TS1410: File is matched by 'files' list specified here
TS1410: File is matched by 'files' list specified here TypeScript is a strongly-typed programming language that builds on JavaScript, adding optional static types and other powerful features. In simpler terms, TypeScript is a "superset" of JavaScript, meaning it extends the language by introducing new tools that make it easier to catch errors during development. One of TypeScript's key features is its type system, including support for types (a way to define the kinds of data that variables, functions, or objects can hold). The compiler ensures that all types are being used correctly before the code runs, helping developers avoid bugs. If you enjoy learning about TypeScript, best practices, or tools to aid your coding journey, be sure to subscribe to our blog or use AI tools like gpteach.us to level up your skills! Now, let’s dive into today’s topic. What is a Superset Language? A "superset" language means that TypeScript contains everything in JavaScript, along with additional features and functionalities. Since TypeScript directly extends JavaScript, any valid JavaScript code is also valid TypeScript code. Developers can write traditional JavaScript logic side-by-side with type information. This makes adopting TypeScript straightforward for teams already using JavaScript. For instance, in JavaScript, you might write: function add(a, b) { return a + b; } In TypeScript, the same function can be typed for additional safety: function add(a: number, b: number): number { return a + b; } Notice the use of : number? It tells TypeScript that both a and b are numbers, and the function itself will also return a number. This type information allows the compiler to catch mistakes early, like passing a string to the function instead of a number. Explaining TS1410: File is matched by 'files' list specified here. When working with TypeScript, especially in larger projects, you may encounter the error: error TS1410: File is matched by 'files' list specified here. This error originates from the tsconfig.json file, a special configuration file TypeScript uses to understand how to compile your code. The issue arises when a file in your project is explicitly included or referenced incorrectly in the files list of the tsconfig.json configuration. Breaking Down the Error Here’s a simplified explanation of why this happens: The tsconfig.json file allows you to specify which files (or directories) are part of your project. The files property can be used to include specific files rather than relying on automatic inclusion. If a file is included in the files list but TypeScript encounters a conflict, redundancy, or mismatch during compilation, you get this error: "TS1410: File is matched by 'files' list specified here." Example: When Does the Error Occur? Example tsconfig.json File { "compilerOptions": { "strict": true, "target": "ES2020", }, "files": [ "src/main.ts", "src/utils/helper.ts" ] } In this example, the files array explicitly includes the files main.ts and helper.ts. If you mistakenly include src/utils/helper.ts twice, either directly or through additional configuration such as include, you might encounter the TS1410 error. Problematic Case Another clear example is when there’s overlap between the files setting and wildcard or directory-based include/exclude settings in the same configuration file. For example: { "include": ["src/**/*.ts"], "files": ["src/utils/helper.ts"] } Here, the file src/utils/helper.ts would already be included by the include wildcard (src/**/*.ts matches all .ts files in the src directory). Specifying it in both include and files causes redundancy, which leads to the error. How to Fix TS1410: File is matched by 'files' list specified here. Here’s how you can fix the error step-by-step: Avoid Mixing files and include: If you’re using include or exclude patterns in your tsconfig.json, avoid also using the files property unless absolutely necessary. Choose one approach for clarity and simplicity. For example: { "include": ["src/**/*.ts"], "exclude": ["node_modules"] } Instead of explicitly listing files like this: { "include": ["src/**/*.ts"], "files": ["src/utils/helper.ts"] } Remove Redundant Entries: Double-check the files array to remove any duplicates. { "files": [ "src/utils/helper.ts" ] } In most cases, the include directive can be sufficient without needing to manually specify specific files in the files array. Verify Paths: Ensure that the file paths specified in files are correct and do not overlap with include patterns. Use extends to Inherit Configurations: For complex projects, consider breaking configuration files into modular pieces using the extends property to avoid conflicts: Base configuration (base-tsco
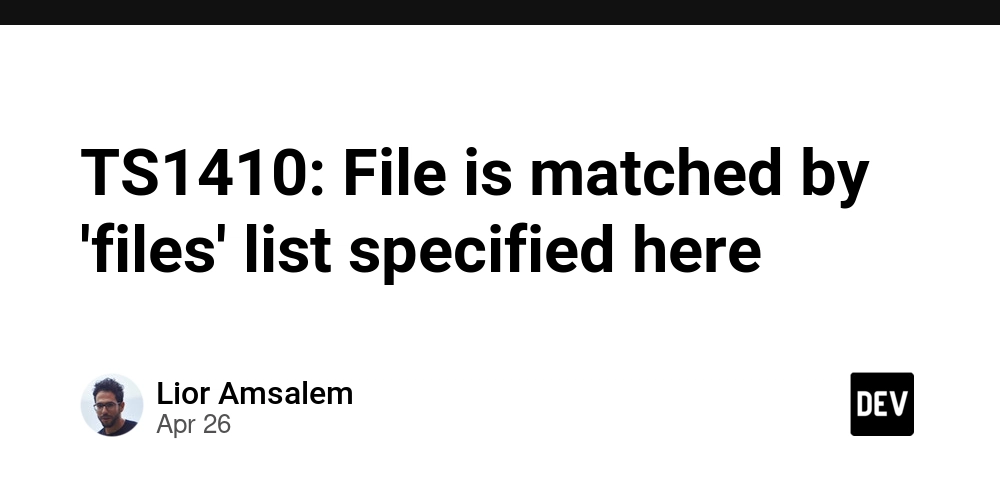
TS1410: File is matched by 'files' list specified here
TypeScript is a strongly-typed programming language that builds on JavaScript, adding optional static types and other powerful features. In simpler terms, TypeScript is a "superset" of JavaScript, meaning it extends the language by introducing new tools that make it easier to catch errors during development. One of TypeScript's key features is its type system, including support for types (a way to define the kinds of data that variables, functions, or objects can hold). The compiler ensures that all types are being used correctly before the code runs, helping developers avoid bugs.
