TOML Be or Not TOML Be: A Rustacean's Guide to Cargo
Are you ready to embark on your Rust journey with Tom's Obvious, Minimal Language (TOML) by your side? The guide will walk you through using Cargo, Rust's powerful build system and package manager, which uses TOML configuration files that are as straightforward as Tom intended. What is Cargo and Why Should You Care? Cargo is like that friend who always has your back. It handles: Building your code Downloading your dependencies Building those dependencies Making coffee (okay, not really, but almost everything else) While simple Rust programs can survive without Cargo, most Rustaceans swear by it because it makes complex projects manageable and dependency handling a breeze. Let's Get This Show on the Road Step 1: Check If Cargo Is Installed Before we dive in, let's make sure Cargo is actually installed on your system: $ cargo --version You should see something like cargo 1.xx.x. If not, you might need to revisit your Rust installation. Step 2: Creating a New Project with Cargo Let's create your first Cargo project: $ cargo new hello_cargo $ cd hello_cargo The magic command creates a new directory called hello_cargo with everything you need to get started. It's like moving into a fully furnished apartment! Step 3: What Did Cargo Actually Do? Take a peek at what Cargo created: hello_cargo/ ├── Cargo.toml ├── .git/ ├── .gitignore └── src/ └── main.rs The most important bits are: Cargo.toml: Your project's manifest file (in Tom's Obvious, Minimal Language!) src/main.rs: Where your Rust code lives Cargo also initialized a Git repository for version control. How thoughtful! Step 4: Understanding the Mysterious TOML File Open up Cargo.toml and you'll see something like: [package] name = "hello_cargo" version = "0.1.0" edition = "2021" [dependencies] The TOML file is pretty self-explanatory, just as Tom intended: [package] section contains metadata about your project [dependencies] is where you'll list external crates your project needs Step 5: Hello World, Cargo Style Look at src/main.rs and you'll find a familiar friend: fn main() { println!("Hello, world!"); } Cargo has generated a "Hello, world!" program for you. No need to start from scratch! Building and Running with Cargo Now for the fun part—let's see Cargo in action! Building Your Project $ cargo build The command creates an executable in the target/debug directory. Cargo uses this directory because the default build is a debug build (unoptimized but with debugging info). Running Your Project You can run the executable directly: $ ./target/debug/hello_cargo # or .\target\debug\hello_cargo.exe on Windows But that's a mouthful. Cargo offers a shortcut: $ cargo run The command builds (if necessary) and runs your program in one go. It's like a one-stop shop! Checking Your Code Without Building If you just want to make sure your code compiles without producing an executable: $ cargo check This is faster than cargo build and is perfect for when you're in the midst of development and want to check your work frequently. Building for Production When your masterpiece is ready for the world: $ cargo build --release This creates an optimized executable in target/release. It's slower to compile but runs faster—perfect for distributing your program to users. Cargo's Greatest Hits: Command Summary Here's your Cargo command cheat sheet: cargo new project_name - Create a new project cargo build - Build your project (debug mode) cargo run - Build and run your project cargo check - Check if your code compiles cargo build --release - Build with optimizations for release cargo update - Update dependencies cargo doc --open - Build and open documentation Working with Existing Projects Want to contribute to an existing Rust project? It's usually as simple as: $ git clone example.org/someproject $ cd someproject $ cargo build The TOML Philosophy Remember, TOML (Tom's Obvious, Minimal Language) was designed to be easy to read and write, with a clear and obvious structure. When you modify your Cargo.toml file to add dependencies or change project settings, the simplicity of TOML makes the process painless. For example, adding a dependency is as simple as: [dependencies] rand = "0.8.5" No complex syntax or mysterious incantations required! Conclusion: To Cargo or Not To Cargo? That's not even a question. Cargo is an essential tool in the Rust ecosystem. While it might seem like overkill for simple "Hello, world!" programs, its value becomes apparent as your projects grow more complex. With Cargo by your side, you can focus on writing great Rust code while it handles the boring stuff. As Shakespeare might have said if he were a Rust programmer: "TOML be, and
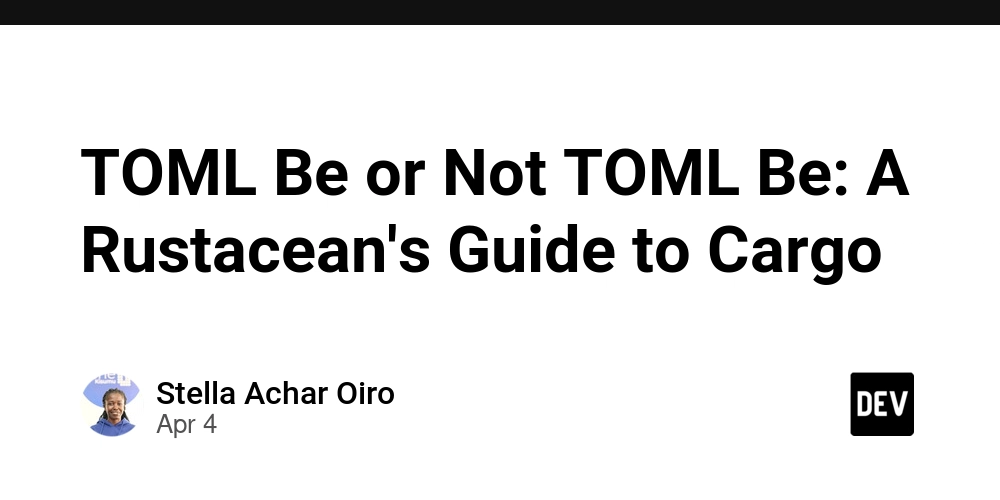
Are you ready to embark on your Rust journey with Tom's Obvious, Minimal Language (TOML) by your side? The guide will walk you through using Cargo, Rust's powerful build system and package manager, which uses TOML configuration files that are as straightforward as Tom intended.
