TargetJS: Rethinking UI with Declarative, Synchronous Pipelines
Introduction Explore TargetJS and contribute on GitHub. I have always been drawn to the JavaScript literal object. It is expressive, compact, and readable, if only there was a way to execute it. But JavaScript didn’t guarantee property order for a long time, until ES2015, which finally made the order predictable. It also introduced the shorter method syntax. This made the object literal more powerful and even more compact. This inspired the core idea behind TargetJS: Provide an internal wrapper (called "targets") for both properties and methods of the literal object. Execute the targets sequentially in the same order as the code is written, using the framework's execution cycle. Enable a functional pipeline between adjacent targets. Add lifecycles, looping, and timing to targets so they can execute or re-execute themselves when a condition or time is met. This also makes the functional pipeline even more powerful as we will see later. That's the basic idea. As a byproduct of uniformly treating properties and methods with lifecycles and states, state management becomes straightforward and implicit in the framework. To make the framework suitable for front-end development, TargetJS allows CSS styles to be integrated into the same object as targets especially since CSS styles closely resemble JavaScript object literals. To enhance style property utility, we added value iteration, so we can animate styles easily. In the following section, we explain how targets work and then demonstrate how they provide a unified solution for UI rendering, event handling, and API interactions. Understanding Targets With Basic examples At the heart of TargetJS lies the concept of "Targets." As mentioned in the introduction, Targets provide a unified wrapper for both properties and methods of JavaScript literal objects. This wrapping process is handled implicitly by the TargetJS engine. The framework's execution cycle run the active targets initially based on the order in which they're written. The execution cycle also ensures that the execution is synchronous. Ok, let's start with a simple example: import { App } from "targetj"; App({ background: "purple", width: 50, height: 50 }); This code renders a 50px purple square. All properties become targets but there is one static value for each so the target lifecycles end, and nothing happens afterward. The object by default will create a div element but can create any other element by implementing the special target baseElement. While a literal object can represent an HTML block, it is best used to represent a single HTML element. This creates an enhanced HTML element that is highly extensible and flexible for handling any logic. In the next example, we animate the width from 50px to 100px and back to 50px, using 50 steps with 10ms pauses. The _ prefix on the height target indicates it's initially inactive and not ready for execution. The $ postfix creates a functional pipeline, activating the height target when the preceding width target executes. This causes the height to dynamically scale with the width's value. prevTargetValue refers to the previous target's value, which, in this case, is the width. import { App } from "targetj"; App({ background: "purple", width: [{ list: [50, 100, 50] }, 50, 10], // Target values, steps, interval _height$() { // activated when width executes return this.prevTargetValue; } }); UI Rendering and Animation Example This example builds upon the previous square animation. We create 10 animated squares. We then transition their background from purple to orange. First, we generate 10 squares. We also want to create one square every 100ms: import { App } from "targetj"; App({ children: { cycles: 9, interval: 100, value: { background: "purple", width: [{ list: [50, 100, 50] }, 50, 10], _height$() { return this.prevTargetValue; } } } }); The children target is a special type of target that adds new items each time it executes. cycles: 9 specifies 10 total cycles (0-9), executed every 100ms, as defined by interval. To transition the background after the square animations, we add another target with the postfix $$, creating a functional pipeline. import { App } from "targetj"; App({ width: 1000, children: { cycles: 9, interval: 100, value: { background: "purple", width: [{ list: [50, 100, 50] }, 50, 10], _height$() { return this.prevTargetValue; } } }, _changeBackround$$() { this.getChildren().forEach((child) => child.setTarget("background", "orange", 100, 10) ); } }); The $$ postfix ensures that the changeBackround target executes only after the children target completes, including all of its children and their respective targets. This guarantees the background transition occurs after all squares are cre
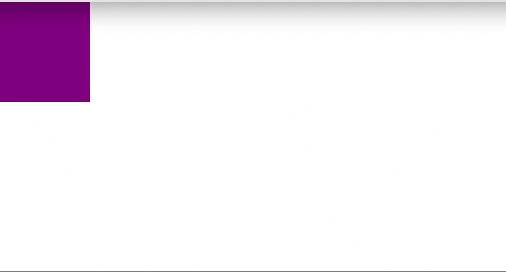
Introduction
Explore TargetJS and contribute on GitHub.
I have always been drawn to the JavaScript literal object. It is expressive, compact, and readable, if only there was a way to execute it. But JavaScript didn’t guarantee property order for a long time, until ES2015, which finally made the order predictable. It also introduced the shorter method syntax. This made the object literal more powerful and even more compact.
This inspired the core idea behind TargetJS:
Provide an internal wrapper (called "targets") for both properties and methods of the literal object.
Execute the targets sequentially in the same order as the code is written, using the framework's execution cycle.
Enable a functional pipeline between adjacent targets.
