TS1371: This import is never used as a value and must use 'import type' because 'importsNotUsedAsValues' is set to 'error'
TS1371: This import is never used as a value and must use 'import type' because 'importsNotUsedAsValues' is set to 'error' TypeScript is a powerful programming language that builds on JavaScript by adding static types. In TypeScript, types are used to define the shape of data, ensuring that the code behaves as expected throughout development. With features like interfaces, enums, and type definitions, TypeScript enhances the experience of writing JavaScript by catching errors at compile time rather than runtime. If you want to dive deeper into learning TypeScript or wish to explore AI tools to help you code, I highly recommend you follow my blog or check out gpteach.us for excellent resources. What are Types? Types in TypeScript are a way of saying what kind of data we are working with. A type can be a primitive (like string, number, or boolean), an object, or even a more complex structure. For example, here’s how we can define a few basic types: let userName: string = "Alice"; // string type let age: number = 30; // number type let isActive: boolean = true; // boolean type Now, let’s dive into our main topic: TS1371: This import is never used as a value and must use 'import type' because 'importsNotUsedAsValues' is set to 'error'. TypeScript has a feature that ensures unused imports are not allowed. This is controlled by the importsNotUsedAsValues compiler option. When this option is set to error, TypeScript checks to see if any import statements are not being used as values in the code. If they are, it raises an error — this is where TS1371 comes into play. The Error Explained The error message "TS1371: This import is never used as a value and must use 'import type' because 'importsNotUsedAsValues' is set to 'error'." indicates that we have imported something that is only used for type annotations (like an interface or a type alias), but the import statement is not specified as a type import. Here's an example that causes the TS1371 error: import { User } from './user'; // Suppose User is an interface const user: User = { name: 'Alice', age: 30 }; In the above code, the User type is imported, but because it’s not being used as a value (like a class or a function), it should be imported using the import type syntax. Fixing the Error To fix this TypeScript error, you need to change the import statement to an import type statement. Here’s how you can do that: import type { User } from './user'; // Type importing const user: User = { name: 'Alice', age: 30 }; Now the code is compliant with the TypeScript rules, and the error TS1371: This import is never used as a value and must use 'import type' because 'importsNotUsedAsValues' is set to 'error' is resolved! Important to Know! What are Imports? Imports are used to bring in modules, functions, or types from other files. It's a fundamental part of modular programming that allows code reusability. TypeScript Enforcements: When using TypeScript, if you fail to use import type correctly under this setting, you will run into the TS1371 error, which helps keep the codebase clean and efficient. Frequently Asked Questions (FAQs) Q1: What happens if I ignore the TS1371 error? Ignoring this error will lead to unnecessary clutter in your codebase. It can also cause confusion for others reading the code who might wonder why a type is imported but never used. Q2: Is it always necessary to use 'import type'? It's necessary when you are importing types that are not expected to be values. This distinction keeps TypeScript's type system effective and clean. Q3: Can I disable the importsNotUsedAsValues option? It is possible to disable this option in your tsconfig.json, but it's generally better practice to maintain strict checks for code quality. Important Things to Know Always use import type for types, interfaces, and enums when importsNotUsedAsValues is set to error. Ensure your tsconfig.json reflects the desired level of strictness for your project. Organizing your imports can lead to better code readability and maintainability. Understanding TS1371 is crucial for writing clean TypeScript code. Regularly encountering and addressing such errors will enhance your proficiency and understanding of TypeScript. To stay updated and learn more, don't forget to subscribe or follow my blog, and explore tools like gpteach.us to advance your coding skills!
