Temporal API for Precise Date Management
Temporal API for Precise Date Management: An Exhaustive Guide Historical and Technical Context JavaScript has long been criticized for its handling of dates and times, primarily due to the Date object. The Date object was introduced in JavaScript 1.0 and was designed to encapsulate the concept of dates and times. However, due to its reliance on the underlying system's time zone settings and several peculiarities around parsing and formatting, it quickly became cumbersome for developers needing precise date management. The Limitations of the Original Date Object The charge against the Date object can be summarized as follows: Imprecise Representations: JavaScript’s Date object operates on milliseconds since the UNIX epoch (January 1, 1970), which can lead to inaccuracies, especially around daylight saving time changes or leap seconds. Complex API: Performing date and time calculations (such as adding durations or comparing dates) necessitated extensive boilerplate code. Time Zone Issues: The absence of native support for managing time zones has often forced developers to rely on third-party libraries like Moment.js or date-fns. Such inadequacies spurred the need for a more robust date and time handling mechanism, which led to the introduction of the Temporal API. As of its latest proposal, the Temporal API aims to seamlessly manage date and time across various contexts, ensuring precision and reliability. Leveraging the Temporal API Core Constructs of the Temporal API The Temporal API is built around several core constructs, each designed to handle specific needs in date and time management: Temporal.Instant: Represents a point in time in UTC. Temporal.PlainDate: Represents a date without a time zone and time. Temporal.PlainTime: Represents a time without a date. Temporal.PlainDateTime: Combines both date and time without a time zone. Temporal.ZonedDateTime: Represents a date and time in a specific time zone. Temporal.Duration: Represents an elapsed amount of time. Temporal.TimeZone: Handles time zone-related issues. Immutable Nature of Temporal Objects One of the significant advantages of the Temporal API is its immutable nature. This means that any modifications made to a Temporal object return a new object rather than altering the original. This behavior promotes functional programming practices, largely reducing side effects and ensuring consistent state management. In-Depth Code Examples To illustrate the power of the Temporal API, we will explore diverse scenarios that demonstrate its capabilities in handling complex date and time management tasks. Creating Dates with Temporal Let's start with creating different types of Temporal objects: // Instantiate Temporal objects const now = Temporal.now.instant(); // Current instant in UTC const today = Temporal.PlainDate.from('2023-10-01'); // A specific date const time = Temporal.PlainTime.from('14:30:00'); // A specific time console.log('Now:', now); console.log('Today:', today); console.log('Time:', time); Performing Date Calculations Arithmetic can pose challenges in date management, but the Temporal API simplifies these operations. // Adding durations to a date const nextWeek = today.add({ days: 7 }); const nextMonth = today.add({ months: 1 }); console.log('Next Week:', nextWeek.toString()); // 2023-10-08 console.log('Next Month:', nextMonth.toString()); // 2023-11-01 Time Zone Handling Consider the complexities of time zones. The Temporal API allows for easier management: // Creating a ZonedDateTime const zonedDateTime = Temporal.ZonedDateTime.from({ year: 2023, month: 10, day: 1, hour: 14, minute: 30, timeZone: 'America/New_York', }); console.log('Zoned DateTime:', zonedDateTime.toString()); // 