Angular 19: Mastering `input.required()` and Functional Inputs with Signals
Angular 19 brings a modernized way to declare inputs using the functional input() API, including input.required(), which replaces the classic @Input() decorator for standalone components and works beautifully with Signals. If you want to master modern Angular and write reactive, performant components, this is a key tool in your toolbox. What Is input() in Angular? The input() function is part of Angular's functional input binding system, introduced in Angular 16 and stabilized in Angular 19. It allows you to declare inputs without decorators. ✅ Example import { input } from '@angular/core'; export class MyComponent { userList = input.required(); } Benefits Over @Input() Feature Classic @Input() Functional input() Decorator-free ❌ ✅ Strong typing ⚠️ (with casting) ✅ Required enforcement ❌ ✅ Throws if missing Signal-compatible ❌ ✅ .signal built-in Works with Standalone ✅ ✅ (designed for it) input.required() vs input.optional() input.required() Requires the parent to always pass a value Throws a runtime error if missing products = input.required(); input.optional() Accepts undefined Can include a default value theme = input.optional('light'); Making Inputs Reactive with .signal You can instantly convert any input into a reactive Signal: userList = input.required().signal; Now you can use userList() in the template or inside computed signals and effects. Full Component Example import { Component, input } from '@angular/core'; interface Product { id: number; name: string; } @Component({ selector: 'app-product-list', standalone: true, template: ` { p.name } `, }) export class ProductListComponent { products = input.required().signal; } ✔️ This component is: Standalone Strongly typed Signal-based and reactive Runtime-safe with input enforcement Best Practices for Angular 19 Rule Why Use input.required() for critical props Enforces safety Prefer .signal when binding to templates Ensures reactivity Avoid mutable structures (e.g. .push()) Signals react to reference changes Combine with OnPush Optimizes rendering Final Thoughts Angular 19 continues the shift toward reactive, composable, and standalone-friendly components. input.required() and input.optional() are a huge leap forward in how we define and enforce inputs—especially when paired with Signals. Leave decorators behind. Embrace the functional future of Angular. Happy coding, and may all your inputs be safely typed and reactively bound! ⚡ angular #typescript #signals #frontend #architecture
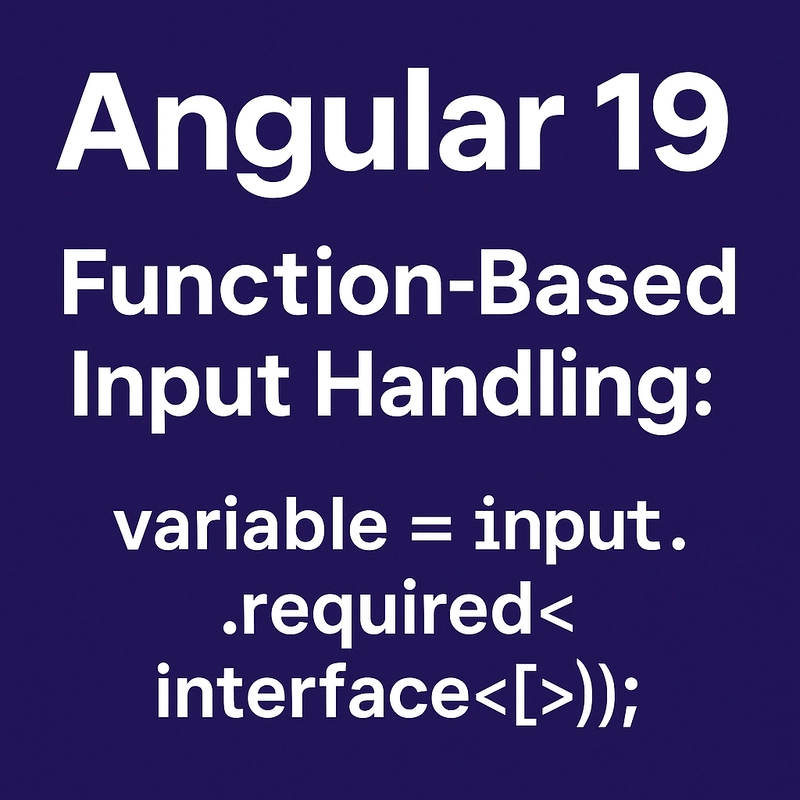
Angular 19 brings a modernized way to declare inputs using the functional input()
API, including input.required
, which replaces the classic @Input()
decorator for standalone components and works beautifully with Signals.
If you want to master modern Angular and write reactive, performant components, this is a key tool in your toolbox.
What Is input()
in Angular?
The input()
function is part of Angular's functional input binding system, introduced in Angular 16 and stabilized in Angular 19. It allows you to declare inputs without decorators.
✅ Example
import { input } from '@angular/core';
export class MyComponent {
userList = input.required<User[]>();
}
Benefits Over @Input()
Feature | Classic @Input()
|
Functional input()
|
---|---|---|
Decorator-free | ❌ | ✅ |
Strong typing | ⚠️ (with casting) | ✅ |
Required enforcement | ❌ | ✅ Throws if missing |
Signal-compatible | ❌ | ✅ .signal built-in |
Works with Standalone | ✅ | ✅ (designed for it) |
input.required()
vs input.optional()
input.required()
- Requires the parent to always pass a value
- Throws a runtime error if missing
products = input.required<Product[]>();
input.optional()
- Accepts
undefined
- Can include a default value
theme = input.optional<'light' | 'dark'>('light');
Making Inputs Reactive with .signal
You can instantly convert any input into a reactive Signal:
userList = input.required<User[]>().signal;
Now you can use userList()
in the template or inside computed signals and effects.
Full Component Example
import { Component, input } from '@angular/core';
interface Product {
id: number;
name: string;
}
@Component({
selector: 'app-product-list',
standalone: true,
template: `
- { p.name }
`,
})
export class ProductListComponent {
products = input.required<Product[]>().signal;
}
✔️ This component is:
- Standalone
- Strongly typed
- Signal-based and reactive
- Runtime-safe with input enforcement
Best Practices for Angular 19
Rule | Why |
---|---|
Use input.required() for critical props |
Enforces safety |
Prefer .signal when binding to templates |
Ensures reactivity |
Avoid mutable structures (e.g. .push() ) |
Signals react to reference changes |
Combine with OnPush
|
Optimizes rendering |
Final Thoughts
Angular 19 continues the shift toward reactive, composable, and standalone-friendly components. input.required()
and input.optional()
are a huge leap forward in how we define and enforce inputs—especially when paired with Signals.
Leave decorators behind. Embrace the functional future of Angular.
Happy coding, and may all your inputs be safely typed and reactively bound! ⚡