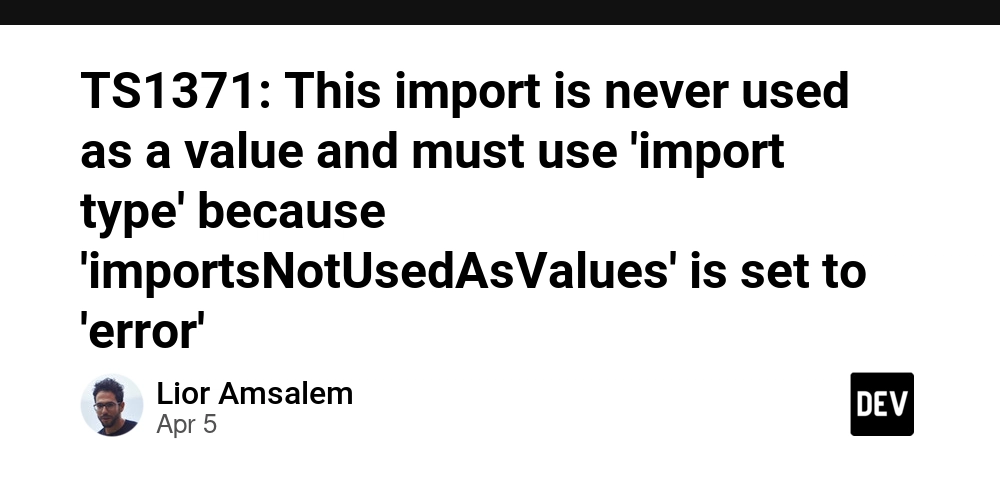
TS1371: This import is never used as a value and must use 'import type' because 'importsNotUsedAsValues' is set to 'error'
TypeScript is a powerful programming language that builds on JavaScript by adding static types. In TypeScript, types are used to define the shape of data, ensuring that the code behaves as expected throughout development. With features like interfaces, enums, and type definitions, TypeScript enhances the experience of writing JavaScript by catching errors at compile time rather than runtime.
If you want to dive deeper into learning TypeScript or wish to explore AI tools to help you code, I highly recommend you follow my blog or check out gpteach.us for excellent resources.
What are Types?
Types in TypeScript are a way of saying what kind of data we are working with. A type can be a primitive (like string
, number
, or boolean
), an object, or even a more complex structure. For example, here’s how we can define a few basic types:
let userName: string = "Alice"; // string type
let age: number = 30; // number type
let isActive: boolean = true; // boolean type
Now, let’s dive into our main topic:
TS1371: This import is never used as a value and must use 'import type' because 'importsNotUsedAsValues' is set to 'error'.
TypeScript has a feature that ensures unused imports are not allowed. This is controlled by the importsNotUsedAsValues
compiler option. When this option is set to error
, TypeScript checks to see if any import statements are not being used as values in the code. If they are, it raises an error — this is where TS1371 comes into play.
The Error Explained
The error message "TS1371: This import is never used as a value and must use 'import type' because 'importsNotUsedAsValues' is set to 'error'." indicates that we have imported something that is only used for type annotations (like an interface or a type alias), but the import statement is not specified as a type import.
Here's an example that causes the TS1371 error:
import { User } from './user'; // Suppose User is an interface
const user: User = { name: 'Alice', age: 30 };
In the above code, the User
type is imported, but because it’s not being used as a value (like a class or a function), it should be imported using the import type
syntax.
Fixing the Error
To fix this TypeScript error, you need to change the import
statement to an import type
statement. Here’s how you can do that:
import type { User } from './user'; // Type importing
const user: User = { name: 'Alice', age: 30 };
Now the code is compliant with the TypeScript rules, and the error TS1371: This import is never used as a value and must use 'import type' because 'importsNotUsedAsValues' is set to 'error' is resolved!
Important to Know!
What are Imports?
Imports are used to bring in modules, functions, or types from other files. It's a fundamental part of modular programming that allows code reusability.TypeScript Enforcements:
When using TypeScript, if you fail to useimport type
correctly under this setting, you will run into the TS1371 error, which helps keep the codebase clean and efficient.
Frequently Asked Questions (FAQs)
Q1: What happens if I ignore the TS1371 error?
Ignoring this error will lead to unnecessary clutter in your codebase. It can also cause confusion for others reading the code who might wonder why a type is imported but never used.
Q2: Is it always necessary to use 'import type'?
It's necessary when you are importing types that are not expected to be values. This distinction keeps TypeScript's type system effective and clean.
Q3: Can I disable the importsNotUsedAsValues
option?
It is possible to disable this option in your tsconfig.json
, but it's generally better practice to maintain strict checks for code quality.
Important Things to Know
- Always use
import type
for types, interfaces, and enums whenimportsNotUsedAsValues
is set toerror
. - Ensure your
tsconfig.json
reflects the desired level of strictness for your project. - Organizing your imports can lead to better code readability and maintainability.
Understanding TS1371 is crucial for writing clean TypeScript code. Regularly encountering and addressing such errors will enhance your proficiency and understanding of TypeScript. To stay updated and learn more, don't forget to subscribe or follow my blog, and explore tools like gpteach.us to advance your coding skills!