2023-10-01T14:30:00-04:00[America/New_York] Edge Cases and Advanced Implementation Techniques Handling edge cases and discrepancies in date management can often lead to complicated scenarios. Consider the leap year and daylight saving transitions: // Checking leap years const isLeapYear = (year) => { return new Temporal.PlainDate(year, 2, 29).month === 2; }; console.log('Is 2024 a leap year?', isLeapYear(2024)); // true // Correctly managing daylight saving change const dstDate = Temporal.ZonedDateTime.from({ year: 2023, month: 11, day: 5, hour: 1, minute: 30, timeZone: 'America/New_York', }); console.log('DST Date:', dstDate.toString()); // Automatically adjusted for DST Real-World Use Cases in Industry The Temporal API is poised to redefine common applications in various industries: Financial Applications: Where precision in timestamps is critical for transactions and interest calculations. Event Management Systems: For scheduling events and managing recurring events that need to consider varying time zone adjustments. Data Analytics: For
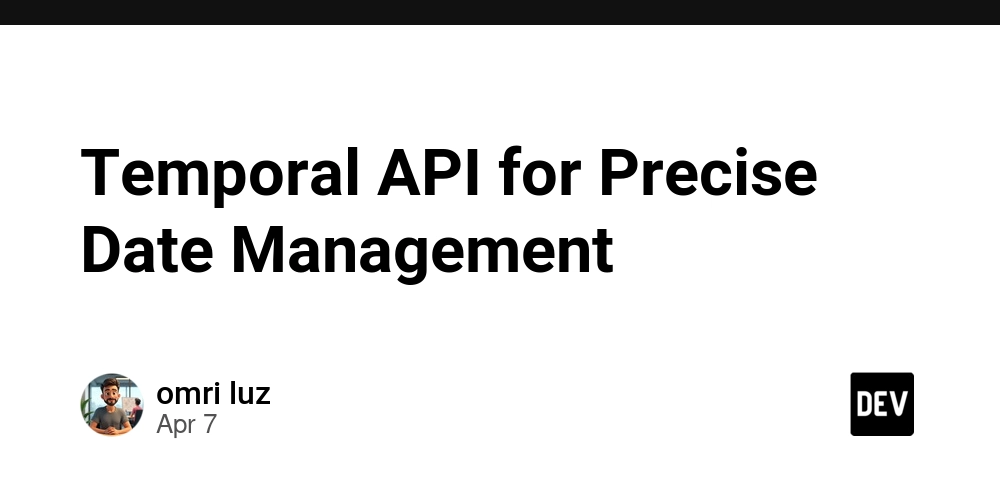
Temporal API for Precise Date Management: An Exhaustive Guide
Historical and Technical Context
JavaScript has long been criticized for its handling of dates and times, primarily due to the Date
object. The Date
object was introduced in JavaScript 1.0 and was designed to encapsulate the concept of dates and times. However, due to its reliance on the underlying system's time zone settings and several peculiarities around parsing and formatting, it quickly became cumbersome for developers needing precise date management.
The Limitations of the Original Date Object
The charge against the Date
object can be summarized as follows:
-
Imprecise Representations: JavaScript’s
Date
object operates on milliseconds since the UNIX epoch (January 1, 1970), which can lead to inaccuracies, especially around daylight saving time changes or leap seconds. - Complex API: Performing date and time calculations (such as adding durations or comparing dates) necessitated extensive boilerplate code.
- Time Zone Issues: The absence of native support for managing time zones has often forced developers to rely on third-party libraries like Moment.js or date-fns.
Such inadequacies spurred the need for a more robust date and time handling mechanism, which led to the introduction of the Temporal API. As of its latest proposal, the Temporal API aims to seamlessly manage date and time across various contexts, ensuring precision and reliability.
Leveraging the Temporal API
Core Constructs of the Temporal API
The Temporal API is built around several core constructs, each designed to handle specific needs in date and time management:
- Temporal.Instant: Represents a point in time in UTC.
- Temporal.PlainDate: Represents a date without a time zone and time.
- Temporal.PlainTime: Represents a time without a date.
- Temporal.PlainDateTime: Combines both date and time without a time zone.
- Temporal.ZonedDateTime: Represents a date and time in a specific time zone.
- Temporal.Duration: Represents an elapsed amount of time.
- Temporal.TimeZone: Handles time zone-related issues.
Immutable Nature of Temporal Objects
One of the significant advantages of the Temporal API is its immutable nature. This means that any modifications made to a Temporal object return a new object rather than altering the original. This behavior promotes functional programming practices, largely reducing side effects and ensuring consistent state management.
In-Depth Code Examples
To illustrate the power of the Temporal API, we will explore diverse scenarios that demonstrate its capabilities in handling complex date and time management tasks.
