TS1407: Matched by include pattern '{0}' in '{1}'
TS1407: Matched by include pattern '{0}' in '{1}' TypeScript is a superset of JavaScript designed to improve the development experience by adding strong typing and additional language features. It enables developers to catch potential issues during development by leveraging static type checking, a feature not natively available in JavaScript. The "types" in TypeScript refer to specific constraints or shapes you assign to variables, functions, or objects, describing what kind of values they can hold—be it strings, numbers, arrays, or custom object shapes. If you’re new to TypeScript or want to deepen your understanding, it’s beneficial to explore modern tools to enhance your learning. Feel free to subscribe to my blog and try AI tools like GPTeach to understand how to use TypeScript effectively and improve your coding skills. In this article, we’ll focus on TypeScript compilation issues, specifically the error TS1407: Matched by include pattern '{0}' in '{1}'. This error can be confusing, so we'll break down what it means, why it happens, and how to fix it. By the end of this guide, you’ll know how to resolve it and avoid common pitfalls. What Are Types in TypeScript? To better understand the TS1407 error, it’s essential to grasp the concept of types in TypeScript. A type defines the shape or structure of data and ensures a consistent way of handling values throughout your program. Example of basic types: let name: string = "Alice"; let age: number = 30; let isStudent: boolean = false; Types are crucial because they enable TypeScript's type-checking features, which help you write safer and more maintainable code. They prevent you from assigning the wrong data type, which could lead to runtime bugs in JavaScript. Understanding TS1407: Matched by include pattern '{0}' in '{1}' The TS1407 error typically occurs during the compilation process. It is not a runtime error but a TypeScript compiler issue, often related to the tsconfig.json file—a configuration file that dictates how TypeScript should process your code. Specifically, this error indicates that the TypeScript compiler is including or attempting to include files based on a pattern you've specified (often in the include or files array) but there’s a mismatch or unexpected behavior with those patterns. Let’s break down what this error means step by step. Error Breakdown: {0} and {1}: These placeholders in the error message will be replaced with actual values by the TypeScript compiler: {0}: The include pattern you've specified in your tsconfig.json. {1}: The location or path where this pattern matched files. Example error message: TS1407: Matched by include pattern 'src/**/*.ts' in 'src/app'. Implication: The error hints that the TypeScript compiler matched certain files based on the glob (glob is a widely-used file-matching pattern) or path in your include pattern, but the files might not conform to expected TypeScript type definitions or could cause issues with your type system. Why It Happens: Your tsconfig.json file's include patterns may be too broad, causing files with errors or incorrect types to be included. Errors may arise if incompatible JavaScript files are getting compiled as TypeScript (e.g., files with missing type annotations). The files being matched may not be intended for TypeScript compilation (e.g., libraries, test files, or plain JavaScript files). Example of TS1407: Matched by include pattern '{0}' in '{1}' Let’s consider the following code structure: File Structure: project/ tsconfig.json src/ main.ts utils.js helper/ index.ts helper-spec.js tsconfig.json Example: { "compilerOptions": { "target": "ESNext", "module": "commonjs" }, "include": [ "src/**/*" ] } This configuration tells the TypeScript compiler to match all files in src/ and its subdirectories. Since the pattern is broad, the compiler includes files like utils.js and helper-spec.js, which may not have valid TypeScript definitions or type annotations. This can trigger the TS1407: Matched by include pattern '{0}' in '{1}' error if those files contain issues or conflicts. How to Fix TS1407: Matched by include pattern '{0}' in '{1}' To fix the error, take the following steps: 1. Narrow Down Include Patterns Revise the include patterns (glob expressions) in your tsconfig.json to include only the necessary files for TypeScript compilation. For example: { "include": [ "src/**/*.ts" // Only include .ts files in src/ subdirectories ] } 2. Exclude Problematic Files Use the exclude field to explicitly exclude files that shouldn’t be compiled: { "exclude": [ "src/**/*.js", // Exclude plain JavaScript files "src/helper/*.spec" // Exclude test files ] } 3. Add Type Definitions to Non-Type
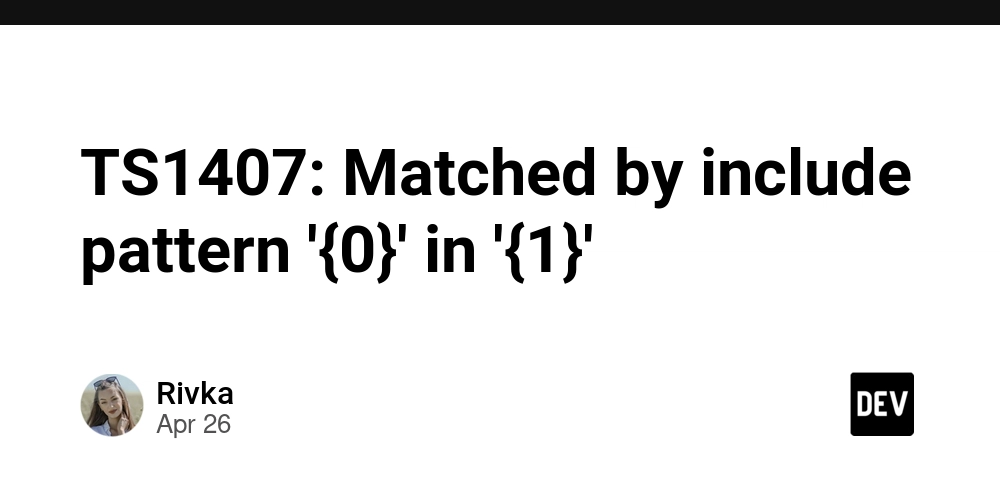
TS1407: Matched by include pattern '{0}' in '{1}'
TypeScript is a superset of JavaScript designed to improve the development experience by adding strong typing and additional language features. It enables developers to catch potential issues during development by leveraging static type checking, a feature not natively available in JavaScript. The "types" in TypeScript refer to specific constraints or shapes you assign to variables, functions, or objects, describing what kind of values they can hold—be it strings, numbers, arrays, or custom object shapes.
