TS2333: 'this' cannot be referenced in constructor arguments
TS2333: 'this' cannot be referenced in constructor arguments TypeScript is a statically-typed superset of JavaScript designed to add type safety and developer-friendly features to JavaScript code. A "superset" means that TypeScript builds on JavaScript by adding new functionalities, while still allowing you to write plain JavaScript. One of its most powerful features is the introduction of types (a system to define constraints on the structure of your data). This is immensely beneficial when working in large projects to detect errors at compile time, ensuring your codebase is reliable and maintainable. Types in TypeScript help us describe the shape of an object, function, or data. For instance, when you define a function or an object, you can explicitly specify what inputs it expects and what outputs it will produce. This reduces ambiguity and promotes clean coding practices. If you’re looking to master TypeScript concepts or even use AI tools to boost your coding journey, consider subscribing to our blog or using GPTeach to explore how AI can assist you in becoming an expert developer. Now, let’s jump into one of the common errors in TypeScript: TS2333: 'this' cannot be referenced in constructor arguments. What are Types in TypeScript? Before diving into TS2333: 'this' cannot be referenced in constructor arguments, let’s define what types are. Put simply, types in TypeScript are a mechanism to define how variables, functions, and objects behave. For example: // Example of type annotations let username: string = 'John'; // 'username' must always be a string let age: number = 25; // 'age' must always be a number // Example of a function type function add(a: number, b: number): number { return a + b; // Both "a" and "b" must be numbers, and the result is also a number } Types act like a safety net for your applications, preventing runtime errors by ensuring everything functions as expected during compile time. Understanding the Error: TS2333: 'this' cannot be referenced in constructor arguments TypeScript error TS2333: 'this' cannot be referenced in constructor arguments occurs when you accidentally try to reference the current instance (this) during the parameter initialization in the constructor of a class. This is problematic because the this keyword refers to the current instance of the class, but during the parameter initialization phase of a constructor, the current instance is not yet fully created. Therefore, TypeScript disallows this usage to prevent inconsistent or improper behavior. Here's an example of bad code that causes this error: class Person { name: string; constructor(public id: number, name = this.name) { // ERROR: TS2333: 'this' cannot be referenced in constructor arguments this.name = name; } } When compiling this code, TypeScript throws the error TS2333. The issue arises because you're trying to use this.name when this hasn’t been fully initialized yet. TypeScript explicitly prohibits such references to ensure the safety of the code. Why Does This Restriction Exist? When a class is being instantiated (created), the constructor function runs to initialize the object. However, the object itself does not exist before the constructor finishes executing. External references to this during the parameter definition stage might lead to undefined or incorrect object states. This restriction helps protect against subtle bugs where the object instance is partially defined during its initialization. Fixing TS2333: 'this' cannot be referenced in constructor arguments The solution is to avoid referencing this directly in constructor arguments. Instead, rely on initializing these properties explicitly within the body of the constructor, after the instance is fully usable. Here’s a corrected version of the above code: class Person { name: string; constructor(public id: number, name?: string) { this.name = name || "Default Name"; } } In this fixed example: name is passed as an optional parameter (name?). We check if name is provided. If not, a default value is assigned. The this.name property is set explicitly inside the constructor body (not in the argument list), ensuring no reference to this occurs before the object is fully created. Important to Know! When encountering TS2333: 'this' cannot be referenced in constructor arguments, never use this in the parameter section. Always perform any initialization within the constructor's body. TypeScript protects your code by flagging these situations, as improperly using this can lead to undefined behavior in critical portions of your application. FAQ About TS2333: 'this' cannot be referenced in constructor arguments Q: Can I use this in other parts of the constructor? Yes, you can safely use this anywhere inside the constructor after the object has been initialized.
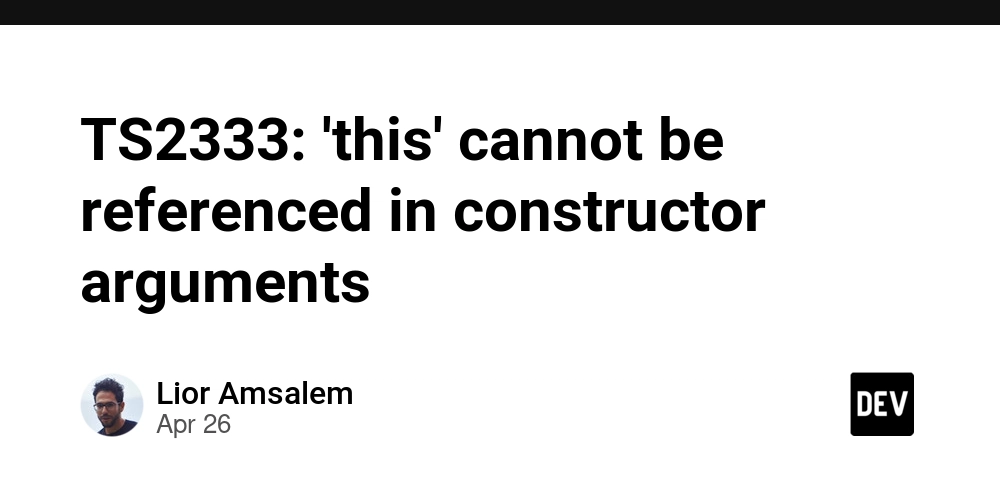
TS2333: 'this' cannot be referenced in constructor arguments
TypeScript is a statically-typed superset of JavaScript designed to add type safety and developer-friendly features to JavaScript code. A "superset" means that TypeScript builds on JavaScript by adding new functionalities, while still allowing you to write plain JavaScript. One of its most powerful features is the introduction of types (a system to define constraints on the structure of your data). This is immensely beneficial when working in large projects to detect errors at compile time, ensuring your codebase is reliable and maintainable.
