The Hidden Risks of Coding with AI Agents (and How to Avoid Them)
AI can speed up your workflow. But hidden beneath that convenience are risks you can’t afford to ignore. In the rush to adopt AI-powered coding agents like ChatGPT, Claude, Cursor, and GitHub Copilot, many developers forget a basic truth: code is not just about what works—it’s about what’s safe, secure, and maintainable. This article explores the hidden risks of AI-assisted development, based on real-world experience, and offers clear, actionable strategies to avoid those pitfalls. Risk #1: Code That Looks Right But Fails Silently AI often generates syntactically correct code that compiles and runs—but behaves incorrectly under specific conditions. Real Case: Silent Data Corruption in an AI-Suggested Function // AI-generated function updateInventory(itemId, quantity) { db.items.find({ id: itemId }).quantity += quantity; } Looks fine, right? But it's not updating the database—find() returns a copy, not a reference. This can silently corrupt logic across production. Fix: await db.items.updateOne({ id: itemId }, { $inc: { quantity } }); Avoid it: Always verify the data model and database methods used. AI doesn’t know your schema. Risk #2: Security Loopholes from Missing Context AI often omits critical context like authentication, authorization, and sanitization—especially for dynamic routes or forms. Real Case: No Authorization Check in Admin Route app.get('/admin/export', async (req, res) => { const data = await getSensitiveData(); res.send(data); }); No middleware. No access control. This route might be exposed in production. Fix: app.get('/admin/export', isAuthenticated, isAdmin, async (req, res) => { const data = await getSensitiveData(); res.send(data); }); Avoid it: Add security prompts, then review every route manually for access control. Risk #3: Dependency Injection with Untrusted Inputs AI might unknowingly suggest logic that injects user-controlled variables into unsafe contexts. Real Case: Template Injection via Unescaped Variables res.send(`Hello ${req.query.name}`); If nameis alert(1), you've got XSS. Fix: const escape = require('escape-html'); res.send(`Hello ${escape(req.query.name)}`); Avoid it: Never trust output that uses user input—always escape or sanitize. How to Use AI Without Falling into These Traps Prompt defensively: Include terms like "secure", "safe", "type-checked", and "idiomatic" in your prompt. Audit everything: Assume nothing is production-ready. Review for correctness, safety, and context. Pair with static analysis: Use tools like ESLint, SonarQube, or SAST to catch structural flaws. Write tests (with AI): Ask AI to generate edge case tests, then extend them yourself. Use AI as a second brain—not a second engineer. Final Take AI agents are brilliant at surfacing ideas and saving time. But when it comes to secure, maintainable code—you’re still the expert in the room. Trust your judgment, not the autocomplete. Let’s Talk: What’s the worst AI-generated bug you’ve seen? What’s your system for catching subtle mistakes before they go live?
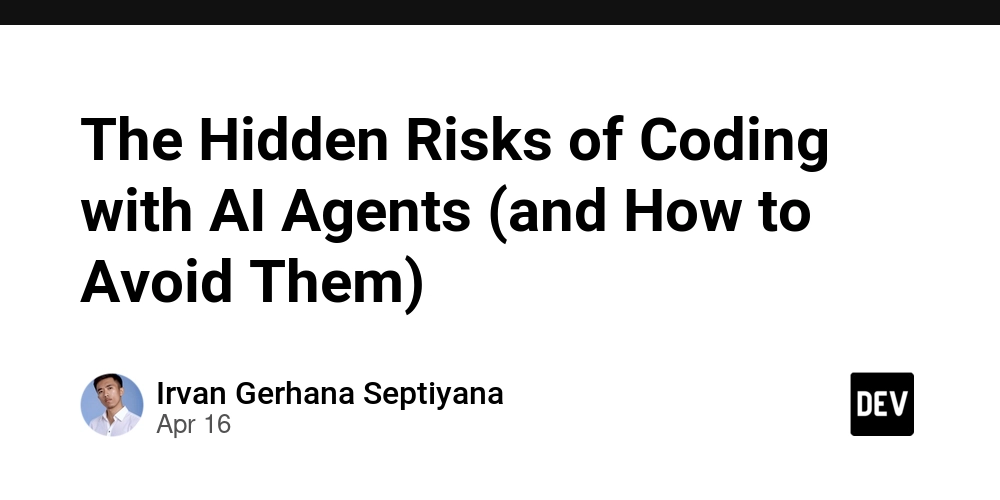
AI can speed up your workflow. But hidden beneath that convenience are risks you can’t afford to ignore.
In the rush to adopt AI-powered coding agents like ChatGPT, Claude, Cursor, and GitHub Copilot, many developers forget a basic truth: code is not just about what works—it’s about what’s safe, secure, and maintainable.
This article explores the hidden risks of AI-assisted development, based on real-world experience, and offers clear, actionable strategies to avoid those pitfalls.
Risk #1: Code That Looks Right But Fails Silently
AI often generates syntactically correct code that compiles and runs—but behaves incorrectly under specific conditions.
Real Case: Silent Data Corruption in an AI-Suggested Function
// AI-generated
function updateInventory(itemId, quantity) {
db.items.find({ id: itemId }).quantity += quantity;
}
Looks fine, right? But it's not updating the database—find() returns a copy, not a reference. This can silently corrupt logic across production.
Fix:
await db.items.updateOne({ id: itemId }, { $inc: { quantity } });
Avoid it: Always verify the data model and database methods used. AI doesn’t know your schema.
Risk #2: Security Loopholes from Missing Context
AI often omits critical context like authentication, authorization, and sanitization—especially for dynamic routes or forms.
Real Case: No Authorization Check in Admin Route
app.get('/admin/export', async (req, res) => {
const data = await getSensitiveData();
res.send(data);
});
No middleware. No access control. This route might be exposed in production.
Fix:
app.get('/admin/export', isAuthenticated, isAdmin, async (req, res) => {
const data = await getSensitiveData();
res.send(data);
});
Avoid it: Add security prompts, then review every route manually for access control.
Risk #3: Dependency Injection with Untrusted Inputs
AI might unknowingly suggest logic that injects user-controlled variables into unsafe contexts.
Real Case: Template Injection via Unescaped Variables
res.send(`Hello ${req.query.name}`);
If name
is , you've got XSS.
Fix:
const escape = require('escape-html');
res.send(`Hello ${escape(req.query.name)}`);
Avoid it: Never trust output that uses user input—always escape or sanitize.
How to Use AI Without Falling into These Traps
- Prompt defensively: Include terms like "secure", "safe", "type-checked", and "idiomatic" in your prompt.
- Audit everything: Assume nothing is production-ready. Review for correctness, safety, and context.
- Pair with static analysis: Use tools like ESLint, SonarQube, or SAST to catch structural flaws.
- Write tests (with AI): Ask AI to generate edge case tests, then extend them yourself.
- Use AI as a second brain—not a second engineer.
Final Take
AI agents are brilliant at surfacing ideas and saving time. But when it comes to secure, maintainable code—you’re still the expert in the room.
Trust your judgment, not the autocomplete.
Let’s Talk: What’s the worst AI-generated bug you’ve seen? What’s your system for catching subtle mistakes before they go live?