The First Step into the World of RxJS: Getting to Know Observables
Learning something new is always a challenge, especially when you're deluged with streams of terms, functions, and concepts that aren't immediately obvious. Let's dive into some simple examples and try to create a mini-plan for mastering this topic. I’m even considering writing a series of articles in this vein, inspired by my experience interacting with newcomers to the field (as long as the topic and presentation spark interest). Why Learn RxJS at All? Reactive programming is becoming an increasingly popular approach in modern application development, especially where asynchronous data is critical—user input, working with WebSockets, event handling, or API requests. RxJS is widely used in frameworks like Angular, where its knowledge is practically essential for working with components, services, and data streams. But even beyond specific framework requirements, RxJS is a powerful tool that simplifies and enhances how we handle asynchronous operations compared to traditional callback or promise approaches. Mastering it will not only give you an edge in managing complex applications but also provide a new perspective on managing data and events. The Problem With Getting Started The problem many beginners face when first encountering the topic of subscriptions lies in the abundance of topics and nuances related to it. Tons of articles, discussions, and documentation on topics like "RxJS mergeMap vs switchMap vs concatMap vs exhaustMap" can cause a headache in just the first fifteen minutes. That’s why my first piece of advice is this: limit the list of topics and tools you’re trying to master to the bare minimum. Decomposition is always important — you need to "eat the elephant piece by piece." Similar to how a chess beginner learns, they first study each piece individually, memorize its capabilities and nuances, and gradually move on to the overarching strategy of the game. So, let’s sweep all the pieces off the board and leave only the pawn. Imagine Yourself as a Magazine Subscriber The core idea of Observables is observing events and responding to them. To clarify, let’s compare Observables with a magazine subscription system: Publisher (Data Source) sends data. The subscriber wants to get updates regularly (data). The subscriber agrees and subscribes. While the subscription is active, updates (data) are received. Key Observations: Subscribed data only starts flowing after the subscription. You can unsubscribe to stop receiving data. Sometimes the publisher stops sending updates entirely. In programming terms: There’s a data source (e.g., an event stream or HTTP response). Subscribers (you) monitor changes. You "subscribe" using subscribe(). While subscribed, you continuously get updates. Diving into Observable Code Basics Traditional Observable Example An Observable is an object that produces data or notifications. The data can be of any type: numbers, strings, arrays, results of HTTP requests. And here’s where the interesting part begins: traditionally, at this point, it’s common to show examples like: import { Observable } from 'rxjs'; const observable = new Observable(subscriber => { subscriber.next(1); subscriber.next(2); subscriber.next(3); subscriber.complete(); }); observable.subscribe({ next: value => console.log(value), complete: () => console.log('Stream completed'), }); // Console output: // 1 // 2 // 3 // Stream completed But in my experience, this approach doesn’t work at all with beginners – too many things happen all at once, which either require lengthy line-by-line explanations or forcing them to “look the other way” and focus only on the “main idea” while ignoring the “other non-essential details.” But we agreed to try and find the smallest possible topic, so I propose starting with the from() operator and building the learning process around it. Why? Simply because it “sweeps away” all the questions related to creating streams, emitting events, completing subscriptions, and so on. Going back to the newspaper example: for now, we just want to figure out how to get our favorite newspaper delivered every day, not how to build a printing press and set up its operations. So, let’s agree that for our basic experiments, we’ll only need two things: the from operator and some collection of objects that we’ll come up with as we go. Where to Run the Code? If you're comfortable setting up a local app for experimenting, do so. Otherwise, consider online tools like PlayCode—quick and easy with RxJS support. Creating a Basic Number Stream Let’s simplify the earlier example using from(): import { from } from 'rxjs'; const obs = from([1, 2, 3]); obs.subscribe(value => console.log(value)); // Console output: // 1 // 2 // 3 That’s the entire basic example, though it’s worth dedicating enough time to it. The printing press analogy isn’t very fitt
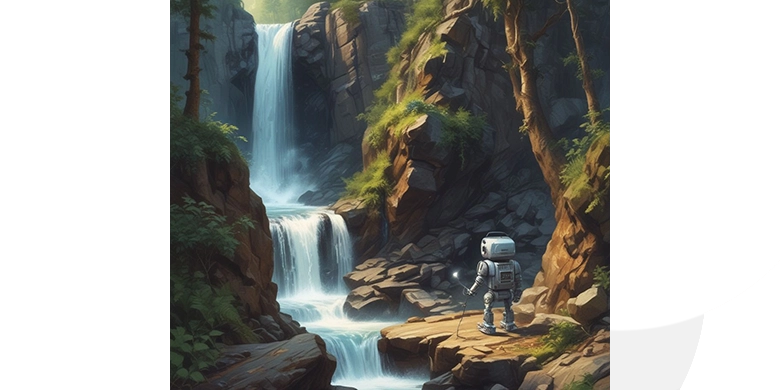
Learning something new is always a challenge, especially when you're deluged with streams of terms, functions, and concepts that aren't immediately obvious. Let's dive into some simple examples and try to create a mini-plan for mastering this topic. I’m even considering writing a series of articles in this vein, inspired by my experience interacting with newcomers to the field (as long as the topic and presentation spark interest).