What is Cargo and Why Should You Care?
Cargo is like that friend who always has your back. It handles:
- Building your code
- Downloading your dependencies
- Building those dependencies
- Making coffee (okay, not really, but almost everything else)
While simple Rust programs can survive without Cargo, most Rustaceans swear by it because it makes complex projects manageable and dependency handling a breeze.
Let's Get This Show on the Road
Step 1: Check If Cargo Is Installed
Before we dive in, let's make sure Cargo is actually installed on your system:
$ cargo --version
You should see something like cargo 1.xx.x
. If not, you might need to revisit your Rust installation.
Step 2: Creating a New Project with Cargo
Let's create your first Cargo project:
$ cargo new hello_cargo
$ cd hello_cargo
The magic command creates a new directory called hello_cargo
with everything you need to get started. It's like moving into a fully furnished apartment!
Step 3: What Did Cargo Actually Do?
Take a peek at what Cargo created:
hello_cargo/
├── Cargo.toml
├── .git/
├── .gitignore
└── src/
└── main.rs
The most important bits are:
- Cargo.toml: Your project's manifest file (in Tom's Obvious, Minimal Language!)
- src/main.rs: Where your Rust code lives
Cargo also initialized a Git repository for version control. How thoughtful!
Step 4: Understanding the Mysterious TOML File
Open up Cargo.toml
and you'll see something like:
[package]
name = "hello_cargo"
version = "0.1.0"
edition = "2021"
[dependencies]
The TOML file is pretty self-explanatory, just as Tom intended:
-
[package]
section contains metadata about your project -
[dependencies]
is where you'll list external crates your project needs
Step 5: Hello World, Cargo Style
Look at src/main.rs
and you'll find a familiar friend:
fn main() {
println!("Hello, world!");
}
Cargo has generated a "Hello, world!" program for you. No need to start from scratch!
Building and Running with Cargo
Now for the fun part—let's see Cargo in action!
Building Your Project
$ cargo build
The command creates an executable in the target/debug
directory. Cargo uses this directory because the default build is a debug build (unoptimized but with debugging info).
Running Your Project
You can run the executable directly:
$ ./target/debug/hello_cargo # or .\target\debug\hello_cargo.exe on Windows
But that's a mouthful. Cargo offers a shortcut:
$ cargo run
The command builds (if necessary) and runs your program in one go. It's like a one-stop shop!
Checking Your Code Without Building
If you just want to make sure your code compiles without producing an executable:
$ cargo check
This is faster than cargo build
and is perfect for when you're in the midst of development and want to check your work frequently.
Building for Production
When your masterpiece is ready for the world:
$ cargo build --release
This creates an optimized executable in target/release
. It's slower to compile but runs faster—perfect for distributing your program to users.
Cargo's Greatest Hits: Command Summary
Here's your Cargo command cheat sheet:
-
cargo new project_name
- Create a new project -
cargo build
- Build your project (debug mode) -
cargo run
- Build and run your project -
cargo check
- Check if your code compiles -
cargo build --release
- Build with optimizations for release -
cargo update
- Update dependencies -
cargo doc --open
- Build and open documentation
Working with Existing Projects
Want to contribute to an existing Rust project? It's usually as simple as:
$ git clone example.org/someproject
$ cd someproject
$ cargo build
The TOML Philosophy
Remember, TOML (Tom's Obvious, Minimal Language) was designed to be easy to read and write, with a clear and obvious structure. When you modify your Cargo.toml
file to add dependencies or change project settings, the simplicity of TOML makes the process painless.
For example, adding a dependency is as simple as:
[dependencies]
rand = "0.8.5"
No complex syntax or mysterious incantations required!
Conclusion: To Cargo or Not To Cargo?
That's not even a question. Cargo is an essential tool in the Rust ecosystem. While it might seem like overkill for simple "Hello, world!" programs, its value becomes apparent as your projects grow more complex.
With Cargo by your side, you can focus on writing great Rust code while it handles the boring stuff. As Shakespeare might have said if he were a Rust programmer: "TOML be, and that is the answer!"
Happy Rusting!