If you enjoy learning about TypeScript, best practices, or tools to aid your coding journey, be sure to subscribe to our blog or use AI tools like gpteach.us to level up your skills! Now, let’s dive into today’s topic.
What is a Superset Language?
A "superset" language means that TypeScript contains everything in JavaScript, along with additional features and functionalities. Since TypeScript directly extends JavaScript, any valid JavaScript code is also valid TypeScript code. Developers can write traditional JavaScript logic side-by-side with type information. This makes adopting TypeScript straightforward for teams already using JavaScript.
For instance, in JavaScript, you might write:
function add(a, b) {
return a + b;
}
In TypeScript, the same function can be typed for additional safety:
function add(a: number, b: number): number {
return a + b;
}
Notice the use of : number
? It tells TypeScript that both a
and b
are numbers, and the function itself will also return a number. This type information allows the compiler to catch mistakes early, like passing a string to the function instead of a number.
Explaining TS1410: File is matched by 'files' list specified here.
When working with TypeScript, especially in larger projects, you may encounter the error:
error TS1410: File is matched by 'files' list specified here.
This error originates from the tsconfig.json
file, a special configuration file TypeScript uses to understand how to compile your code. The issue arises when a file in your project is explicitly included or referenced incorrectly in the files
list of the tsconfig.json
configuration.
Breaking Down the Error
Here’s a simplified explanation of why this happens:
- The
tsconfig.json
file allows you to specify which files (or directories) are part of your project. Thefiles
property can be used to include specific files rather than relying on automatic inclusion. - If a file is included in the
files
list but TypeScript encounters a conflict, redundancy, or mismatch during compilation, you get this error: "TS1410: File is matched by 'files' list specified here."
Example: When Does the Error Occur?
Example tsconfig.json File
{
"compilerOptions": {
"strict": true,
"target": "ES2020",
},
"files": [
"src/main.ts",
"src/utils/helper.ts"
]
}
In this example, the files
array explicitly includes the files main.ts
and helper.ts
. If you mistakenly include src/utils/helper.ts
twice, either directly or through additional configuration such as include
, you might encounter the TS1410 error.
Problematic Case
Another clear example is when there’s overlap between the files
setting and wildcard or directory-based include
/exclude
settings in the same configuration file. For example:
{
"include": ["src/**/*.ts"],
"files": ["src/utils/helper.ts"]
}
Here, the file src/utils/helper.ts
would already be included by the include
wildcard (src/**/*.ts
matches all .ts
files in the src
directory). Specifying it in both include
and files
causes redundancy, which leads to the error.
How to Fix TS1410: File is matched by 'files' list specified here.
Here’s how you can fix the error step-by-step:
-
Avoid Mixing
files
andinclude
: If you’re usinginclude
orexclude
patterns in yourtsconfig.json
, avoid also using thefiles
property unless absolutely necessary. Choose one approach for clarity and simplicity. For example:
{
"include": ["src/**/*.ts"],
"exclude": ["node_modules"]
}
Instead of explicitly listing files like this:
{
"include": ["src/**/*.ts"],
"files": ["src/utils/helper.ts"]
}
-
Remove Redundant Entries:
Double-check the
files
array to remove any duplicates.
{
"files": [
"src/utils/helper.ts"
]
}
In most cases, the include
directive can be sufficient without needing to manually specify specific files in the files
array.
Verify Paths:
Ensure that the file paths specified infiles
are correct and do not overlap withinclude
patterns.Use
extends
to Inherit Configurations:
For complex projects, consider breaking configuration files into modular pieces using theextends
property to avoid conflicts:
Base configuration (base-tsconfig.json):
{
"compilerOptions": {
"strict": true,
"target": "ES2020",
},
"include": ["src/**/*.ts"]
}
Then, extend it:
{
"extends": "./base-tsconfig.json",
"files": ["src/utils/another-helper.ts"]
}
Important to Know!
-
Understand
tsconfig.json
: Thetsconfig.json
file governs how TypeScript compiles your project. It is essential to understand properties likeinclude
,exclude
, andfiles
to manage your project configurations effectively. -
Avoid Overlapping Configuration: Be careful not to mix explicit file references (
files
) with generic patterns (include
). Doing so can cause redundancy and confusion. -
Validate Paths: Ensure the paths in
files
orinclude
settings are accurate, including case sensitivity on case-sensitive filesystems (e.g., macOS or Linux).
FAQ: Common Questions About TS1410
Q1: Can I just remove the files
property altogether?
Yes, you can remove the files
property if your project is meant to include all .ts
files in a directory using the include
setting. Use files
only if you need to specify individual files explicitly.
Q2: Why doesn’t TypeScript ignore duplicates automatically?
While this might seem like a feature to have, TypeScript enforces strict rules to ensure clarity in the compilation process. Conflicts caused by redundancy may indicate potential problems in project setup.
Q3: How do I debug this error in large projects?
Start by commenting out the files
or include
properties section by section in your tsconfig.json
. Rebuild your project after each change to identify the exact source of duplication or mismatch.
By understanding and addressing the underlying cause of TS1410: File is matched by 'files' list specified here., you can resolve this error and manage your TypeScript configurations with confidence. Proper configuration is crucial for ensuring your TypeScript project compiles efficiently and accurately. Keep exploring TypeScript to unlock its full potential!