Why Learn RxJS at All?
Reactive programming is becoming an increasingly popular approach in modern application development, especially where asynchronous data is critical—user input, working with WebSockets, event handling, or API requests. RxJS is widely used in frameworks like Angular, where its knowledge is practically essential for working with components, services, and data streams.
But even beyond specific framework requirements, RxJS is a powerful tool that simplifies and enhances how we handle asynchronous operations compared to traditional callback or promise approaches. Mastering it will not only give you an edge in managing complex applications but also provide a new perspective on managing data and events.
The Problem With Getting Started
The problem many beginners face when first encountering the topic of subscriptions lies in the abundance of topics and nuances related to it. Tons of articles, discussions, and documentation on topics like "RxJS mergeMap vs switchMap vs concatMap vs exhaustMap" can cause a headache in just the first fifteen minutes. That’s why my first piece of advice is this: limit the list of topics and tools you’re trying to master to the bare minimum. Decomposition is always important — you need to "eat the elephant piece by piece." Similar to how a chess beginner learns, they first study each piece individually, memorize its capabilities and nuances, and gradually move on to the overarching strategy of the game. So, let’s sweep all the pieces off the board and leave only the pawn.
Imagine Yourself as a Magazine Subscriber
The core idea of Observables is observing events and responding to them. To clarify, let’s compare Observables with a magazine subscription system:
- Publisher (Data Source) sends data.
- The subscriber wants to get updates regularly (data).
- The subscriber agrees and subscribes.
- While the subscription is active, updates (data) are received.
Key Observations:
- Subscribed data only starts flowing after the subscription.
- You can unsubscribe to stop receiving data.
- Sometimes the publisher stops sending updates entirely.
In programming terms:
- There’s a data source (e.g., an event stream or HTTP response).
- Subscribers (you) monitor changes.
- You "subscribe" using
subscribe()
. - While subscribed, you continuously get updates.
Diving into Observable Code Basics
Traditional Observable Example
An Observable is an object that produces data or notifications. The data can be of any type: numbers, strings, arrays, results of HTTP requests. And here’s where the interesting part begins: traditionally, at this point, it’s common to show examples like:
import { Observable } from 'rxjs';
const observable = new Observable<number>(subscriber => {
subscriber.next(1);
subscriber.next(2);
subscriber.next(3);
subscriber.complete();
});
observable.subscribe({
next: value => console.log(value),
complete: () => console.log('Stream completed'),
});
// Console output:
// 1
// 2
// 3
// Stream completed
But in my experience, this approach doesn’t work at all with beginners – too many things happen all at once, which either require lengthy line-by-line explanations or forcing them to “look the other way” and focus only on the “main idea” while ignoring the “other non-essential details.” But we agreed to try and find the smallest possible topic, so I propose starting with the from()
operator and building the learning process around it. Why? Simply because it “sweeps away” all the questions related to creating streams, emitting events, completing subscriptions, and so on.
Going back to the newspaper example: for now, we just want to figure out how to get our favorite newspaper delivered every day, not how to build a printing press and set up its operations.
So, let’s agree that for our basic experiments, we’ll only need two things: the from
operator and some collection of objects that we’ll come up with as we go.
Where to Run the Code?
- If you're comfortable setting up a local app for experimenting, do so.
- Otherwise, consider online tools like PlayCode—quick and easy with RxJS support.
Creating a Basic Number Stream
Let’s simplify the earlier example using from()
:
import { from } from 'rxjs';
const obs = from([1, 2, 3]);
obs.subscribe(value => console.log(value));
// Console output:
// 1
// 2
// 3
That’s the entire basic example, though it’s worth dedicating enough time to it. The printing press analogy isn’t very fitting here due to the simplicity of what’s happening, so let’s switch to people instead. Sometimes in conversations, we touch on a topic that’s really interesting to the other person, and they start pouring out their knowledge/opinions on the subject (it could be office gossip about colleagues, or it could be the lore of Warhammer – luck plays a role here). That’s exactly how obs
holds its “knowledge” about the numbers 1, 2, 3, so it can dump them on anyone who subscribes. When we subscribe to obs
, we sequentially receive the entire collection of elements. Moreover, if another listener connects to obs
, they will again receive the entire collection from the beginning – just like in real life, you just can’t get this guy to stop talking!
