Higher-Order?
- The
operate
function takes an anonymous function as a parameter. - No need to define a separate function like
subtract
.
First-Order vs. Higher-Order Functions
Feature | First-Order Function | Higher-Order Function |
---|---|---|
Accepts another function? | ❌ No | ✅ Yes |
Returns another function? | ❌ No | ✅ Yes |
Example | sumArray([]int) |
operate(4, 2, func(x, y int) int { return x * y }) |
When to Use Higher-Order Functions?
✅ When writing generic, reusable functions
✅ When using callbacks or function composition
✅ When implementing custom sorting, filtering, or mapping operations
✅ When applying functional programming patterns