Understanding DOM Element Selection Methods in JavaScript: A Deep Dive
When working with the Document Object Model (DOM), selecting elements is a fundamental operation. This article explores three common methods: getElementById, getElementsByClassName, and querySelector. While they might seem similar at first glance, each has unique characteristics and use cases that are crucial to understand. The Test HTML Let's examine these methods using a simple HTML structure: A Study in Scarlet The Valley of Fear 1. getElementById: The Specific Selector const title = document.getElementById('title'); console.log(title); // Output: // A Study in Scarlet getElementById is the most straightforward and fastest of the three methods. Here's why: Returns a single Element object (or null if not found) Performs a direct lookup in the document's ID map Has the best performance of all selector methods Is not live-updating (returns a static reference)
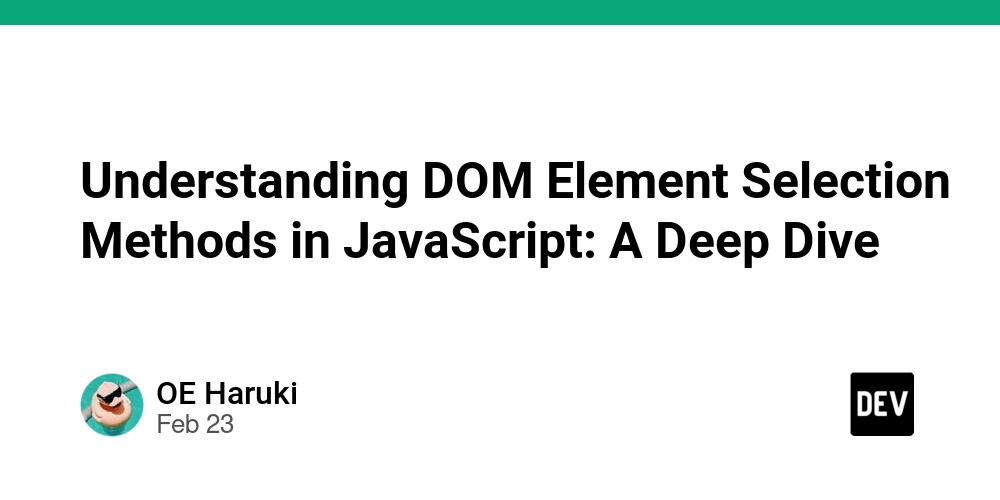
When working with the Document Object Model (DOM), selecting elements is a fundamental operation. This article explores three common methods: getElementById
, getElementsByClassName
, and querySelector
. While they might seem similar at first glance, each has unique characteristics and use cases that are crucial to understand.
The Test HTML
Let's examine these methods using a simple HTML structure:
id="title" class="title">A Study in Scarlet
class="title">The Valley of Fear
1. getElementById: The Specific Selector
const title = document.getElementById('title');
console.log(title);
// Output:
// A Study in Scarlet
getElementById
is the most straightforward and fastest of the three methods. Here's why:
- Returns a single Element object (or null if not found)
- Performs a direct lookup in the document's ID map
- Has the best performance of all selector methods
- Is not live-updating (returns a static reference)