Typescript interfaces vs types
In TypeScript there are two ways for defining the shape of an object. Using interfaces: interface Person { name: string; age: number; } Using type alias: type Person = { name: string; age: number; } What are the differences between them? Interfaces can only define the shape of objects, but types can be used to define any type. type MyVar = string | number; // we can't do this with interfaces Interfaces can be reopened interface Person { name: string; } interface Person { age: number; } // Person must have the two properties name and age const john: Person = { name: "John", age: 20 } // This could be very helpful if I'm using an interface from a third-party library, so I can add more properties to the imported interface without creating a new one. Interfaces can be inherited using the extends keyword interface Animal { name: string; } interface Dog extends Animal { age: number; } // Multiple inheritance interface Patient { id: number; } interface Husky extends Dog, Patient { order: number; } Inheritance can also be implemented with type alias using the & intersection operator type Animal = { name: string; }; type Dog = Animal & { breed: string; }; Conclusion: Interfaces are used to define objects' shapes. Interfaces can be reopened. Interfaces can be inherited using the extends keyword. Both interfaces and type aliases can be used to define objects' shapes, but interfaces make more sense as they look more familiar when it comes to inheritance and OOP concepts.
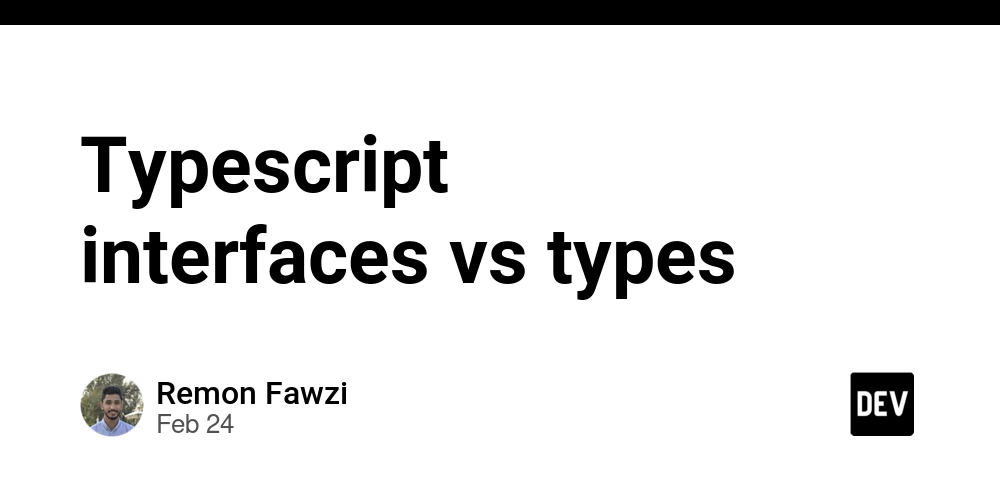
In TypeScript there are two ways for defining the shape of an object.
Using interfaces:
interface Person {
name: string;
age: number;
}
Using type alias:
type Person = {
name: string;
age: number;
}
What are the differences between them?
Interfaces can only define the shape of objects, but types can be used to define any type.
type MyVar = string | number; // we can't do this with interfaces
- Interfaces can be reopened
interface Person {
name: string;
}
interface Person {
age: number;
}
// Person must have the two properties name and age
const john: Person = {
name: "John",
age: 20
}
// This could be very helpful if I'm using an interface from a third-party library, so I can add more properties to the imported interface without creating a new one.
- Interfaces can be inherited using the extends keyword
interface Animal {
name: string;
}
interface Dog extends Animal {
age: number;
}
// Multiple inheritance
interface Patient {
id: number;
}
interface Husky extends Dog, Patient {
order: number;
}
Inheritance can also be implemented with type alias using the & intersection operator
type Animal = {
name: string;
};
type Dog = Animal & {
breed: string;
};
Conclusion:
- Interfaces are used to define objects' shapes.
- Interfaces can be reopened.
- Interfaces can be inherited using the extends keyword.
- Both interfaces and type aliases can be used to define objects' shapes, but interfaces make more sense as they look more familiar when it comes to inheritance and OOP concepts.