Ultimate Interview Prep Guide: React, Git, Behavioral, and DSA
Preparing for a tech interview can be overwhelming, especially when it covers various domains like React, JavaScript, Git, behavioral questions, and data structures. This guide brings together the most frequently asked and impactful questions to help you ace your next interview. ⚛️ React & JavaScript – Core Concepts 1. JSX Syntax JSX lets you write HTML-like syntax in JavaScript. Expressions are wrapped in curly braces: const greeting = Hello, {userName}!; 2. JSX in the Browser Browsers don't understand JSX directly. Tools like Babel transpile JSX to vanilla JavaScript. 3. Key Prop for Lists React uses the key prop to efficiently update and re-render list items: {items.map(item => {item.name})} 4. State in React The state holds dynamic data. For class components, update it with: this.setState({ count: 5 }); 5. Refs in React Refs give access to DOM nodes or React elements. Updating refs does not trigger a re-render. 6. useMemo for Optimization useMemo memoizes values to prevent expensive recalculations: const memoizedValue = useMemo(() => computeExpensiveValue(a, b), [a, b]); 7. useEffect Hook useEffect acts as a combination of componentDidMount, componentDidUpdate, and componentWillUnmount. 8. Portals React Portals allow rendering elements outside the DOM hierarchy of the parent component. 9. Controlled vs. Uncontrolled Components Controlled: State handled by React. Uncontrolled: DOM manages its own state. 10. Virtual DOM React uses a Virtual DOM to compare changes and efficiently update only what's necessary. 11. Higher-Order Components (HOCs) HOCs are functions that return a new component with added behavior.
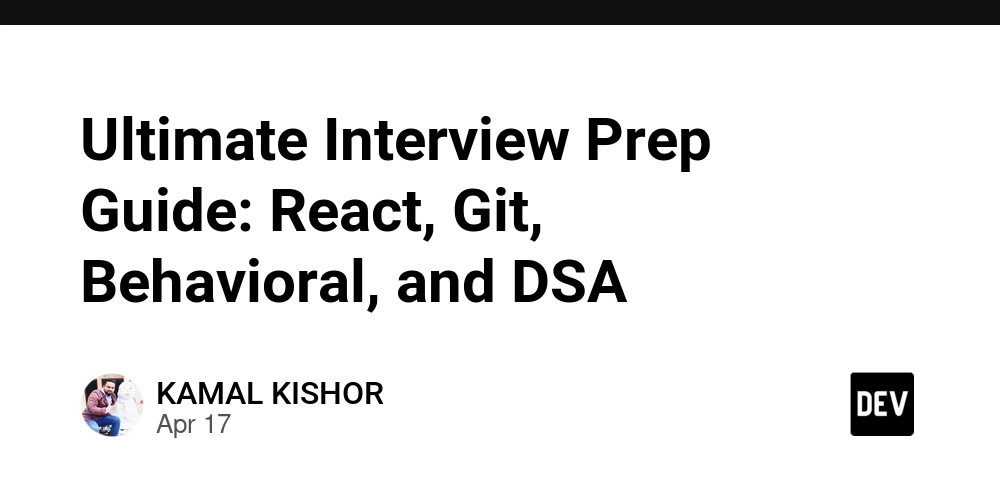
Preparing for a tech interview can be overwhelming, especially when it covers various domains like React, JavaScript, Git, behavioral questions, and data structures. This guide brings together the most frequently asked and impactful questions to help you ace your next interview.
⚛️ React & JavaScript – Core Concepts
1. JSX Syntax
JSX lets you write HTML-like syntax in JavaScript. Expressions are wrapped in curly braces:
const greeting = <h1>Hello, {userName}!h1>;
2. JSX in the Browser
Browsers don't understand JSX directly. Tools like Babel transpile JSX to vanilla JavaScript.
3. Key Prop for Lists
React uses the key
prop to efficiently update and re-render list items:
{items.map(item => <li key={item.id}>{item.name}li>)}
4. State in React
The state
holds dynamic data. For class components, update it with:
this.setState({ count: 5 });
5. Refs in React
Refs give access to DOM nodes or React elements. Updating refs does not trigger a re-render.
6. useMemo for Optimization
useMemo
memoizes values to prevent expensive recalculations:
const memoizedValue = useMemo(() => computeExpensiveValue(a, b), [a, b]);
7. useEffect Hook
useEffect
acts as a combination of componentDidMount
, componentDidUpdate
, and componentWillUnmount
.
8. Portals
React Portals allow rendering elements outside the DOM hierarchy of the parent component.
9. Controlled vs. Uncontrolled Components
- Controlled: State handled by React.
- Uncontrolled: DOM manages its own state.
10. Virtual DOM
React uses a Virtual DOM to compare changes and efficiently update only what's necessary.
11. Higher-Order Components (HOCs)
HOCs are functions that return a new component with added behavior.