Types in TypeScript help us describe the shape of an object, function, or data. For instance, when you define a function or an object, you can explicitly specify what inputs it expects and what outputs it will produce. This reduces ambiguity and promotes clean coding practices.
If you’re looking to master TypeScript concepts or even use AI tools to boost your coding journey, consider subscribing to our blog or using GPTeach to explore how AI can assist you in becoming an expert developer.
Now, let’s jump into one of the common errors in TypeScript: TS2333: 'this' cannot be referenced in constructor arguments.
What are Types in TypeScript?
Before diving into TS2333: 'this' cannot be referenced in constructor arguments, let’s define what types are. Put simply, types in TypeScript are a mechanism to define how variables, functions, and objects behave.
For example:
// Example of type annotations
let username: string = 'John'; // 'username' must always be a string
let age: number = 25; // 'age' must always be a number
// Example of a function type
function add(a: number, b: number): number {
return a + b; // Both "a" and "b" must be numbers, and the result is also a number
}
Types act like a safety net for your applications, preventing runtime errors by ensuring everything functions as expected during compile time.
Understanding the Error: TS2333: 'this' cannot be referenced in constructor arguments
TypeScript error TS2333: 'this' cannot be referenced in constructor arguments occurs when you accidentally try to reference the current instance (this
) during the parameter initialization in the constructor of a class. This is problematic because the this
keyword refers to the current instance of the class, but during the parameter initialization phase of a constructor, the current instance is not yet fully created. Therefore, TypeScript disallows this usage to prevent inconsistent or improper behavior.
Here's an example of bad code that causes this error:
class Person {
name: string;
constructor(public id: number, name = this.name) {
// ERROR: TS2333: 'this' cannot be referenced in constructor arguments
this.name = name;
}
}
When compiling this code, TypeScript throws the error TS2333. The issue arises because you're trying to use this.name
when this
hasn’t been fully initialized yet. TypeScript explicitly prohibits such references to ensure the safety of the code.
Why Does This Restriction Exist?
When a class is being instantiated (created), the constructor
function runs to initialize the object. However, the object itself does not exist before the constructor finishes executing. External references to this
during the parameter definition stage might lead to undefined or incorrect object states.
This restriction helps protect against subtle bugs where the object instance is partially defined during its initialization.
Fixing TS2333: 'this' cannot be referenced in constructor arguments
The solution is to avoid referencing this
directly in constructor arguments. Instead, rely on initializing these properties explicitly within the body of the constructor, after the instance is fully usable. Here’s a corrected version of the above code:
class Person {
name: string;
constructor(public id: number, name?: string) {
this.name = name || "Default Name";
}
}
In this fixed example:
-
name
is passed as an optional parameter (name?
). - We check if
name
is provided. If not, a default value is assigned. - The
this.name
property is set explicitly inside the constructor body (not in the argument list), ensuring no reference tothis
occurs before the object is fully created.
Important to Know!
- When encountering TS2333: 'this' cannot be referenced in constructor arguments, never use
this
in the parameter section. Always perform any initialization within the constructor's body. - TypeScript protects your code by flagging these situations, as improperly using
this
can lead to undefined behavior in critical portions of your application.
FAQ About TS2333: 'this' cannot be referenced in constructor arguments
Q: Can I use this
in other parts of the constructor?
Yes, you can safely use this
anywhere inside the constructor after the object has been initialized. For example:
class Example {
value: string;
constructor() {
this.value = "Hello, World!";
}
}
Q: What other scenarios can cause TS2333?
TS2333 will occur in any situation where you directly use this
as part of a constructor parameter, as shown:
class ErrorExample {
property = this.method(); // Error: Cannot reference 'this' before super()
constructor() {
// Fix: Move initialization to constructor
this.property = this.method();
}
method() {
return "value";
}
}
Move all this
-dependent calls inside the constructor body or after super()
if you’re working with inheritance.
Important to Know!
- If you are extending a base class, the
super()
method must be called before usingthis
in any derived class. Otherwise, TypeScript will throw a similar error.
class Base { }
class Derived extends Base {
value: string;
constructor() {
super();
this.value = "Derived instance"; // Safe to use `this` after `super`
}
}
Summary: Key Considerations to Avoid TS2333
To prevent running into TS2333: 'this' cannot be referenced in constructor arguments:
- Avoid referencing
this
in constructor arguments. - Use optional parameters or default values in the constructor definition.
- Perform all
this
-dependent logic inside the constructor body. - When working with inheritance, always call
super()
before usingthis
.
By following these steps, you’ll avoid errors like TS2333 and produce more reliable, maintainable code in TypeScript. Keep practicing your TypeScript knowledge, learning about advanced topics like types, interfaces, and enums as you go. And don’t forget to check out tools like GPTeach to help you on your coding journey.