If you’re new to TypeScript or want to deepen your understanding, it’s beneficial to explore modern tools to enhance your learning. Feel free to subscribe to my blog and try AI tools like GPTeach to understand how to use TypeScript effectively and improve your coding skills.
In this article, we’ll focus on TypeScript compilation issues, specifically the error TS1407: Matched by include pattern '{0}' in '{1}'. This error can be confusing, so we'll break down what it means, why it happens, and how to fix it. By the end of this guide, you’ll know how to resolve it and avoid common pitfalls.
What Are Types in TypeScript?
To better understand the TS1407 error, it’s essential to grasp the concept of types in TypeScript. A type defines the shape or structure of data and ensures a consistent way of handling values throughout your program.
Example of basic types:
let name: string = "Alice";
let age: number = 30;
let isStudent: boolean = false;
Types are crucial because they enable TypeScript's type-checking features, which help you write safer and more maintainable code. They prevent you from assigning the wrong data type, which could lead to runtime bugs in JavaScript.
Understanding TS1407: Matched by include pattern '{0}' in '{1}'
The TS1407 error typically occurs during the compilation process. It is not a runtime error but a TypeScript compiler issue, often related to the tsconfig.json
file—a configuration file that dictates how TypeScript should process your code. Specifically, this error indicates that the TypeScript compiler is including or attempting to include files based on a pattern you've specified (often in the include
or files
array) but there’s a mismatch or unexpected behavior with those patterns.
Let’s break down what this error means step by step.
Error Breakdown:
-
{0}
and{1}
: These placeholders in the error message will be replaced with actual values by the TypeScript compiler:-
{0}
: The include pattern you've specified in yourtsconfig.json
. -
{1}
: The location or path where this pattern matched files.
-
Example error message:
TS1407: Matched by include pattern 'src/**/*.ts' in 'src/app'.
Implication: The error hints that the TypeScript compiler matched certain files based on the glob (
glob
is a widely-used file-matching pattern) or path in your include pattern, but the files might not conform to expected TypeScript type definitions or could cause issues with your type system.-
Why It Happens:
- Your
tsconfig.json
file'sinclude
patterns may be too broad, causing files with errors or incorrect types to be included. - Errors may arise if incompatible JavaScript files are getting compiled as TypeScript (e.g., files with missing type annotations).
- The files being matched may not be intended for TypeScript compilation (e.g., libraries, test files, or plain JavaScript files).
- Your
Example of TS1407: Matched by include pattern '{0}' in '{1}'
Let’s consider the following code structure:
File Structure:
project/
tsconfig.json
src/
main.ts
utils.js
helper/
index.ts
helper-spec.js
tsconfig.json Example:
{
"compilerOptions": {
"target": "ESNext",
"module": "commonjs"
},
"include": [
"src/**/*"
]
}
This configuration tells the TypeScript compiler to match all files in src/
and its subdirectories. Since the pattern is broad, the compiler includes files like utils.js
and helper-spec.js
, which may not have valid TypeScript definitions or type annotations. This can trigger the TS1407: Matched by include pattern '{0}' in '{1}' error if those files contain issues or conflicts.
How to Fix TS1407: Matched by include pattern '{0}' in '{1}'
To fix the error, take the following steps:
1. Narrow Down Include Patterns
Revise the include
patterns (glob expressions) in your tsconfig.json
to include only the necessary files for TypeScript compilation. For example:
{
"include": [
"src/**/*.ts" // Only include .ts files in src/ subdirectories
]
}
2. Exclude Problematic Files
Use the exclude
field to explicitly exclude files that shouldn’t be compiled:
{
"exclude": [
"src/**/*.js", // Exclude plain JavaScript files
"src/helper/*.spec" // Exclude test files
]
}
3. Add Type Definitions to Non-TypeScript Files
If you want certain JavaScript files to be included, add type definitions using .d.ts
files. For example, if utils.js
has a function:
// utils.js
export function greet(name) {
return `Hello, ${name}`;
}
You can create a utils.d.ts
file with the following:
declare module "./utils" {
export function greet(name: string): string;
}
Important to Know!
-
Glob Patterns Matter: Broad patterns like
src/**/*
can lead to unexpected matches. Be specific with your patterns to avoid including irrelevant files. -
Strict Compiler Options Prevent Errors: Enabling strictness options, such as
strict: true
, can help catch type errors early.
FAQ: TS1407: Matched by include pattern '{0}' in '{1}'
Q1: How do I know which files are causing the issue?
You can enable verbose logging in TypeScript by running the compiler with the --traceResolution
flag:
tsc --traceResolution
This will show which files were included.
Q2: Can I ignore certain files without removing them?
Yes, use the exclude
field in tsconfig.json
. It prevents files from being processed while keeping them in your project.
Important to Know!
Correct Module Imports Are Essential: When working with JavaScript files in a TypeScript project, ensure they're imported correctly, and type definitions exist if needed.
Use
noEmit
to Troubleshoot: Pass the--noEmit
option to TypeScript when debugging include issues. This will halt file outputs, letting you focus exclusively on troubleshooting.
Understanding and fixing compilation errors like TS1407: Matched by include pattern '{0}' in '{1}' requires careful configuration of TypeScript projects. By narrowing down patterns, adding type definitions, and using proper exclusions, you can eliminate any unnecessary type errors and improve project structure.