Example (Multiple Subscriptions):
import { from } from 'rxjs';
const obs = from([1, 2, 3]);
obs.subscribe(value => console.log(value)); // First subscriber
obs.subscribe(value => console.log(value)); // Second subscriber
// Console output:
// 1
// 2
// 3
// 1
// 2
// 3
If a second subscriber connects, the data replays from the first item.
What’s Next?
Next, start experimenting with this micro-code, gradually expanding its functionality. Change the data and their types; work, for example, with a collection of strings or objects. Get used to the idea that all these subscriptions are just a versatile tool that works according to a pattern, not some highly complex space shuttle.
Next, it's worth exploring the logic of pipe()
with two operators: map()
and filter()
.
As for pipe()
itself, there’s no need to dwell on it too much—it’s simply a function that allows us to modify the data stream by applying the corresponding operators (we’ll start with the two mentioned).
Operators: filter
and map
filter
– Set Conditions for Data
That guy with the gossip is unlikely to share gossip about you directly with you; he’ll save those stories for another conversation partner. And there you have the idea of filtering: we won’t always work with the full data stream—sometimes, we need to filter out irrelevant data.
import { from, filter } from 'rxjs';
const obs = from([1, 2, 3]).pipe(filter(n => n > 2));
obs.subscribe(value => console.log(value));
// Console output:
// 3
filter
sets a condition for publishing data, and once again, the same suggestion: experiment, change data types and conditions, get fully comfortable with the ideas of filtering so that you approach other operators later with more awareness and understanding.
It's worth noting an important detail here: pipe
can be applied to the entire subscription as a whole or to a specific subscriber only.
import { from, filter } from 'rxjs';
const obs = from([1, 2, 3]).pipe(filter(n => n > 2));
obs.subscribe(value => console.log(value));
obs.subscribe(value => console.log(value));
// Console output:
// 3
// 3
Here, the source itself filters the data, and none of the subscribers can get the unfiltered set—our “gossiper” is not willing to share everything they know.
import { from, filter } from 'rxjs';
const obs = from([1, 2, 3]);
obs.pipe(filter(n => n > 2)).subscribe(value => console.log(value));
obs.subscribe(value => console.log(value));
// Console output:
// 3
// 1
// 2
// 3
In this example, one of the listeners doesn’t want to know anything about numbers less than two, while obs
faithfully provides the entire set.
map
– Transform Data
When telling stories, it’s hard to resist embellishing them a little! And that’s where map
comes to the rescue, capable of transforming the data stream on the fly.
import { from, map } from 'rxjs';
const obs = from([1, 2, 3]).pipe(map(n => n * 2));
obs.subscribe(value => console.log(value));
// Console output:
// 2
// 4
// 6
-
map
takes each value (e.g., a number, text, or object) from the stream. - Transforms it as instructed by you.
- Passes the modified version of the data further.
Using interval
for Continuous Data
The previous steps help us get familiar with the mechanisms of working with RxJS, but they don't really resemble anything asynchronous— the code executes instantly, with no waiting or delays. That's why we should include one more function in our starter set: interval
. It creates an infinite stream of numbers starting from 0, emitting them at a given time interval in milliseconds.
Let's create an Observable that emits a new value every second:
import { interval } from 'rxjs';
const timerObservable = interval(1000); // Emit values every second.
timerObservable.subscribe(value => {
console.log(`One second passed: ${value}`);
});
// Console output:
// One second passed: 0
// One second passed: 1
// One second passed: 2
// ...
This generator will help you slightly expand the base for experiments alongside the functions mentioned above.
Conclusion
The main thing to remember is that mastering powerful tools like RxJS takes time. And that's completely normal. Honestly, no one becomes an expert in a single day.
Don't try to understand everything at once or apply dozens of operators immediately. It’s easy to get lost when attempting to "embrace the immense." Start with the simplest concepts, with topics you already grasp to some extent: experiment with from()
, interval()
, filter
, and map
until you feel comfortable writing code with these topics without effort or confusion. Once you’ve achieved that ease, the next steps will be considerably simpler.
Remember that every complex topic can be broken down into simpler parts. A deliberate, gradual approach is the key to making RxJS less of a confusing magic trick and more of a convenient, powerful tool that you’ll genuinely enjoy using.
Don’t be afraid to make mistakes, ask questions, or return to basic examples. Everyone progresses differently, but each of us started from the basics at some point.
In upcoming articles, we’ll explore how to make Observables even more powerful with other operators like switchMap
, mergeMap
, and others while trying to understand how to approach mastering this extensive list of operators properly.
Happy Coding!