Smart Function to Connect a Wallet and Sign Transactions on EVM or SOL Via Phantom
Phantom Wallet is one of the most popular wallets for interacting with the Solana blockchain. If you're building a dApp that requires users to connect their wallets and sign transactions, here's a smart way to achieve that using JavaScript or typescript. Connecting Phantom Wallet First, ensure the user has Phantom installed. Then, use the following function to connect async function connectPhantom() { if (window.solana && window.solana.isPhantom) { try { const response = await window.solana.connect(); console.log("Connected with public key:", response.publicKey.toString()); return response.publicKey; } catch (err) { console.error("Connection failed", err); } } else { console.error("Phantom wallet not found! Install it from https://phantom.app/"); } } Signing a Transaction Once connected, you can create and sign a transaction using Phantom async function signAndSendTransaction(transaction) { if (!window.solana || !window.solana.isPhantom) { console.error("Phantom wallet is not connected"); return; } try { transaction.feePayer = window.solana.publicKey; transaction.recentBlockhash = (await connection.getRecentBlockhash()).blockhash; const signedTransaction = await window.solana.signTransaction(transaction); const signature = await connection.sendRawTransaction(signedTransaction.serialize()); console.log("Transaction signature:", signature); return signature; } catch (error) { console.error("Transaction signing failed", error); } } Using the Functions with a Button To integrate these functions into your UI, you can use a simple button like this Connect Wallet Sign Transaction document.getElementById("connect-button").addEventListener("click", async () => { await connectPhantom(); }); document.getElementById("sign-button").addEventListener("click", async () => { const transaction = new solanaWeb3.Transaction(); // Add instructions as needed await signAndSendTransaction(transaction); }); This function allows your dApp to connect with Phantom and sign transactions like swap, lend, stake or others , making it easy for users to interact with the Solana blockchain. Make sure to handle connection errors and display appropriate messages to improve user experience. Thanks
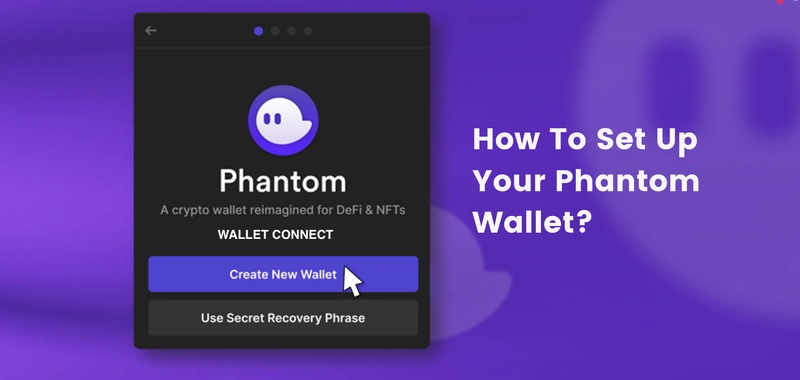
Phantom Wallet is one of the most popular wallets for interacting with the Solana blockchain. If you're building a dApp that requires users to connect their wallets and sign transactions, here's a smart way to achieve that using JavaScript or typescript.
Connecting Phantom Wallet
First, ensure the user has Phantom installed. Then, use the following function to connect
async function connectPhantom() {
if (window.solana && window.solana.isPhantom) {
try {
const response = await window.solana.connect();
console.log("Connected with public key:", response.publicKey.toString());
return response.publicKey;
} catch (err) {
console.error("Connection failed", err);
}
} else {
console.error("Phantom wallet not found! Install it from https://phantom.app/");
}
}
Signing a Transaction
Once connected, you can create and sign a transaction using Phantom
async function signAndSendTransaction(transaction) {
if (!window.solana || !window.solana.isPhantom) {
console.error("Phantom wallet is not connected");
return;
}
try {
transaction.feePayer = window.solana.publicKey;
transaction.recentBlockhash = (await connection.getRecentBlockhash()).blockhash;
const signedTransaction = await window.solana.signTransaction(transaction);
const signature = await connection.sendRawTransaction(signedTransaction.serialize());
console.log("Transaction signature:", signature);
return signature;
} catch (error) {
console.error("Transaction signing failed", error);
}
}
Using the Functions with a Button
To integrate these functions into your UI, you can use a simple button like this
This function allows your dApp to connect with Phantom and sign transactions like swap, lend, stake or others , making it easy for users to interact with the Solana blockchain. Make sure to handle connection errors and display appropriate messages to improve user experience.
Thanks