Add lifecycles, looping, and timing to targets so they can execute or re-execute themselves when a condition or time is met. This also makes the functional pipeline even more powerful as we will see later.
That's the basic idea.
As a byproduct of uniformly treating properties and methods with lifecycles and states, state management becomes straightforward and implicit in the framework.
To make the framework suitable for front-end development, TargetJS allows CSS styles to be integrated into the same object as targets especially since CSS styles closely resemble JavaScript object literals. To enhance style property utility, we added value iteration, so we can animate styles easily.
In the following section, we explain how targets work and then demonstrate how they provide a unified solution for UI rendering, event handling, and API interactions.
Understanding Targets With Basic examples
At the heart of TargetJS lies the concept of "Targets." As mentioned in the introduction, Targets provide a unified wrapper for both properties and methods of JavaScript literal objects. This wrapping process is handled implicitly by the TargetJS engine. The framework's execution cycle run the active targets initially based on the order in which they're written. The execution cycle also ensures that the execution is synchronous.
Ok, let's start with a simple example:
import { App } from "targetj";
App({
background: "purple",
width: 50,
height: 50
});
This code renders a 50px purple square. All properties become targets but there is one static value for each so the target lifecycles end, and nothing happens afterward.
The object by default will create a div
element but can create any other element by implementing the special target baseElement
. While a literal object can represent an HTML block, it is best used to represent a single HTML element. This creates an enhanced HTML element that is highly extensible and flexible for handling any logic.
In the next example, we animate the width from 50px to 100px and back to 50px, using 50 steps with 10ms pauses. The _
prefix on the height target indicates it's initially inactive and not ready for execution. The $
postfix creates a functional pipeline, activating the height target when the preceding width target executes. This causes the height to dynamically scale with the width's value. prevTargetValue refers to the previous target's value, which, in this case, is the width.
import { App } from "targetj";
App({
background: "purple",
width: [{ list: [50, 100, 50] }, 50, 10], // Target values, steps, interval
_height$() { // activated when width executes
return this.prevTargetValue;
}
});
UI Rendering and Animation Example
This example builds upon the previous square animation. We create 10 animated squares. We then transition their background from purple to orange.
First, we generate 10 squares. We also want to create one square every 100ms:
import { App } from "targetj";
App({
children: {
cycles: 9,
interval: 100,
value: {
background: "purple",
width: [{ list: [50, 100, 50] }, 50, 10],
_height$() {
return this.prevTargetValue;
}
}
}
});
The children
target is a special type of target that adds new items each time it executes. cycles: 9
specifies 10 total cycles (0-9), executed every 100ms, as defined by interval
.
To transition the background after the square animations, we add another target with the postfix $$
, creating a functional pipeline.
import { App } from "targetj";
App({
width: 1000,
children: {
cycles: 9,
interval: 100,
value: {
background: "purple",
width: [{ list: [50, 100, 50] }, 50, 10],
_height$() {
return this.prevTargetValue;
}
}
},
_changeBackround$$() {
this.getChildren().forEach((child) =>
child.setTarget("background", "orange", 100, 10)
);
}
});
The $$
postfix ensures that the changeBackround
target executes only after the children
target completes, including all of its children and their respective targets. This guarantees the background transition occurs after all squares are created and their animations finish.
Event Handling Example
This example adds click event handling to the squares, resetting their width animation on each click. onClick
is another specialized target that activates in response to click events.
import { App } from "targetj";
App({
width: 1000,
children: {
cycles: 9,
interval: 100,
value: {
background: "purple",
width: [{ list: [50, 100, 50] }, 50, 10],
_height$() {
return this.prevTargetValue;
},
onClick() {
this.activateTarget("width");
}
}
},
_changeBackround$$() {
this.getChildren().forEach(child =>
child.setTarget("background", "orange", 100, 10)
);
}
});
API Call Example
This example demonstrates integrating API calls into the square animation. We have added two new targets: loadSquare
to fetch data, and populate
to display the result. As the populate
target ends with $
, it executes after the API completes. prevTargetValue
refers to the API's response.
Note that the changeBackround
target will not execute until the API result is available and all other targets have completed.
import { App, fetch } from "targetj";
App({
width: 1000,
children: {
cycles: 9,
interval: 100,
value: {
id: "square",
background: "purple",
width: [{list: [50, 100, 50]}, 50, 10],
_height$() {
return this.prevTargetValue;
},
loadSquare() {
fetch(this, "https://targetjs.io/api/randomUser", {id: this.oid});
},
_populate$() {
this.setTarget("html", this.prevTargetValue.name);
}
}
},
_changeBackround$$() {
this.getChildren().forEach(child => child.setTarget("background", "orange", 100, 10));
}
});
Try TargetJS Today!
TargetJS is an evolving framework. We invite you to explore its potential and contribute to its development.