Creating Dates with Temporal
Let's start with creating different types of Temporal objects:
// Instantiate Temporal objects
const now = Temporal.now.instant(); // Current instant in UTC
const today = Temporal.PlainDate.from('2023-10-01'); // A specific date
const time = Temporal.PlainTime.from('14:30:00'); // A specific time
console.log('Now:', now);
console.log('Today:', today);
console.log('Time:', time);
Performing Date Calculations
Arithmetic can pose challenges in date management, but the Temporal API simplifies these operations.
// Adding durations to a date
const nextWeek = today.add({ days: 7 });
const nextMonth = today.add({ months: 1 });
console.log('Next Week:', nextWeek.toString()); // 2023-10-08
console.log('Next Month:', nextMonth.toString()); // 2023-11-01
Time Zone Handling
Consider the complexities of time zones. The Temporal API allows for easier management:
// Creating a ZonedDateTime
const zonedDateTime = Temporal.ZonedDateTime.from({
year: 2023,
month: 10,
day: 1,
hour: 14,
minute: 30,
timeZone: 'America/New_York',
});
console.log('Zoned DateTime:', zonedDateTime.toString()); // 2023-10-01T14:30:00-04:00[America/New_York]
Edge Cases and Advanced Implementation Techniques
Handling edge cases and discrepancies in date management can often lead to complicated scenarios. Consider the leap year and daylight saving transitions:
// Checking leap years
const isLeapYear = (year) => {
return new Temporal.PlainDate(year, 2, 29).month === 2;
};
console.log('Is 2024 a leap year?', isLeapYear(2024)); // true
// Correctly managing daylight saving change
const dstDate = Temporal.ZonedDateTime.from({
year: 2023,
month: 11,
day: 5,
hour: 1,
minute: 30,
timeZone: 'America/New_York',
});
console.log('DST Date:', dstDate.toString()); // Automatically adjusted for DST
Real-World Use Cases in Industry
The Temporal API is poised to redefine common applications in various industries:
- Financial Applications: Where precision in timestamps is critical for transactions and interest calculations.
- Event Management Systems: For scheduling events and managing recurring events that need to consider varying time zone adjustments.
- Data Analytics: For timestamp data management that requires seamless aggregation across time zones and varying granularities.
Alternatives: Comparing Temporal API with Third-Party Libraries
Traditionally, developers have relied on libraries like Moment.js or date-fns for date manipulation. Here’s a brief comparison:
Moment.js: While powerful, the library is mutable and heavy, leading to performance issues with large datasets. It’s also no longer actively maintained.
date-fns: While lightweight and functional, it relies on many utility functions, which can lead to code verbosity and require careful attention to the handling of time zones.
The Temporal API integrates the best components of these libraries with a native implementation that encourages immutability, concise syntax, and efficient performance.
Performance Considerations and Optimization Strategies
In performance-critical applications, understanding the security of Temporal operations is essential. The API is optimized for efficiency, but developers should keep an eye on:
- Avoiding unnecessary object creation; working with immutable objects can cause excessive memory usage if not managed correctly.
- Be cautious with conversions between Temporal and native Date objects, as these conversions can introduce performance overhead.
Debugging Techniques and Potential Pitfalls
Debugging date and time issues can be confounding. Here are advanced techniques and pitfalls to watch for:
Immutable Structures: Always be aware of which objects are mutable and which are not. Use tools like immutability-helper to track changes in state.
Date Parsing: Ensure date strings use ISO 8601 formatting, as the Temporal API tends to be less forgiving than other libraries in parsing ambiguous formats.
Time Zone Awareness: Automate tests that confirm proper behavior across different time zones. This could include simulating different locales in a test environment.
Edge Case Handling: Implement unit tests for leap seconds, DST changes, and abnormal month lengths to catch surprises early.
Conclusion
The Temporal API represents a seismic shift in how JavaScript handles date and time. By combining precision, immutability, and nuanced time-zone management, it emerges as the viable solution for complex date-related use cases. As developers dive into this API, understanding its intricacies, capabilities, and the inherent advantages it offers will distinguish seasoned developers in the evolving landscape of JavaScript programming.
References
By engaging deeply with the subject matter, you gain not only technical proficiency but also insight into the design philosophy behind the Temporal API, enabling you to harness its potential fully in your projects.